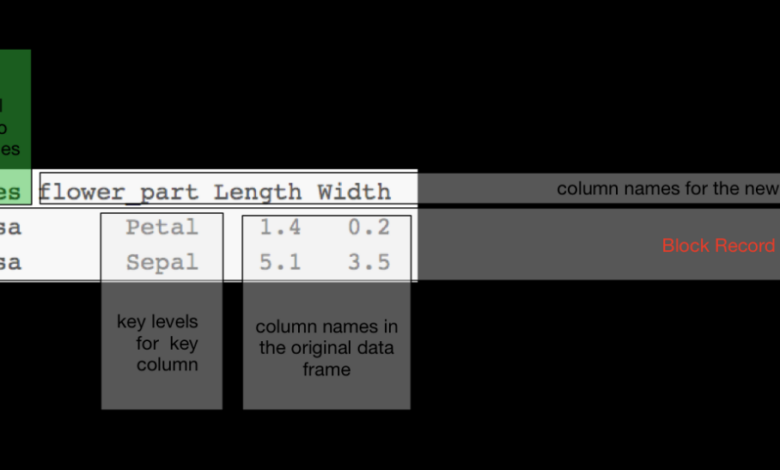
Storing Code in Model Files Using CDATA
Storing code in model files using CDATA: This might sound a bit geeky, but trust me, it’s a fascinating rabbit hole to dive into! We’re talking about embedding code snippets directly within your XML or HTML files using CDATA sections – a clever way to keep things organized and avoid those pesky character encoding issues. But, like any powerful tool, there are security implications to consider.
This post explores the hows, whys, and potential pitfalls of using CDATA for code storage, along with safer alternatives.
We’ll unpack the mechanics of CDATA sections, comparing them to other character escaping methods. We’ll then dive into the security concerns – how vulnerable is this approach to injection attacks? We’ll examine best practices for secure implementation, exploring examples across various programming languages like JavaScript, Python, and SQL. Finally, we’ll weigh CDATA against alternative approaches, like using external files or databases, to help you choose the best method for your project.
Understanding CDATA Sections in XML and HTML
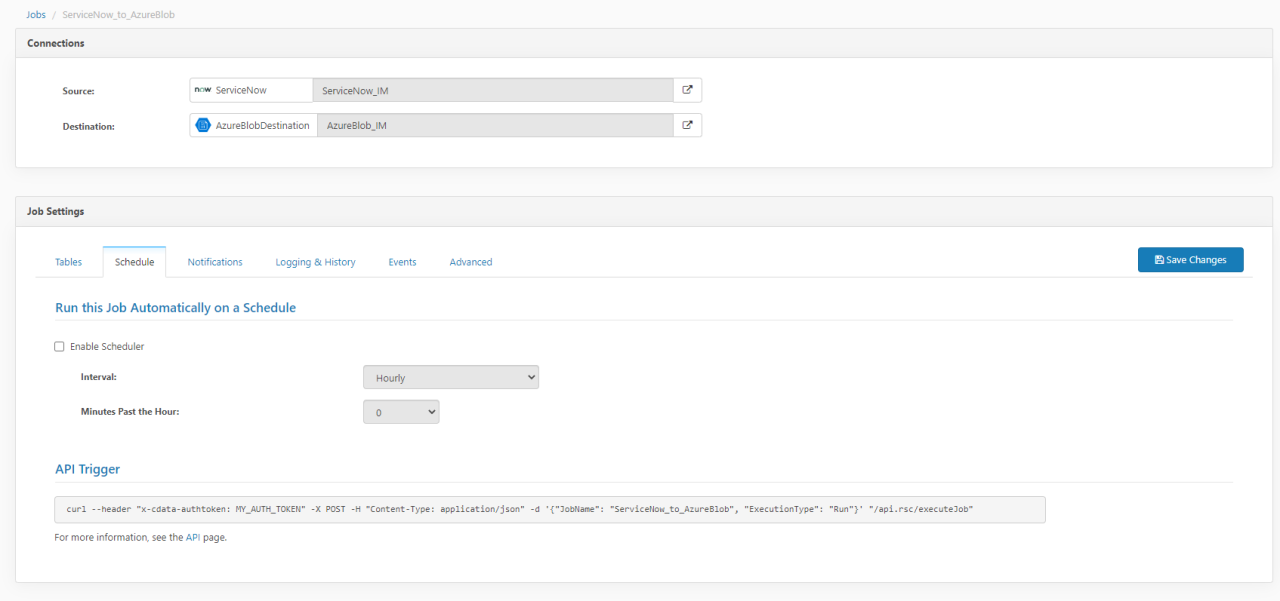
CDATA sections provide a mechanism for including literal text within XML and HTML documents, especially useful when dealing with content that might otherwise be interpreted as markup. This is particularly important when embedding code snippets or text containing characters that have special meanings within the XML or HTML context. Understanding CDATA sections allows developers to avoid the complexities of escaping special characters, resulting in cleaner and more maintainable code.
CDATA Section Purpose and Function, Storing code in model files using cdata
CDATA sections are used to prevent the XML or HTML parser from interpreting certain text content as markup. Any text enclosed within a CDATA section is treated as literal data, regardless of the presence of characters like ` <`, `>`, `&`, or `”`. This is crucial for preserving the integrity of code snippets, scripts, or any text containing characters that would otherwise cause parsing errors or unintended behavior. The parser simply ignores any markup within the CDATA section, treating it as plain text.
CDATA Section Syntax
The syntax for a CDATA section is straightforward. It begins with the marker `
Comparison of CDATA with Other Escaping Methods
Several methods exist for handling special characters in XML and HTML, each with its own strengths and weaknesses. CDATA sections offer a concise alternative to entity encoding, particularly when dealing with large blocks of text containing numerous special characters. While entity encoding is perfectly suitable for individual characters, using it for extensive code snippets can lead to less readable and more error-prone code.
Method | Description | Advantages | Disadvantages |
---|---|---|---|
CDATA Section | Treats enclosed text as literal data, ignoring markup. | Simple syntax, suitable for large blocks of text, avoids numerous entity escapes. | Cannot contain the sequence `]]>`, potentially less readable for small snippets. |
Entity Encoding | Replaces special characters with their corresponding entities (e.g., `<` for `<`). | Widely supported, handles individual special characters effectively. | Can become cumbersome for large blocks of text with many special characters, reduces readability. |
Numeric Character References | Represents characters using their Unicode code points (e.g., `<` for `<`). | Similar to entity encoding, provides an alternative for characters without predefined entities. | Less readable than entity encoding, can be cumbersome for large text blocks. |
Beneficial Scenarios for Storing Code Snippets with CDATA
CDATA sections are particularly useful when embedding code snippets within XML or HTML documents. For example, consider storing JavaScript code within an XML configuration file. Using CDATA prevents the XML parser from interpreting the ` <`, `>`, and `&` characters within the JavaScript code as XML markup, ensuring the JavaScript code remains intact and functional. Another example would be embedding SQL queries in an XML document used for database interactions; CDATA sections ensure the SQL remains correctly parsed by the database system.
Using CDATA sections simplifies the process of embedding code snippets within XML and HTML documents, improving code readability and reducing the risk of parsing errors.
Security Implications of Storing Code in CDATA Sections
Storing code within CDATA sections in XML or HTML might seem like a convenient way to embed scripts or configurations, but it’s crucial to understand the inherent security risks. While CDATA sections prevent the XML/HTML parser from interpreting the enclosed content as markup, they do not inherently sanitize or validate that content. This leaves the door open for various vulnerabilities.
Potential Injection Attacks
Improperly handled CDATA sections can be exploited through various injection attacks. For instance, if user-supplied code is directly inserted into a CDATA section without proper sanitization, an attacker could inject malicious scripts. Consider a scenario where a web application allows users to input JavaScript code within a CDATA section to customize their experience. If the application fails to validate and sanitize this input, an attacker could inject malicious JavaScript to steal cookies, redirect users to phishing sites, or execute arbitrary code on the victim’s browser.
This is essentially a classic Cross-Site Scripting (XSS) vulnerability, even though the code is technically within a CDATA section. The CDATA section merely prevents the XML/HTML parser from interpreting the malicious code as markup; it doesn’t neutralize its harmful effects.
Code Sanitization and Validation Best Practices
Preventing these attacks requires robust sanitization and validation of any code stored within CDATA sections. This means more than just escaping special characters. A multi-layered approach is recommended. First, input validation should be implemented to ensure that the user-supplied code adheres to predefined rules and formats. This might involve checking the length, allowed characters, and overall structure of the code.
Second, escaping or encoding any potentially harmful characters within the code itself is crucial. This helps prevent the code from being interpreted as HTML or JavaScript commands. Finally, output encoding should be used when displaying the code to the user, ensuring that any special characters are properly rendered as text and not interpreted as executable code.
Secure Handling of User-Supplied Code
A secure approach to handling user-supplied code embedded within CDATA sections involves a combination of techniques. Firstly, never trust user input. Always treat user-supplied code as potentially malicious. Secondly, implement a strict whitelist approach, allowing only specific, pre-approved code snippets or functions. This significantly reduces the attack surface.
Thirdly, use a robust sanitization library or function specifically designed to handle the type of code being stored (e.g., a JavaScript sanitizer if you’re storing JavaScript code). Finally, consider using a sandboxed environment to execute user-supplied code. This isolates the code from the rest of the application, minimizing the impact of any potential vulnerabilities.
Examples of Secure Coding Practices
Consider a hypothetical scenario where a web application allows users to define custom CSS styles within a CDATA section. A secure implementation would involve: 1) Validating the input to ensure it conforms to CSS syntax and contains no malicious code (e.g., using regular expressions to check for prohibited s or patterns). 2) Escaping any special characters within the CSS code to prevent XSS attacks.
3) Encoding the output before displaying the styles on the webpage, ensuring that the browser interprets the code as styles and not as executable JavaScript. Another example could involve a configuration file where users can define custom settings within CDATA sections. A secure approach would involve using a configuration parser that specifically validates the syntax and structure of the configuration data, preventing the injection of malicious commands.
This approach should also implement appropriate input validation and output encoding, preventing potential vulnerabilities.
Practical Applications and Examples: Storing Code In Model Files Using Cdata
CDATA sections offer a straightforward method for embedding various data types within XML and HTML, including code snippets from different programming languages. This capability proves particularly useful when dealing with dynamic content or configurations that need to be integrated directly into the markup. This section explores practical applications and provides examples demonstrating the process of embedding and retrieving code from CDATA sections.
The core benefit lies in preventing the XML or HTML parser from interpreting the embedded code as markup. This avoids potential conflicts and ensures the code remains intact, ready for execution or processing by the appropriate interpreter.
Embedding and Retrieving Code from Different Programming Languages
CDATA sections can seamlessly accommodate code from various languages like JavaScript, Python, and SQL. The key is to treat the code as plain text within the CDATA section, preventing the XML/HTML parser from interfering. Consider the following examples:
JavaScript within HTML:
<script>
<![CDATA[
function myJavaScriptFunction()
alert("This is a JavaScript function within a CDATA section!");
]]>
</script>
Python within XML: Imagine an XML configuration file for a Python application. The Python code defining a specific task could be embedded within a CDATA section.
<configuration>
<task>
<code>
<![CDATA[
import sys
print("This Python code is executed from within an XML configuration file.")
]]>
</code>
</task>
</configuration>
SQL within XML: A similar approach can be used to store SQL queries within an XML file, for example, for a database configuration.
<database>
<query>
<![CDATA[
SELECT
- FROM users WHERE id = 1;
]]>
</query>
</database>
Retrieving and executing this code requires parsing the XML or HTML document, extracting the CDATA section content, and then using the appropriate interpreter (e.g., a JavaScript engine for JavaScript code, a Python interpreter for Python code, etc.).
Advantages and Disadvantages of Storing Code in CDATA Sections
Weighing the pros and cons is crucial before adopting this approach. The decision depends on the specific application and context.
- Advantages:
- Simplicity: Easy to implement and understand.
- Direct Embedding: Allows direct inclusion of code within the markup.
- Preservation of Code Integrity: Prevents XML/HTML parser from interfering with the code.
- Disadvantages:
- Security Risks: Potentially vulnerable to cross-site scripting (XSS) attacks if not handled carefully (as previously discussed).
- Maintainability: Can become difficult to maintain for large code blocks.
- Limited Functionality: Does not provide sophisticated code management or version control.
Storing a JavaScript Function in HTML using CDATA
This example demonstrates the practical implementation of embedding a JavaScript function within an HTML file using a CDATA section.
<!DOCTYPE html>
<html>
<head>
<title>CDATA Example</title>
</head>
<body>
<script>
<![CDATA[
function greet(name)
alert("Hello, " + name + "!");
greet("World");
]]>
</script>
</body>
</html>
Implementing CDATA Sections for XML Configuration Data
This Artikels a step-by-step procedure for embedding configuration data in an XML file using CDATA sections.
- Define the XML Structure: Design the XML structure to accommodate the configuration data. Use elements to represent different configuration parameters.
- Wrap Configuration Data in CDATA: Enclose the configuration data within CDATA sections to prevent XML parser interpretation.
- XML File Creation: Create the XML file and populate it with the structured data, including the CDATA sections.
- XML Parsing: Use an XML parser (available in most programming languages) to read and parse the XML file.
- Data Extraction: Extract the configuration data from the CDATA sections.
- Data Usage: Use the extracted configuration data to configure your application.
Alternative Approaches and Comparisons
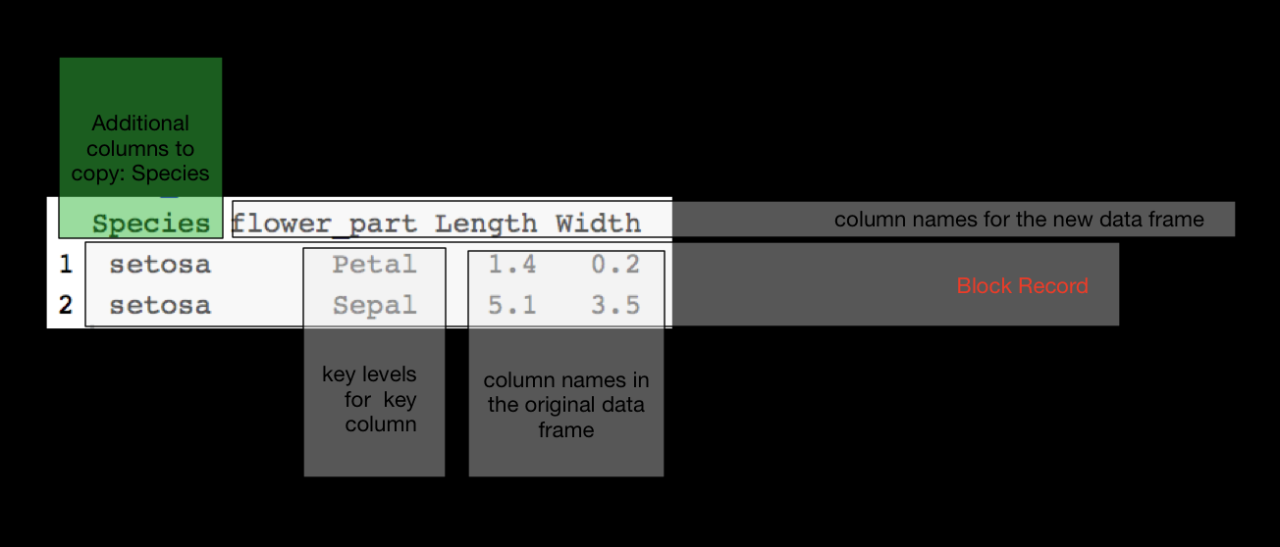
Storing code within CDATA sections, while seemingly convenient, isn’t always the best approach. Let’s explore alternative methods and weigh their pros and cons against using CDATA. The optimal choice depends heavily on the project’s specific needs and priorities.
Several methods exist for managing external code, each offering a unique balance of security, maintainability, and performance. Understanding these differences is crucial for making informed decisions.
Storing Code in External Files
Storing code in separate files (e.g., `.js`, `.css`, `.py`) offers several advantages. Security improves because the code isn’t directly embedded within the XML or HTML document, reducing the risk of cross-site scripting (XSS) vulnerabilities. Maintainability also benefits; changes to the code don’t require modifying the main document, simplifying updates and version control. Performance can be slightly improved, especially for large codebases, as the browser doesn’t need to parse the code inline.
However, managing many external files can become complex, requiring careful organization and potentially increasing the number of HTTP requests.
For example, instead of embedding JavaScript code within a CDATA section in an XML configuration file, you would create a separate `script.js` file. The XML would then reference this file using a standard ` ` tag (in HTML) or a similar mechanism in other contexts. This approach is especially beneficial for larger projects or when collaboration is involved, as multiple developers can work on different code files simultaneously.
Storing Code in Databases
Using a database (e.g., MySQL, PostgreSQL, MongoDB) provides a centralized and structured approach for managing code. This method is particularly suitable for dynamic code generation or when code needs to be versioned or accessed programmatically. Security can be enhanced through database access controls and encryption. Maintainability is improved through database management tools and version control systems. However, database interactions add overhead, potentially impacting performance, especially for frequent code updates or retrieval.
Imagine a scenario where your application generates custom JavaScript snippets based on user preferences. Storing these snippets in a database allows for easy retrieval and customization. The application can query the database, retrieve the relevant code, and then dynamically inject it into the HTML document. This approach is scalable and allows for efficient management of a large number of code snippets.
Comparison Table
A summary table highlights the key differences between the three methods:
Method | Security | Maintainability | Performance | Best Use Cases |
---|---|---|---|---|
CDATA Sections | Lower (XSS risk) | Lower (requires main document modification) | Can be slower for large codebases | Small, static code snippets within a single document |
External Files | Higher | Higher | Generally faster for large codebases | Larger codebases, collaborative projects, version control |
Databases | High (with proper access controls) | High (database management tools) | Can be slower for frequent updates | Dynamic code generation, versioning, programmatic access |
Factors to Consider When Choosing a Method
The optimal method depends on several factors. Security concerns should always be paramount, particularly when dealing with user-supplied code. Maintainability is crucial for long-term project success, considering ease of updates, collaboration, and version control. Performance implications, especially for large codebases or high-traffic applications, should be carefully assessed. The complexity of implementation and the overall project architecture also play significant roles.
For instance, using a database adds complexity but offers significant benefits in dynamic environments.
Illustrative Examples (without image links)
Let’s explore some concrete scenarios demonstrating the benefits and risks associated with storing code within CDATA sections. These examples will highlight both performance improvements and potential security vulnerabilities.
JavaScript Code Optimization for Webpage Performance
Imagine a complex web application heavily reliant on JavaScript for interactive elements and dynamic content updates. This application includes a large JavaScript library, perhaps 50,000 lines of code, responsible for handling user interactions, data visualizations, and AJAX calls. Instead of embedding this script directly within the HTML, we can place it within a CDATA section. This prevents the HTML parser from interpreting the JavaScript code as HTML markup, leading to faster parsing and rendering.
The browser’s JavaScript engine then handles the code separately, leading to a performance boost, particularly noticeable on lower-end devices or slower internet connections. The improvement might be a reduction in initial page load time by several seconds, depending on the network conditions and the user’s device capabilities. The perceived speed of interaction and overall user experience is enhanced.
SQL Injection Vulnerability and Prevention
Consider a web application that uses SQL queries to fetch data from a database. Suppose the application allows users to input search terms, which are then incorporated directly into an SQL query stored within a CDATA section within the XML configuration file. If a malicious user enters a crafted input string containing SQL injection code, such as `’; DROP TABLE users; –`, the CDATA section won’t protect against this attack.
The SQL parser will still interpret the malicious code, potentially leading to a devastating database compromise. To prevent this, the application must use parameterized queries or prepared statements. These techniques separate the user input from the SQL code, preventing the injection of malicious commands. The database query should be constructed using parameterized queries, where the user-supplied data is treated as a parameter rather than part of the SQL command itself.
This prevents the injection of arbitrary SQL code.
CDATA Section Parsing: A Textual Representation
Let’s visualize how an XML or HTML parser handles a CDATA section. Imagine a simple XML snippet:“`xml HTML-like tags which are not interpreted as markup.]]> “`The parser, upon encountering ` HTML-like tags which are not interpreted as markup.” as the value of the ` ` element. No HTML parsing is performed on the content within the CDATA section. The parser treats it as a single block of text.
Concluding Remarks
So, is storing code in CDATA sections the right choice for you? The answer, as with most things in tech, is “it depends.” While CDATA offers a convenient way to embed code directly within your documents, the security implications can’t be ignored. Weigh the convenience against the potential risks, and remember that thorough sanitization and validation are crucial if you choose this path.
Exploring alternative methods like external files or databases should always be part of the decision-making process. Ultimately, selecting the best approach hinges on balancing convenience, security, and maintainability for your specific project.
Common Queries
What happens if I don’t close a CDATA section correctly?
An improperly closed CDATA section can lead to parsing errors and unexpected behavior. The parser might treat the remaining content as regular XML/HTML, potentially causing errors or security vulnerabilities.
Can I store binary data in CDATA?
No, CDATA is designed for text data. Attempting to store binary data directly within a CDATA section will likely result in corruption or errors.
Are there performance implications to using CDATA for large code blocks?
For extremely large code blocks, using external files might be more efficient. While CDATA itself doesn’t inherently impact performance significantly, excessively large embedded code can slow down parsing.