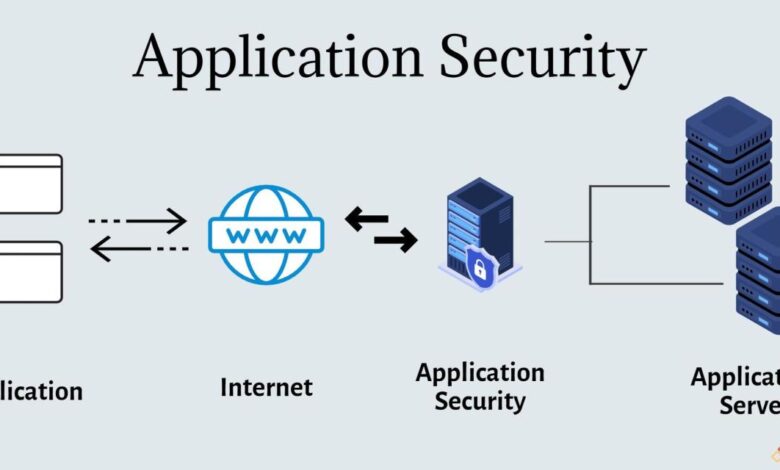
7 Steps to Secure Design Patterns A Robust Software Security Foundation
7 Steps to implement secure design patterns a robust foundation for software security – that’s what we’re diving into today! Building secure software isn’t just about adding a few security features after the fact; it’s about weaving security into the very fabric of your application from the ground up. Ignoring secure design patterns can lead to vulnerabilities that expose your users’ data and your reputation to significant risk.
We’ll explore seven crucial steps to build a system that’s resilient against common attacks, from input validation to continuous monitoring. Get ready to level up your security game!
This post will walk you through practical, actionable steps to improve your software security. We’ll cover essential concepts like input validation, authentication, secure data handling, and more, providing real-world examples and best practices along the way. By the end, you’ll have a solid understanding of how to build a secure foundation for your next project – or significantly bolster the security of your existing one.
Let’s get started!
Introduction
Secure design patterns are pre-defined, reusable solutions to common security vulnerabilities in software development. They provide a blueprint for building secure applications by incorporating security considerations from the very beginning of the design process, rather than as an afterthought. This proactive approach is crucial for mitigating risks and preventing costly security breaches.A robust foundation for software security is paramount because it directly impacts the confidentiality, integrity, and availability of data and systems.
Building security into the design, rather than patching it on later, leads to more efficient, reliable, and cost-effective security measures. A well-structured, secure design makes it significantly harder for attackers to exploit vulnerabilities, even if some are discovered later. Proactive security measures also reduce the likelihood of significant reputational damage and financial losses associated with data breaches.
Examples of Security Breaches Caused by Poor Design
Poorly designed software is a major contributor to many significant security breaches. For example, the Heartbleed vulnerability (2014) exploited a flaw in the OpenSSL cryptographic library, allowing attackers to steal sensitive data, including usernames, passwords, and private keys. This vulnerability stemmed from a fundamental design flaw that failed to properly validate the length of data being read from memory.
Another example is the Equifax data breach (2017), where a failure to patch a known vulnerability in the Apache Struts framework allowed attackers to access personal information of millions of individuals. This breach highlights the importance of using up-to-date software and adhering to secure coding practices. The Yahoo data breaches (2013 and 2014), which compromised billions of user accounts, were also partly attributed to weaknesses in their system architecture and inadequate security controls, demonstrating the devastating consequences of poor design choices at scale.
These examples highlight that failing to prioritize secure design leads to widespread and costly consequences.
Step 1: Input Validation and Sanitization
Building a secure application starts with controlling what goes in. Neglecting input validation and sanitization is like leaving your front door unlocked – inviting trouble. This step focuses on rigorously checking and cleaning user-supplied data to prevent injection attacks and other vulnerabilities. We’ll explore various techniques and their strengths and weaknesses.Input validation and sanitization are crucial for preventing various attacks, most notably SQL injection, cross-site scripting (XSS), and command injection.
These attacks exploit vulnerabilities in how applications handle user input, allowing attackers to execute malicious code on the server or manipulate the application’s behavior. By implementing robust input validation and sanitization, we can significantly reduce the risk of these attacks.
Input Validation Techniques
A multi-layered approach to input validation is best. This involves checking data type, length, format, and content, often employing a combination of techniques. The following table compares several common methods:
Technique | Description | Pros | Cons |
---|---|---|---|
Whitelist Validation | Only allows explicitly defined characters or patterns. | Highly secure; prevents unexpected input. | Requires careful definition of allowed input; can be complex for varied input. |
Blacklist Validation | Blocks known malicious characters or patterns. | Easier to implement initially. | Vulnerable to bypass if new malicious patterns emerge; less secure than whitelisting. |
Regular Expressions | Uses patterns to define acceptable input formats. | Flexible and powerful for complex validation rules. | Can be difficult to write and debug; performance can be an issue with complex patterns. |
Data Type Validation | Checks if the input conforms to the expected data type (e.g., integer, string, date). | Simple and effective for basic type checking. | May not catch all invalid input, especially for complex data structures. |
Escaping User-Supplied Data
Even with thorough input validation, it’s vital to escape user-supplied data before displaying it on a web page. Escaping, or encoding, transforms special characters into their corresponding HTML entities. This prevents the browser from interpreting them as code, thus mitigating XSS attacks. For example, the less-than symbol ( <) should be replaced with < before being displayed. Failure to escape data can lead to attackers injecting malicious JavaScript code, stealing cookies, or redirecting users to phishing sites. Different contexts (HTML attributes, JavaScript, CSS, etc.) require different escaping mechanisms. Using a well-tested and context-aware escaping library is highly recommended.
Step 2: Authentication and Authorization
Securing your application hinges on effectively verifying user identities (authentication) and controlling what they can access (authorization).
This step is crucial for preventing unauthorized access and maintaining data integrity. A robust authentication and authorization system acts as a gatekeeper, ensuring only legitimate users can interact with sensitive resources within your application.Authentication mechanisms determine who a user is, while authorization defines what actions they are permitted to perform. A well-designed system considers both aspects to create a secure environment.
Authentication Mechanisms
Several methods exist for verifying user identities. The choice depends on the security requirements and the context of your application. Stronger methods typically involve multiple factors to enhance security.
- Password-Based Authentication: This traditional method relies on users providing a username and password. While simple to implement, it’s vulnerable to password cracking and phishing attacks. Strong password policies, including length, complexity requirements, and regular password changes, are essential for mitigating these risks. Salting and hashing passwords are also crucial to prevent easy retrieval even if a database is compromised.
- Multi-Factor Authentication (MFA): MFA adds an extra layer of security by requiring users to provide multiple forms of authentication. Common factors include something they know (password), something they have (security token or smartphone), and something they are (biometrics like fingerprint or facial recognition). MFA significantly reduces the risk of unauthorized access, even if one factor is compromised.
- Token-Based Authentication: This approach uses short-lived tokens (like JWTs – JSON Web Tokens) instead of passwords. These tokens are generated upon successful authentication and are used for subsequent requests. They offer enhanced security compared to passwords as they don’t require storing sensitive credentials directly in the database. The use of short-lived tokens reduces the impact of compromised tokens.
- Biometric Authentication: This method uses unique biological characteristics, such as fingerprints or facial recognition, to authenticate users. It offers a high level of security and convenience but can raise privacy concerns. It’s crucial to implement robust security measures to protect biometric data.
Access Control Models: RBAC vs. ABAC
Access control models determine which users have access to specific resources and actions. Role-Based Access Control (RBAC) and Attribute-Based Access Control (ABAC) are two prominent models.
- Role-Based Access Control (RBAC): RBAC assigns users to roles, and roles are associated with permissions. For example, an “administrator” role might have full access, while a “user” role has limited access. This simplifies access management, especially in large organizations with many users. However, managing roles and permissions can become complex as the system grows.
- Attribute-Based Access Control (ABAC): ABAC is a more granular and flexible model. It allows access control decisions based on attributes of the user, the resource, and the environment. For example, access could be granted based on the user’s department, the resource’s sensitivity level, and the time of day. This provides finer-grained control than RBAC but requires more complex implementation.
RBAC is generally easier to implement and manage than ABAC, making it suitable for simpler applications. ABAC offers more flexibility and granularity, making it better suited for complex systems requiring dynamic access control policies.
Authentication and Authorization Process Flowchart
Imagine a simplified flowchart for a typical web application.The flowchart would begin with a user attempting to access a protected resource. This would trigger an authentication request. If the user provides valid credentials (e.g., username and password), the authentication system verifies them against a database or other authentication source. Upon successful authentication, a session token or similar is issued.
Next, the authorization system evaluates the user’s roles and permissions against the requested resource. If the user has the necessary permissions, access is granted. Otherwise, access is denied, and an appropriate message is returned to the user. This entire process would be encapsulated within a secure communication channel, such as HTTPS. The session token would be used for subsequent requests within the same session, ensuring continued access without repeated authentication.
Regular token expiration and refresh mechanisms should also be implemented to improve security.
Step 3: Secure Data Handling and Storage
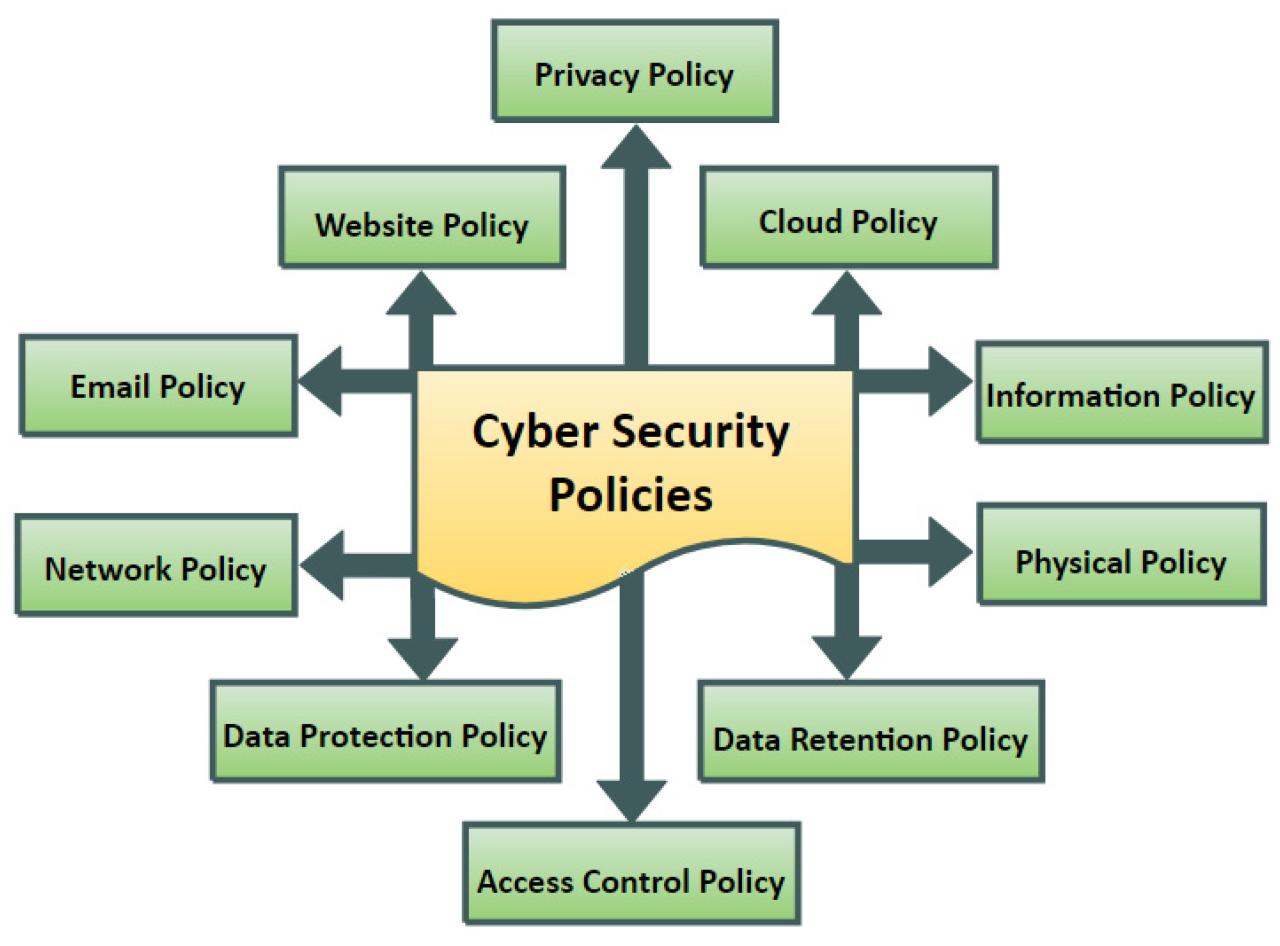
Protecting sensitive data is paramount in building secure software. This step focuses on implementing robust strategies for data encryption, loss prevention, and database security to ensure confidentiality, integrity, and availability. Neglecting these measures can lead to significant breaches and reputational damage.Data encryption is crucial for safeguarding sensitive information both while it’s being transmitted (in transit) and when it’s stored (at rest).
Effective encryption renders data unintelligible to unauthorized individuals, even if they gain access to the storage or network. Implementing robust data loss prevention (DLP) measures complements encryption by minimizing the risk of accidental or malicious data exposure.
Data Encryption: In Transit and At Rest
Encrypting data in transit protects it from interception during transmission across networks. This is commonly achieved using Transport Layer Security (TLS) or Secure Sockets Layer (SSL) protocols, which establish encrypted connections between communicating systems. For data at rest, encryption algorithms like Advanced Encryption Standard (AES) with strong key lengths (e.g., AES-256) are essential. The encryption keys themselves must be securely managed and protected, often using dedicated key management systems.
Consider using different encryption keys for different data types or sensitivity levels. For example, customer credit card data might require a separate, more robust encryption key than less sensitive contact information.
Data Loss Prevention (DLP) Measures
Data loss prevention involves implementing strategies and technologies to prevent sensitive data from leaving the organization’s control. This includes measures such as access control lists (ACLs) that restrict who can access specific data, data masking techniques that obscure sensitive information while allowing it to be used for testing or analysis, and intrusion detection systems (IDS) that monitor network traffic for suspicious activity.
DLP also encompasses regular data backups and disaster recovery plans, ensuring business continuity in the event of data loss. A comprehensive DLP strategy also includes employee training on data security best practices and the importance of following security protocols. Regular security audits and penetration testing can help identify vulnerabilities and improve the effectiveness of DLP measures.
Database Security Best Practices
Database security is critical as databases are central repositories of sensitive information. Implementing strong access control mechanisms, such as role-based access control (RBAC), is fundamental. RBAC assigns permissions based on user roles, limiting access to only the data necessary for a given role. This granular control minimizes the risk of unauthorized data access. Additionally, rigorous query sanitization is crucial to prevent SQL injection attacks.
Parameterization or prepared statements should always be used to prevent malicious code from being injected into database queries. Regular database security audits and vulnerability assessments are essential to identify and address potential weaknesses. Finally, data encryption at rest within the database itself, using features like Transparent Data Encryption (TDE) in SQL Server, is a vital component of a comprehensive database security strategy.
Step 4: Session Management and Security: 7 Steps To Implement Secure Design Patterns A Robust Foundation For Software Security
Session management is a critical aspect of web application security. It governs how users are identified and authenticated throughout their interaction with a system. Insecure session management practices leave applications vulnerable to a range of attacks, potentially compromising user data and system integrity. Understanding and implementing robust session management techniques is therefore paramount.Insecure session management practices expose applications to several significant risks.
For example, session hijacking occurs when an attacker gains unauthorized access to a user’s session ID, allowing them to impersonate the user and access their data or perform actions on their behalf. Session fixation, where an attacker forces a victim to use a specific session ID, is another serious vulnerability. Furthermore, improper session timeout configurations can lead to prolonged sessions, increasing the window of opportunity for attackers.
Finally, predictable session IDs can be easily guessed or brute-forced, bypassing authentication mechanisms.
Building secure applications starts with a solid foundation, and that’s exactly what my 7 steps to implement secure design patterns provide. These steps are crucial, regardless of your development approach, even when considering the exciting advancements in domino app dev the low code and pro code future , which offers both speed and flexibility. Ultimately, applying these 7 steps ensures your Domino apps—no matter how they’re built—remain secure and resilient.
Secure Session Management Techniques
Employing secure session management involves several key strategies. HTTPS, by encrypting the communication between the client and the server, protects session IDs from being intercepted during transmission. Secure cookies, configured with appropriate attributes like `HttpOnly` and `Secure`, prevent client-side scripting attacks and ensure that cookies are only transmitted over HTTPS. Regular session timeouts automatically terminate sessions after a period of inactivity, mitigating the risk of prolonged sessions.
Additionally, using unpredictable session IDs, generated using cryptographically secure random number generators, makes it significantly harder for attackers to guess or brute-force session IDs. Regularly rotating session IDs further enhances security.
Comparison of Session Management Techniques
The following table compares different session management techniques, highlighting their strengths and weaknesses.
Technique | Strengths | Weaknesses | Suitable Use Cases |
---|---|---|---|
Cookie-based (with HTTPS and Secure/HttpOnly flags) | Simple to implement, widely supported, efficient. | Vulnerable to XSS if not properly configured (lack of HttpOnly flag). Relies on browser’s cookie handling. | Most web applications; requires careful configuration. |
Token-based (JWT) | Stateless, more secure against session hijacking, can be used with HTTPS or other secure channels. | Requires more complex implementation, potentially larger payload sizes. | APIs, Single Page Applications (SPAs), microservices. |
Server-side session management (without cookies) | High security, avoids client-side storage of sensitive session data. | More complex implementation, may require additional infrastructure (e.g., distributed caching). | High-security applications where client-side storage is undesirable. |
Step 5: Error Handling and Logging
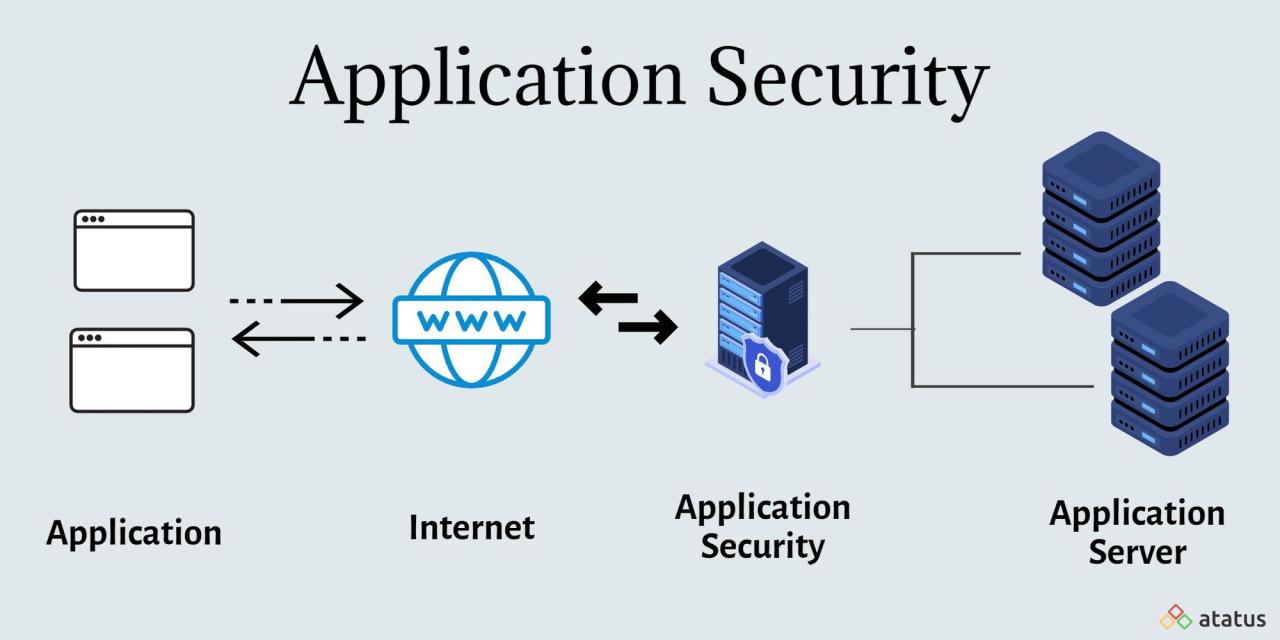
Secure error handling and robust logging are critical components of a secure application. Failing to implement these properly can expose sensitive information to attackers, making your application vulnerable to exploitation. Effective error handling and logging provide both a defensive mechanism against attacks and valuable insight into the system’s behavior, aiding in identifying and resolving security vulnerabilities.Proper error handling prevents the leakage of sensitive information by carefully controlling what information is displayed to the user and what is logged internally.
Robust logging mechanisms provide a detailed audit trail of security-relevant events, enabling security professionals to quickly identify and respond to potential threats. By combining these practices, developers significantly enhance the security posture of their applications.
Secure Error Message Handling
The information revealed in error messages can inadvertently expose sensitive details about your system’s architecture and data. Instead of displaying detailed error messages to the end-user, generic error messages should be presented. These messages should provide enough information for the user to understand the problem without revealing any sensitive information. For example, instead of “Database query failed: Incorrect username and password for user ‘admin’ at 10:30 AM”, a more secure message would be “We are experiencing a temporary problem.
Please try again later.” Internal logs, however, should record the full details of the error for debugging and security analysis.
Implementation of Robust Logging Mechanisms
A robust logging system is crucial for tracking security-relevant events. This system should log events such as login attempts (successful and failed), data access requests, security policy changes, and error occurrences. The logs should include timestamps, user IDs (or equivalent identifiers), IP addresses, and detailed descriptions of the events. It’s important to use a secure logging mechanism that protects the logs from unauthorized access and modification.
Consider using centralized logging systems with appropriate access controls and encryption to ensure the integrity and confidentiality of your logs. Furthermore, regular review of logs is essential for proactive threat detection and incident response.
Examples of Secure Error Messages
Consider the following scenarios and how to handle them securely:
Scenario | Insecure Error Message | Secure Error Message |
---|---|---|
File not found | “Error 404: File ‘/etc/passwd’ not found.” | “The requested resource could not be found.” |
Database connection error | “Database connection failed: Connection refused to ‘localhost:5432’.” | “We are currently experiencing technical difficulties. Please try again later.” |
Invalid input | “Error: Invalid SQL injection attempt detected.” | “The information you provided is invalid. Please check your input and try again.” |
Remember, the goal is to provide enough information to the user to understand the problem without revealing sensitive details about the system’s internal workings or data. Internal logs should capture the complete details of the error for debugging and security analysis.
Step 6: Regular Security Audits and Penetration Testing
Building a secure application isn’t a one-time event; it’s an ongoing process. Even with the best design patterns implemented, vulnerabilities can still emerge over time due to evolving threats, code changes, and unforeseen circumstances. This is where regular security audits and penetration testing become crucial. They act as a safety net, identifying weaknesses before malicious actors can exploit them.Regular security assessments and penetration testing are vital for maintaining a robust security posture.
These proactive measures help identify vulnerabilities, assess the effectiveness of existing security controls, and ultimately reduce the risk of security breaches. Failing to conduct these assessments leaves your application vulnerable to attacks, potentially leading to data breaches, financial losses, and reputational damage. Think of it like a regular health checkup for your software – it’s preventative maintenance that pays off in the long run.
Penetration Testing Methodologies, 7 steps to implement secure design patterns a robust foundation for software security
Penetration testing employs various methodologies, each with its own approach and focus. The choice of methodology depends on factors such as the application’s criticality, budget, and available time. Understanding these methodologies helps you choose the most appropriate approach for your specific needs.
- Black Box Testing: Testers have no prior knowledge of the system’s internal workings. This simulates a real-world attack scenario, where attackers have limited information.
- White Box Testing: Testers have complete knowledge of the system’s architecture, code, and configuration. This allows for a more in-depth analysis of vulnerabilities.
- Gray Box Testing: This approach combines elements of both black box and white box testing. Testers have partial knowledge of the system, such as network diagrams or high-level architecture details.
Tools and Techniques for Security Audits and Penetration Testing
A range of tools and techniques are employed during security audits and penetration testing. The specific tools used often depend on the chosen methodology and the target system. However, some common tools and techniques include:
- Static Application Security Testing (SAST): Analyzes source code without executing it to identify potential vulnerabilities. Examples include SonarQube and Fortify.
- Dynamic Application Security Testing (DAST): Tests the running application to identify vulnerabilities during runtime. Examples include Burp Suite and OWASP ZAP.
- Network Scanning: Uses tools like Nmap to identify open ports and services on a network, revealing potential entry points for attackers.
- Vulnerability Scanners: Automated tools that scan for known vulnerabilities based on publicly available databases like the National Vulnerability Database (NVD). Examples include Nessus and QualysGuard.
- Manual Penetration Testing: Involves skilled security professionals using a combination of techniques to exploit vulnerabilities. This often includes social engineering, exploiting known vulnerabilities, and attempting to bypass security controls.
Step 7: Continuous Monitoring and Improvement
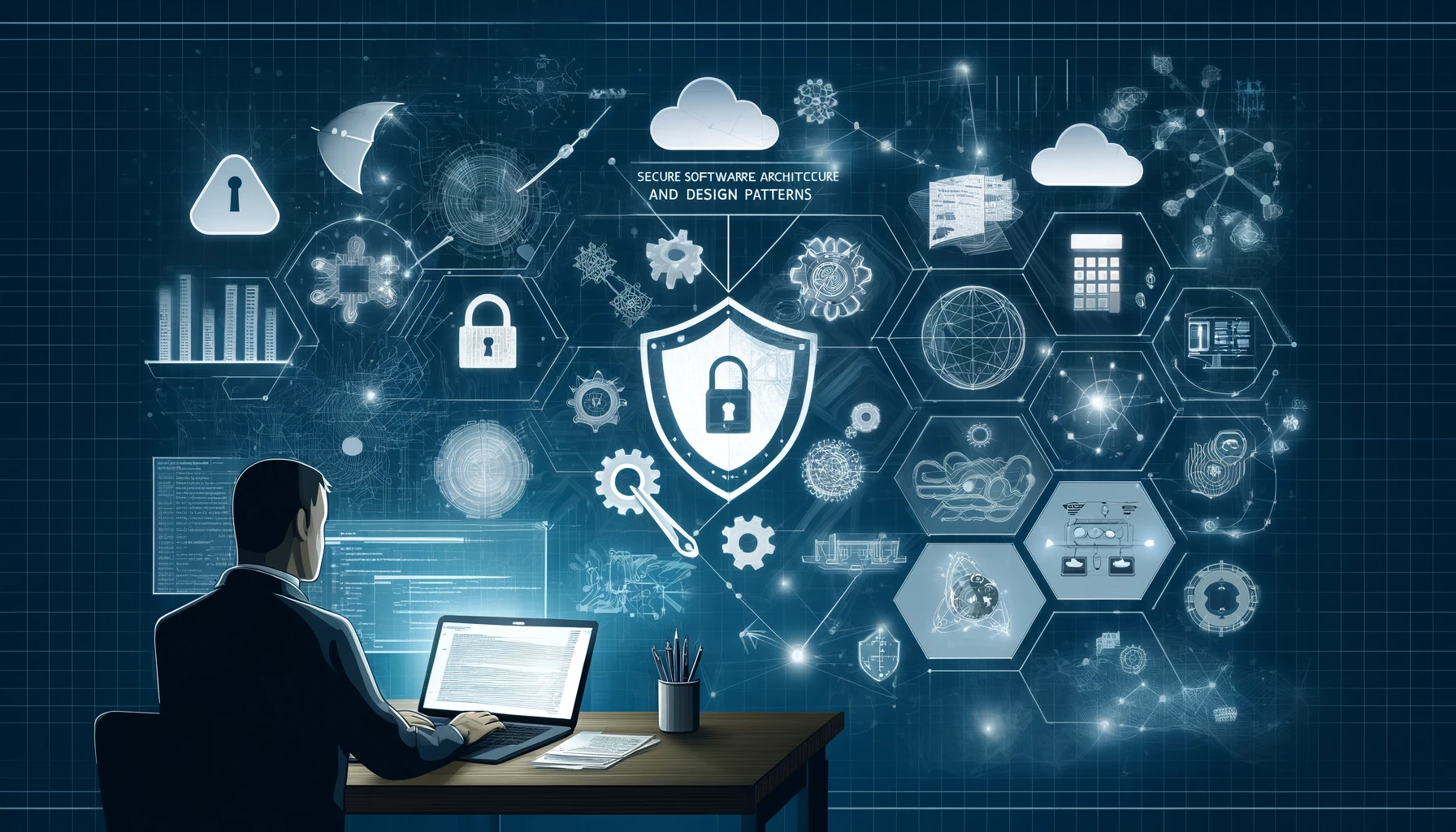
Building secure software isn’t a one-time event; it’s an ongoing process. Continuous monitoring and improvement are crucial for maintaining the long-term security of your application. This final step ensures that vulnerabilities are identified and addressed promptly, preventing breaches and maintaining user trust.Implementing continuous security monitoring involves a multi-faceted approach that combines automated tools with human oversight. This proactive strategy allows for early detection of threats and minimizes the impact of potential security incidents.
A robust response plan is also essential to effectively manage and resolve any security issues that may arise. Finally, integrating security practices into every phase of the software development lifecycle (SDLC) is paramount for building secure software from the ground up.
Strategies for Implementing Continuous Security Monitoring
Effective continuous security monitoring relies on a combination of automated tools and human expertise. Automated tools provide real-time alerts and analysis of system logs, network traffic, and application behavior. These tools can identify suspicious activities, potential vulnerabilities, and anomalies that might indicate a security breach. Human analysts are crucial for interpreting the data provided by these tools, prioritizing alerts, and investigating potential threats.
They bring context and judgment to the process, which automated systems alone often lack. For example, a sudden spike in login attempts from an unusual geographic location might be flagged by an automated system, but a human analyst would need to determine whether it’s a legitimate user or a potential attack.
Responding to Security Incidents Effectively
A well-defined incident response plan is critical for minimizing the damage caused by security incidents. This plan should Artikel clear procedures for identifying, containing, eradicating, recovering from, and learning from security incidents. Key elements include: establishing a dedicated incident response team, defining communication protocols, creating a process for evidence collection and preservation, and outlining steps for system restoration and user notification.
For instance, a company might have a documented process that dictates the steps to be taken if a data breach is detected, including notifying affected users, law enforcement, and relevant regulatory bodies within a specific timeframe. Regular drills and simulations are also crucial to ensure that the incident response team is prepared to handle real-world scenarios effectively.
Integrating Security into the Software Development Lifecycle (SDLC)
Integrating security into the SDLC is a shift-left approach that emphasizes building security into the software from the initial design phase. This contrasts with traditional approaches where security is often an afterthought. Implementing a Secure SDLC involves incorporating security practices into each stage, from requirements gathering and design to development, testing, deployment, and maintenance. This includes activities such as security requirements analysis, threat modeling, secure coding practices, automated security testing, and regular security reviews.
By integrating security throughout the SDLC, organizations can significantly reduce the risk of vulnerabilities and improve the overall security posture of their software. For example, conducting regular code reviews to identify and address potential vulnerabilities before they reach production can significantly reduce the risk of exploitation. The use of static and dynamic application security testing (SAST and DAST) tools throughout the development process further enhances this proactive approach.
Wrap-Up
So there you have it – seven key steps to building robust software security by implementing secure design patterns. Remember, security is an ongoing process, not a one-time fix. Regular audits, penetration testing, and continuous monitoring are crucial for staying ahead of evolving threats. By integrating these practices into your development lifecycle, you’ll create applications that are not only functional and efficient but also secure and trustworthy.
Prioritizing security from the beginning is an investment that pays off in the long run, protecting your users, your data, and your reputation. Let’s build a safer digital world, one secure application at a time!
Questions Often Asked
What are the biggest risks of ignoring secure design patterns?
Ignoring secure design patterns significantly increases your risk of data breaches, financial losses, reputational damage, legal repercussions, and loss of customer trust. Vulnerabilities can be easily exploited leading to significant consequences.
How often should I perform security audits and penetration testing?
The frequency depends on your application’s criticality and sensitivity of the data it handles. However, at a minimum, annual penetration testing and regular security audits are recommended. More frequent assessments may be necessary for high-risk applications.
What are some common examples of insecure design patterns?
Examples include insufficient input validation (leading to injection attacks), weak authentication mechanisms, improper session management, and inadequate error handling (revealing sensitive information).
Can I implement these steps without specialized security expertise?
While having dedicated security expertise is beneficial, many of these steps can be implemented by developers with a solid understanding of security best practices. Resources like OWASP and SANS offer valuable information and guidance.