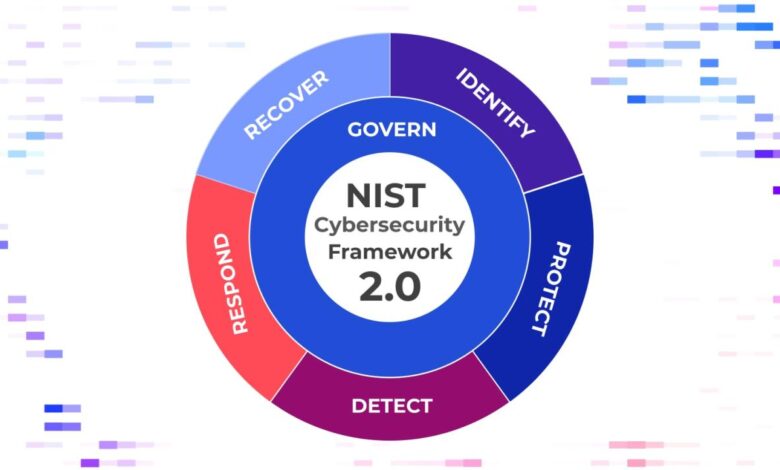
How NIST CSF 2.0 Can Help Schools
How nist csf 2 0 can help schools – How NIST CSF 2.0 can help schools is a question increasingly crucial in our hyper-connected world. Think about it: schools are treasure troves of sensitive data – student records, financial information, research projects – all vulnerable to cyberattacks. But navigating the complex world of cybersecurity doesn’t have to be a daunting task. The NIST Cybersecurity Framework (CSF) version 2.0 offers a practical, adaptable roadmap for schools of all sizes to strengthen their defenses and protect what matters most.
This framework, developed by the National Institute of Standards and Technology, provides a flexible approach, not a rigid set of rules. It Artikels five core functions – Identify, Protect, Detect, Respond, and Recover – each with practical steps schools can take to improve their cybersecurity posture. From implementing robust access controls to developing comprehensive incident response plans, NIST CSF 2.0 empowers schools to build a resilient cybersecurity program tailored to their specific needs and resources.
We’ll explore how each function applies to the unique challenges faced by schools, providing concrete examples and addressing common concerns along the way.
NIST CSF 2.0 Framework Overview for Schools
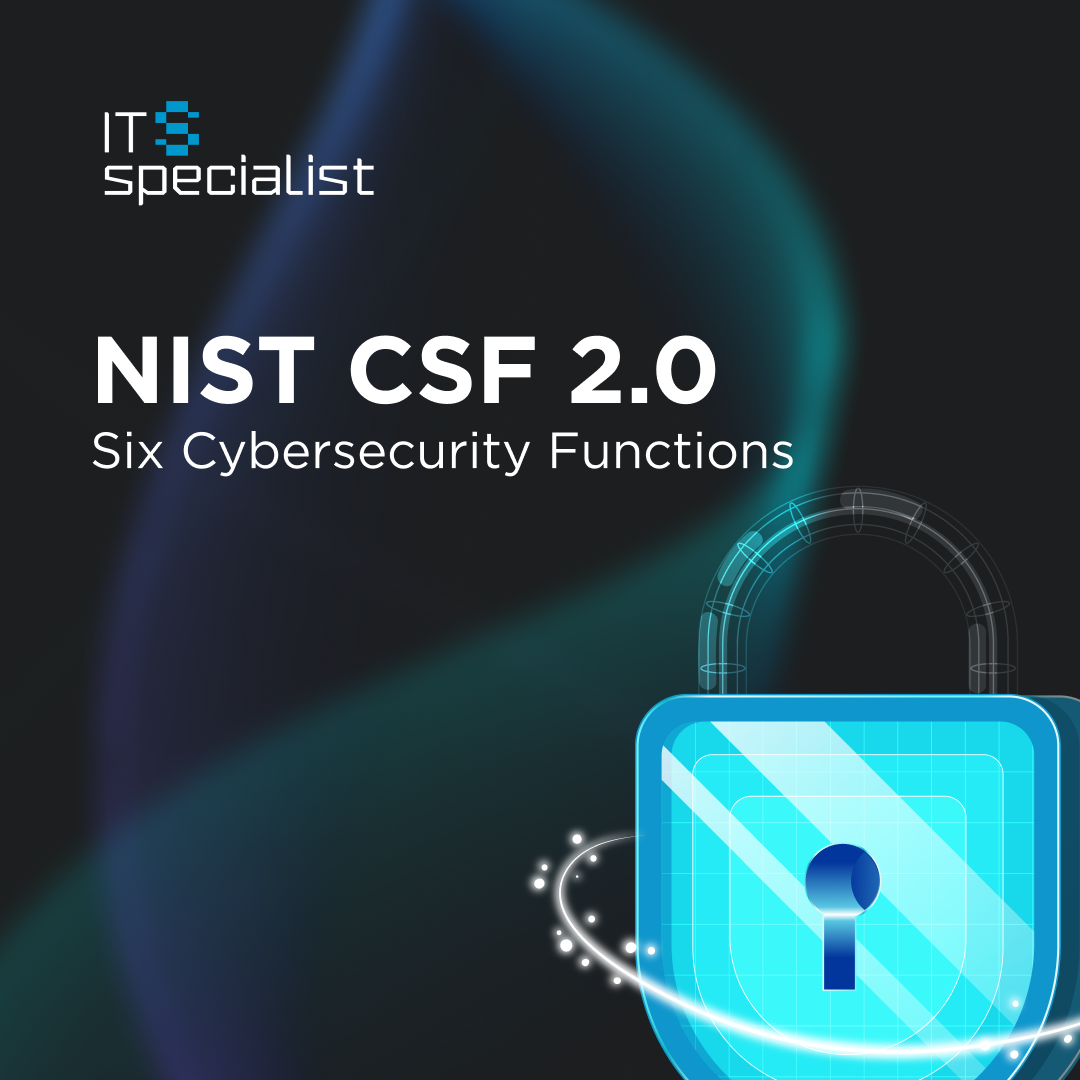
Cybersecurity threats are increasingly sophisticated and prevalent, impacting even educational institutions. The National Institute of Standards and Technology Cybersecurity Framework (NIST CSF) version 2.0 offers a voluntary, adaptable framework to help schools manage and reduce these risks. This framework provides a common language and approach for assessing, managing, and improving cybersecurity posture, regardless of size or resources.
Core Principles of NIST CSF 2.0 for Schools
The NIST CSF 2.0 is built upon a set of core principles that emphasize risk management, flexibility, and continuous improvement. These principles guide the implementation of the framework’s five functions within the unique context of a K-12 school environment. Key among these is the understanding that a school’s cybersecurity posture is not a one-time achievement but an ongoing process of assessment, improvement, and adaptation.
The framework prioritizes a risk-based approach, focusing resources where they are most needed to protect sensitive student and staff data.
The Five Functions of NIST CSF 2.0 in Schools
The NIST CSF 2.0 organizes cybersecurity activities into five core functions: Identify, Protect, Detect, Respond, and Recover. Each function is crucial for building a robust cybersecurity program within a school setting.
Function Implementation Examples in K-12 Schools
The following table illustrates how each function can be implemented in a K-12 school environment. Remember, implementation will vary depending on the specific school’s size, resources, and risk profile.
Function | Specific School Application | Implementation Steps | Potential Challenges |
---|---|---|---|
Identify | Inventory of all school-owned IT assets (computers, servers, network devices, etc.), identification of sensitive data (student records, financial information), assessment of current cybersecurity risks. | Conduct regular IT asset inventories, map network infrastructure, perform data classification, develop a risk assessment methodology, document findings. | Maintaining accurate and up-to-date inventories, classifying data effectively, allocating sufficient resources for risk assessments. |
Protect | Implementation of access controls (user accounts, passwords, multi-factor authentication), security awareness training for staff and students, network security measures (firewalls, intrusion detection systems). | Develop and enforce strong password policies, implement multi-factor authentication, provide regular security awareness training, install and maintain firewalls and intrusion detection systems. | Maintaining employee compliance with security policies, securing all endpoints (laptops, tablets, mobile devices), keeping security software updated. |
Detect | Implementation of security monitoring tools (intrusion detection systems, security information and event management (SIEM) systems), regular security audits and vulnerability scans. | Deploy monitoring tools, configure alerts for suspicious activity, perform regular vulnerability scans, conduct penetration testing (where resources allow). | Analyzing large volumes of security logs, responding effectively to alerts, obtaining necessary expertise for security audits and penetration testing. |
Respond | Development of incident response plan, establishment of communication protocols, procedures for containment and eradication of security incidents. | Develop and regularly test an incident response plan, establish clear communication channels, define roles and responsibilities for incident response. | Ensuring timely and effective response to incidents, managing communication during an incident, addressing potential legal and regulatory implications. |
Recover | Data backup and recovery procedures, disaster recovery planning, business continuity planning. | Implement regular data backups, test data recovery procedures, develop a disaster recovery plan, create a business continuity plan. | Ensuring sufficient data storage capacity, testing recovery procedures effectively, addressing potential disruptions to learning. |
Addressing Specific School Cybersecurity Risks with NIST CSF 2.0
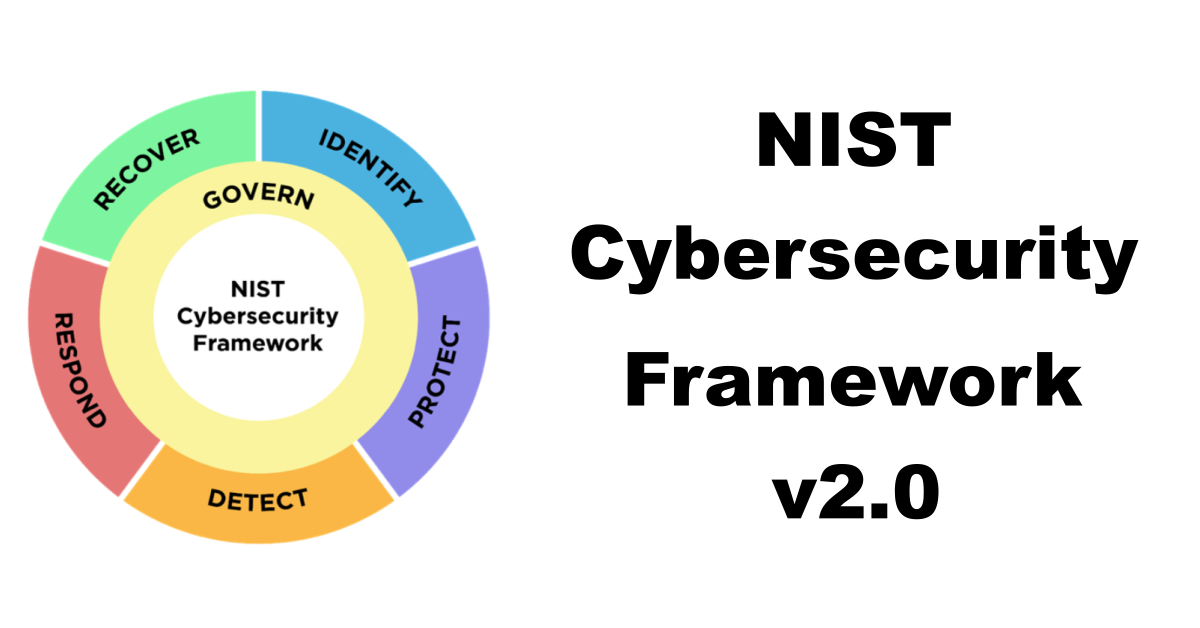
The NIST Cybersecurity Framework (CSF) version 2.0 provides a flexible and adaptable approach to managing cybersecurity risk, making it particularly valuable for schools facing a unique set of challenges. Unlike large corporations with dedicated IT teams, schools often have limited resources and expertise, yet they handle sensitive student and staff data, making them prime targets for cyberattacks. The framework’s focus on risk management and its adaptable nature allows schools to prioritize and address their most pressing cybersecurity concerns effectively.The NIST CSF 2.0 framework helps schools proactively address and mitigate various threats by providing a structured approach to identify, assess, and manage their risks.
It encourages a risk-based approach, meaning schools can focus their limited resources where they are needed most. This is crucial for schools with limited budgets and IT staff.
Common Cybersecurity Threats Facing Schools
Schools face a range of cybersecurity threats, each requiring a different approach for mitigation. Understanding these threats is the first step towards effective cybersecurity. These threats often exploit vulnerabilities in systems and human behavior.
- Ransomware: This malicious software encrypts a school’s data, demanding a ransom for its release. The disruption to learning and the cost of recovery can be devastating.
- Phishing: Deceptive emails or messages designed to trick individuals into revealing sensitive information, such as usernames, passwords, or financial details. This is particularly effective against less tech-savvy individuals.
- Data Breaches: Unauthorized access to sensitive student and staff data, leading to identity theft, financial loss, and reputational damage. This can have severe legal and ethical consequences.
- Malware Infections: The introduction of malicious software onto school computers or networks, potentially leading to data theft, system disruption, or denial-of-service attacks. This can range from simple viruses to sophisticated spyware.
- Insider Threats: Malicious or negligent actions by individuals within the school community, such as employees or students. This can include accidental data leaks or deliberate sabotage.
Mitigating Cybersecurity Threats with NIST CSF 2.0
The NIST CSF 2.0 framework provides a structured approach to mitigate these threats. Its five core functions – Identify, Protect, Detect, Respond, and Recover – provide a roadmap for building a robust cybersecurity posture.
- Identify: Understanding the school’s assets, data, and systems, as well as potential threats and vulnerabilities. This involves asset inventories, vulnerability scans, and risk assessments.
- Protect: Implementing safeguards to limit or contain the impact of a successful cyberattack. This includes access controls, data encryption, and security awareness training.
- Detect: Establishing mechanisms to identify cybersecurity incidents as quickly as possible. This involves intrusion detection systems, security information and event management (SIEM) tools, and regular security monitoring.
- Respond: Having a plan in place to handle a cybersecurity incident effectively and efficiently. This includes incident response teams, communication protocols, and data recovery procedures.
- Recover: Restoring systems and data to normal operations after an incident. This includes backup and recovery procedures, business continuity planning, and post-incident analysis.
Risk Assessment and Management within NIST CSF 2.0 for Schools
A thorough risk assessment is fundamental to implementing the NIST CSF 2.0 framework effectively. This involves identifying potential threats, analyzing their likelihood and impact, and prioritizing mitigation efforts based on risk levels. The framework provides a structured approach to this process, allowing schools to focus their limited resources on the most critical risks. Regular risk assessments are essential to adapt to evolving threats and vulnerabilities.
Sample Risk Assessment Matrix for Schools
The following table provides a sample risk assessment matrix tailored to a school environment. This matrix uses a simplified approach, but the principles can be expanded to encompass a more detailed assessment.
Threat | Likelihood (Low, Medium, High) | Impact (Low, Medium, High) | Risk Level (Low, Medium, High) | Mitigation Strategies |
---|---|---|---|---|
Ransomware Attack | Medium | High | High | Regular backups, employee training, strong antivirus software, network segmentation |
Phishing Attack | High | Medium | High | Security awareness training, email filtering, multi-factor authentication |
Data Breach (Accidental Data Loss) | Medium | Medium | Medium | Access controls, data encryption, regular audits |
Malware Infection | Medium | Medium | Medium | Antivirus software, regular software updates, employee training |
NIST CSF 2.0 and Data Protection in Schools
Protecting student and staff data is paramount for schools, and the NIST Cybersecurity Framework (CSF) version 2.0 provides a robust, adaptable roadmap to achieve this. Its risk-based approach allows schools of all sizes and technical capabilities to implement appropriate security controls, ensuring compliance with regulations like FERPA (Family Educational Rights and Privacy Act) and other relevant state and federal laws.
This framework moves beyond simple compliance to a more proactive and comprehensive approach to data security.The NIST CSF 2.0 framework helps schools establish a strong data protection posture by providing a structured methodology for identifying, assessing, and mitigating cybersecurity risks related to student and staff data. It emphasizes a continuous improvement cycle, enabling schools to adapt their security measures as threats evolve and technology changes.
This proactive approach is crucial in an era of increasingly sophisticated cyberattacks targeting sensitive educational data.
Data Security Controls Aligned with NIST CSF 2.0
Implementing data security controls is a cornerstone of protecting sensitive information. NIST CSF 2.0 offers guidance on various controls that schools can leverage, tailoring them to their specific context and resources. These controls span the entire data lifecycle, from creation to disposal.
- Access Controls: NIST CSF 2.0 promotes the principle of least privilege, meaning users should only have access to the data they need to perform their job. This can be implemented through robust user authentication, authorization mechanisms (role-based access control), and regular access reviews. For example, a teacher should only have access to their students’ grades and not the entire student database.
- Encryption: Data encryption, both in transit and at rest, is crucial for protecting data from unauthorized access. NIST CSF 2.0 recommends employing strong encryption algorithms and key management practices. This ensures that even if data is breached, it remains unreadable without the decryption key. Schools should encrypt all sensitive data, including student records, financial information, and personnel files.
- Data Loss Prevention (DLP): DLP tools and strategies help prevent sensitive data from leaving the school’s controlled environment without authorization. These tools can monitor data movement, identify sensitive information, and block or alert on unauthorized attempts to transfer data. Examples include preventing the emailing of student social security numbers or blocking the use of unauthorized cloud storage services.
Data Backup and Recovery Plans
A comprehensive data backup and recovery plan is essential for business continuity and data resilience. NIST CSF 2.0 emphasizes the importance of regular backups, testing the recovery process, and maintaining offsite backups to protect against data loss due to disasters or cyberattacks.The plan should detail the frequency of backups, the types of data to be backed up, the backup storage location, and the recovery procedures.
Regular testing of the recovery plan ensures its effectiveness and allows for adjustments as needed. For instance, a school might perform a full backup weekly and incremental backups daily, storing one copy onsite and another in a geographically separate, secure location.
Data Lifecycle and Security Controls Flowchart
The following describes a simplified flowchart illustrating the data lifecycle and corresponding NIST CSF 2.0 aligned security controls. Imagine a visual representation with boxes and arrows. Data Creation: Data is created (e.g., student enrollment forms, grades). Security Controls: Access controls are implemented to restrict data creation to authorized personnel. Data encryption is enabled from the outset.
Data Storage: Data is stored securely (e.g., database, file server). Security Controls: Encryption at rest is used. Access controls limit access based on roles. Regular security audits are performed. Data Processing: Data is processed (e.g., generating reports, updating records).
Security Controls: Access controls restrict access to authorized users only. Data monitoring and logging are implemented to detect anomalies. Data Transmission: Data is transmitted (e.g., sharing data with other departments, external agencies). Security Controls: Encryption in transit is used (e.g., HTTPS, VPN). Data loss prevention measures are in place to prevent unauthorized data exfiltration.
Data Disposal: Data is securely disposed of (e.g., deletion, shredding). Security Controls: Secure deletion methods are used to prevent data recovery. Compliance with data retention policies is maintained.
NIST CSF 2.0 and Technology Infrastructure in Schools: How Nist Csf 2 0 Can Help Schools
Protecting a school’s technology infrastructure is paramount, not just for efficient operations, but also for safeguarding sensitive student and staff data. The NIST Cybersecurity Framework (CSF) version 2.0 provides a robust, adaptable model for schools to implement a comprehensive cybersecurity posture. Its flexible nature allows it to be tailored to the specific needs and resources of any school district, from a small rural school to a large urban district.Securing School Networks and Devices with NIST CSF 2.0The NIST CSF 2.0 framework emphasizes a risk-based approach.
Schools should first identify their most critical assets (student data, financial records, learning management systems) and then prioritize their protection efforts accordingly. This involves securing all aspects of the school’s IT infrastructure, including computers, servers, mobile devices, and network infrastructure. The framework’s Identify function guides the process of asset inventory, risk assessment, and business environment understanding. This forms the foundation for subsequent actions.
For example, identifying all devices connected to the school network is crucial for implementing effective security controls. Regular vulnerability scanning and patching, guided by the Protect function, are essential to mitigate known vulnerabilities. The Detect function highlights the importance of intrusion detection systems and security information and event management (SIEM) tools to monitor network traffic and identify suspicious activity.
Finally, the Respond and Recover functions detail incident response planning and disaster recovery procedures, crucial for minimizing the impact of any security breaches.
Secure Configuration and Management of School IT Infrastructure, How nist csf 2 0 can help schools
NIST CSF 2.0 provides specific guidance on secure configuration and management. For example, the framework recommends implementing strong password policies, multi-factor authentication, and regular software updates. It also emphasizes the importance of access control, ensuring that only authorized personnel have access to sensitive data and systems. The framework’s Protect function guides the implementation of these security controls, while the Govern function highlights the need for policies and procedures to ensure consistent application.
A school might use NIST guidelines to implement secure configurations for its Wi-Fi networks, ensuring strong encryption and limiting access to authorized devices. Similarly, server configurations would follow best practices for hardening, reducing the attack surface, and limiting potential damage from vulnerabilities. Regular security audits, as Artikeld in the framework, are essential for verifying the effectiveness of these configurations and identifying any gaps.
Incident Response Planning Based on NIST CSF 2.0
A comprehensive incident response plan is vital for minimizing the impact of cybersecurity incidents. NIST CSF 2.0 provides a structured approach to incident response, encompassing preparation, detection, analysis, containment, eradication, recovery, and post-incident activity. The Respond and Recover functions are central to this process. Schools should develop detailed procedures for handling various types of incidents, including malware infections, phishing attacks, and data breaches.
This includes establishing communication protocols, defining roles and responsibilities, and designating a dedicated incident response team. Regular drills and simulations, informed by the framework’s guidance, are crucial for testing the effectiveness of the plan and ensuring the team’s preparedness. A well-defined plan, based on NIST CSF 2.0, can greatly reduce the impact of a security incident and help a school recover quickly.
Securing School Technologies Using NIST CSF 2.0
The NIST CSF 2.0 framework is applicable to all school technologies. For Learning Management Systems (LMS), this means securing access, regularly updating the software, and implementing strong authentication. For Student Information Systems (SIS), data encryption both in transit and at rest is crucial, along with robust access controls and regular backups. The framework guides the implementation of appropriate security controls for each system, considering its criticality and the sensitivity of the data it handles.
For instance, a school might use the framework to guide the selection and configuration of firewalls and intrusion detection systems to protect its network from external threats, while simultaneously implementing internal controls to prevent unauthorized access to sensitive data within the school’s network. This ensures that all school technologies are secured to an appropriate level, minimizing the risk of data breaches and operational disruptions.
Building a Cybersecurity Culture within Schools using NIST CSF 2.0
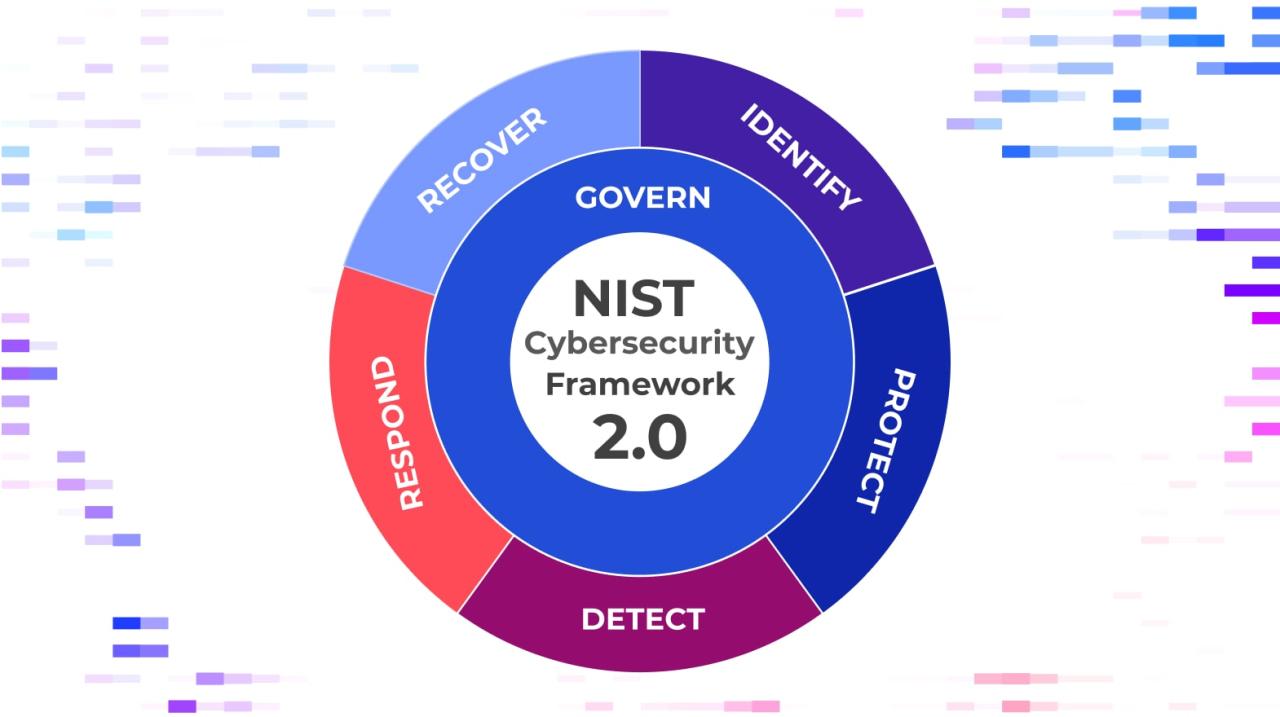
Implementing a robust cybersecurity program isn’t just about technology; it’s about people. NIST CSF 2.0 provides a framework, but its success hinges on cultivating a cybersecurity-aware culture within the school community. This involves educating and training everyone – students, staff, and administrators – to understand and actively participate in protecting school data and systems. A strong cybersecurity culture minimizes risk, improves response times to incidents, and ultimately fosters a safer learning environment.Education and training are the cornerstones of a successful cybersecurity program aligned with NIST CSF 2.0.
The framework’s core functions, such as Identify, Protect, Detect, Respond, and Recover, all require a well-informed workforce. Without proper training, even the most sophisticated security measures can be rendered ineffective by human error. Therefore, a comprehensive training program is crucial for successful implementation.
Education and Training for NIST CSF 2.0 Implementation
A multi-faceted approach to education and training is needed. This includes initial onboarding sessions for new employees and students, regular refresher courses covering updated threats and best practices, and specialized training for IT staff responsible for managing and maintaining the school’s systems. The training should be tailored to the specific roles and responsibilities of each individual, focusing on practical application of NIST CSF 2.0 principles.
For example, teachers might focus on safe online practices for students, while IT staff might receive in-depth training on incident response procedures. Regular phishing simulations and security awareness quizzes can reinforce learning and identify areas needing further attention. This ongoing training should align with the school’s overall cybersecurity plan, which is itself informed by the NIST CSF 2.0 framework.
Successful implementation relies on continuous learning and adaptation to the ever-evolving threat landscape.
Fostering a Cybersecurity-Aware Culture
Creating a cybersecurity-aware culture requires a proactive and multi-pronged approach. It’s not enough to simply provide training; the school needs to actively promote and reinforce cybersecurity best practices throughout its daily operations. This can involve incorporating cybersecurity education into the curriculum, launching school-wide awareness campaigns, and recognizing and rewarding individuals who demonstrate exemplary security behavior. Regular communication, using various channels, is essential to keep the entire community informed and engaged.
Communicating Cybersecurity Risks and Best Practices
Effective communication is key to building a strong cybersecurity culture. Different stakeholders within a school – students, teachers, administrators, and IT staff – require different levels and types of information. For students, communication should be clear, concise, and age-appropriate, focusing on safe online practices such as password security and responsible social media use. Teachers need to understand their role in protecting student data and reporting suspicious activity.
Administrators need to understand the overall security posture of the school and their responsibilities in overseeing its implementation. IT staff requires detailed information on security protocols and incident response procedures. Using multiple channels – newsletters, emails, posters, school announcements, and workshops – ensures that the message reaches everyone effectively.
Sample Cybersecurity Awareness Training Program
This program incorporates elements from the NIST CSF 2.0 framework:
Module 1: Introduction to Cybersecurity and the NIST CSF 2.0 Framework (Identify function): This module provides a general overview of cybersecurity threats and the importance of the NIST CSF 2.0 framework. It introduces core concepts such as confidentiality, integrity, and availability.
Module 2: Password Security and Account Management (Protect function): This module teaches students and staff how to create strong, unique passwords and practice good account management habits. It emphasizes the importance of multi-factor authentication (MFA) where available.
Module 3: Recognizing and Reporting Phishing and Malware (Detect function): This module focuses on identifying and reporting suspicious emails, websites, and attachments. It includes practical exercises and simulations to reinforce learning.
Module 4: Incident Response Procedures (Respond function): This module Artikels the steps to take in the event of a security incident, such as a data breach or malware infection. It emphasizes the importance of reporting incidents promptly to the appropriate authorities.
Module 5: Data Backup and Recovery (Recover function): This module covers the importance of regular data backups and disaster recovery planning. It explains how to restore data in case of a system failure or data loss.
The training program should be delivered using a variety of methods, including online modules, workshops, and hands-on exercises, to cater to different learning styles. Regular reinforcement through quizzes, newsletters, and updates on current threats ensures ongoing awareness and preparedness.
Ending Remarks
Securing our schools in the digital age is a shared responsibility, and the NIST CSF 2.0 framework provides a powerful tool to achieve that goal. By adopting a proactive, risk-based approach, schools can significantly reduce their vulnerability to cyber threats. Remember, it’s not about achieving perfect security, it’s about continuous improvement and building a culture of cybersecurity awareness. Implementing the NIST CSF 2.0 framework is an investment in the safety and security of our students, staff, and the valuable data entrusted to our care.
Let’s work together to make our schools safer, one step at a time.
Essential Questionnaire
What if my school doesn’t have a dedicated IT department?
NIST CSF 2.0 is adaptable. Even smaller schools with limited resources can benefit. Focus on prioritizing risks and implementing the most critical controls first. Outsourcing some functions or using managed security services are also viable options.
How much will implementing NIST CSF 2.0 cost my school?
The cost varies greatly depending on the school’s size, existing infrastructure, and the level of implementation. It’s an investment, but the potential cost savings from avoiding a data breach far outweigh the initial expenses.
How long will it take to fully implement NIST CSF 2.0?
Implementation is a journey, not a race. Start with a phased approach, focusing on high-priority areas first. Regular reviews and updates are key to maintaining an effective cybersecurity program.