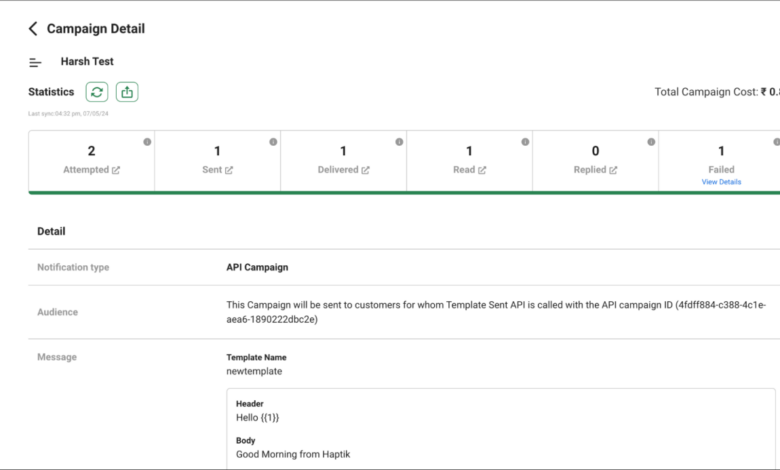
Campaign REST API V1 and V2 A Deep Dive
Campaign REST API V1 and V2: Navigating these two API versions can feel like exploring two different worlds. While both offer ways to manage campaigns, understanding their differences—from data structures and authentication methods to endpoint functionalities and error handling—is crucial for efficient development. This guide delves into the core aspects of both V1 and V2, highlighting key distinctions and providing practical examples to help you choose and effectively utilize the right version for your needs.
We’ll compare and contrast the functionalities, explore authentication mechanisms, examine endpoint usage with code examples, discuss rate limiting and performance optimization, and analyze data structures and response formats. We’ll also provide a migration guide for those upgrading from V1 to V2, along with a detailed workflow for creating a new campaign using the latest V2 API. Whether you’re a seasoned developer or just starting out, this comprehensive guide will equip you with the knowledge you need to master the Campaign REST API.
API Version Comparison
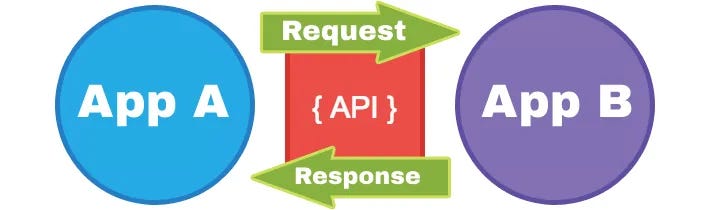
Upgrading our campaign REST API from V1 to V2 represents a significant leap forward in functionality and efficiency. This post details the key differences between the two versions, guiding you through a smooth migration process. Understanding these changes will allow you to leverage the improved features and performance of V2.
Functionalities of V1 and V2
Version 2 offers expanded capabilities compared to V1. V1 primarily focused on core campaign creation, management, and retrieval. It lacked features like real-time analytics dashboards and granular campaign segmentation options. V2, however, introduces robust analytics reporting, allowing for deeper insights into campaign performance. Furthermore, V2 supports advanced segmentation, enabling more targeted campaign delivery based on user demographics and behavior.
The improved automation features in V2 also streamline campaign workflows, reducing manual intervention.
Data Structure Differences
The data structures between V1 and V2 have undergone considerable changes to accommodate the new functionalities. V1 used a simpler, less nested JSON structure. For instance, campaign data in V1 was represented as a flat JSON object, while V2 employs a more hierarchical structure, organizing data into logical groups and sub-objects. This hierarchical approach enhances data readability and facilitates easier access to specific information.
For example, campaign analytics in V2 are now nested within a dedicated “analytics” object, making it easier to parse and utilize. The shift also reflects a move towards a more standardized and extensible data model.
Deprecated Endpoints and Features in V1
Several endpoints and features in V1 have been deprecated in favor of improved alternatives in V2. Specifically, the `/campaigns/report` endpoint in V1, which provided basic campaign performance metrics, has been replaced by the more comprehensive `/campaigns/campaignId/analytics` endpoint in V2. Additionally, the legacy authentication method using API keys in V1 has been replaced with a more secure OAuth 2.0 based authentication system in V2.
These changes reflect a commitment to enhancing security and providing more robust functionality.
Migration Guide from V1 to V2
Migrating from V1 to V2 involves several key steps. First, review the updated API documentation for V2 to familiarize yourself with the new endpoints, data structures, and authentication methods. Next, update your client-side code to reflect the changes in API calls and data parsing. Pay close attention to the deprecated endpoints and replace them with their V2 equivalents.
Thorough testing is crucial to ensure seamless functionality after migration. Finally, monitor your migrated campaigns closely to identify and address any unexpected issues. A phased rollout approach, starting with a subset of campaigns, is recommended to minimize disruption.
Feature Comparison Table
Feature | V1 | V2 |
---|---|---|
Authentication | API Keys | OAuth 2.0 |
Campaign Creation | Basic | Advanced, including segmentation options |
Analytics | Basic reporting | Real-time dashboards and granular metrics |
Data Structure | Flat JSON | Hierarchical JSON |
Error Handling | Basic error codes | Detailed error messages with context |
Segmentation | Limited | Advanced, based on demographics and behavior |
Automation | Limited | Robust workflow automation |
Authentication and Authorization Mechanisms
Securing our campaign APIs, both v1 and v2, is paramount. This section details the authentication and authorization methods employed, ensuring only authorized clients can access and manipulate campaign data. We’ve implemented a robust system to balance ease of use with stringent security protocols.
Both API versions support a range of authentication methods to cater to diverse client needs and security preferences. Authorization, on the other hand, is handled through a consistent, role-based access control system, regardless of the API version used. This approach provides a predictable and secure way to manage access permissions to sensitive campaign information.
Supported Authentication Methods
Both API v1 and v2 primarily utilize API keys for authentication. API keys are unique identifiers assigned to each client application. These keys are included in the request headers to authenticate the client’s identity. While straightforward, API keys are less flexible than OAuth 2.0. Therefore, API v2 also incorporates OAuth 2.0, offering a more granular and secure authentication mechanism, especially beneficial for applications requiring user-level authorization.
API v1, however, relies solely on API keys.
Authorization Schemes
Access control to campaign resources is managed through a role-based access control (RBAC) system. Each client application is assigned one or more roles, defining the level of access granted. For example, a “read-only” role might allow access to campaign performance data but not the ability to modify campaign settings. A “manager” role, on the other hand, would have complete control over the campaign.
So I’ve been diving deep into the differences between Campaign REST API v1 and v2, trying to figure out the best approach for my next project. The upgrade options are significant, especially considering how this impacts the backend. Understanding the implications is crucial, and it made me think about the broader context of app development; check out this great article on domino app dev the low code and pro code future for some insightful perspectives.
Ultimately, choosing between v1 and v2 for my campaign API will depend on how it integrates with the overall app architecture and development strategy.
This RBAC system applies consistently to both API v1 and v2, providing a unified and predictable security model. The specific roles and their associated permissions are configurable and managed through the dedicated campaign management console.
Obtaining and Managing API Credentials, Campaign rest api v1 and v2
To obtain API credentials (API keys for both versions, and OAuth 2.0 client credentials for v2), developers must register their applications through the developer portal. This process involves providing basic application information and agreeing to the terms of service. Upon successful registration, the portal provides the necessary API keys and instructions on how to generate OAuth 2.0 access tokens.
API keys should be treated as sensitive information and securely stored, avoiding hardcoding them directly within client applications. The developer portal also offers tools to manage and revoke API keys as needed, providing an additional layer of security. OAuth 2.0 access tokens have a limited lifespan and should be refreshed periodically as per the OAuth 2.0 specification.
Secure Authentication Flow for a Client Application (API v2 using OAuth 2.0)
A secure authentication flow for a client application using API v2 and OAuth 2.0 would typically involve these steps:
- The client application initiates the OAuth 2.0 authorization flow, typically using the authorization code grant type.
- The authorization server (our API) redirects the client to a login page where the user authenticates.
- Upon successful authentication, the authorization server grants an authorization code to the client application.
- The client application exchanges the authorization code for an access token and refresh token.
- The client application includes the access token in subsequent API requests to access protected resources.
- When the access token expires, the client application uses the refresh token to obtain a new access token.
This flow ensures that the client application only accesses resources on behalf of the authenticated user and that access is controlled through the defined roles and permissions. This is a significantly more secure approach compared to simply using API keys.
Endpoint Functionality and Usage
This section dives into the practical aspects of using our campaign REST APIs, focusing on version 2 (V2) while highlighting key differences from version 1 (V1). We’ll explore the core functionalities – creating, retrieving, updating, and deleting campaign resources – through illustrative HTTP requests and code examples using Python. Understanding these endpoints is crucial for effective integration with our platform.
The V2 API offers significant improvements over V1, primarily in terms of efficiency and data structure. While V1 provides basic functionality, V2 incorporates features like nested resources and improved error handling for a more robust and streamlined experience. We will demonstrate how to leverage these advantages through practical examples.
HTTP Request Examples for Campaign Resource Management (V2)
The following examples illustrate how to interact with campaign resources using standard HTTP methods. These examples assume a base URL of `https://api.example.com/v2/campaigns`. Replace placeholders like `campaign_id` with actual values.
Remember to include appropriate authentication headers in all requests, as detailed in the Authentication and Authorization Mechanisms section.
- GET /campaigns/campaign_id: Retrieve a specific campaign.
curl -X GET "https://api.example.com/v2/campaigns/123" -H "Authorization: Bearer YOUR_API_TOKEN"
- POST /campaigns: Create a new campaign. The request body would contain campaign details in JSON format.
curl -X POST "https://api.example.com/v2/campaigns" -H "Authorization: Bearer YOUR_API_TOKEN" -H "Content-Type: application/json" -d '"name": "My New Campaign", "budget": 1000'
- PUT /campaigns/campaign_id: Update an existing campaign.
curl -X PUT "https://api.example.com/v2/campaigns/123" -H "Authorization: Bearer YOUR_API_TOKEN" -H "Content-Type: application/json" -d '"name": "Updated Campaign Name", "budget": 1500'
- DELETE /campaigns/campaign_id: Delete a campaign.
curl -X DELETE "https://api.example.com/v2/campaigns/123" -H "Authorization: Bearer YOUR_API_TOKEN"
Python Code Examples
This section provides Python code snippets demonstrating interaction with key endpoints in both API versions. We’ll utilize the `requests` library. Remember to install it using pip install requests
.
Error handling is crucial. We’ll show how to gracefully handle common HTTP status codes.
V2 Example (Creating a Campaign):
import requestsurl = "https://api.example.com/v2/campaigns"headers = "Authorization": "Bearer YOUR_API_TOKEN", "Content-Type": "application/json"data = "name": "My Python Campaign", "budget": 2000try: response = requests.post(url, headers=headers, json=data) response.raise_for_status() # Raise HTTPError for bad responses (4xx or 5xx) print(response.json())except requests.exceptions.RequestException as e: print(f"An error occurred: e")
V1 Example (Retrieving a Campaign – Illustrative, specific details omitted for brevity):
#Similar structure to V2, but with different endpoint and potentially different data structure.#Illustrative only, specific V1 endpoint and data handling would be detailed separately.
Common Use Cases and Code Examples
This section Artikels common use cases and demonstrates how to accomplish them using the API.
The examples below use simplified versions for clarity; real-world scenarios might involve more complex data structures and error handling.
Use Case | API Version | Code Snippet (Illustrative – Python) |
---|---|---|
Create a new campaign | V2 | (See V2 example above) |
Get campaign details | V2 | response = requests.get(f"https://api.example.com/v2/campaigns/campaign_id", headers=headers) |
Update campaign budget | V2 | data = "budget": 2500; response = requests.put(f"https://api.example.com/v2/campaigns/campaign_id", headers=headers, json=data) |
Delete a campaign | V2 | response = requests.delete(f"https://api.example.com/v2/campaigns/campaign_id", headers=headers) |
Error Handling Techniques
Robust error handling is essential for any API integration. The following table illustrates how to handle common HTTP response codes.
HTTP Status Code | Meaning | Python Handling Example |
---|---|---|
400 Bad Request | The request was invalid. | if response.status_code == 400: print(f"Bad Request: response.json().get('message', 'Unknown error')") |
404 Not Found | The requested resource was not found. | if response.status_code == 404: print("Campaign not found") |
500 Internal Server Error | An unexpected error occurred on the server. | if response.status_code == 500: print("Internal server error. Please try again later.") |
Rate Limiting and Performance Considerations: Campaign Rest Api V1 And V2
API performance and stability are paramount, especially when dealing with multiple versions. Understanding rate limits and employing optimization strategies are crucial for a smooth user experience and efficient resource management. This section details the rate limiting policies for both API v1 and v2, along with best practices for maximizing performance and handling potential rate limit exceedances.
API Rate Limits
API v1 imposes a rate limit of 100 requests per minute per IP address. API v2, designed for higher throughput, allows 500 requests per minute per IP address. These limits are implemented to prevent abuse and ensure fair resource allocation among all users. Exceeding these limits will result in a temporary HTTP 429 “Too Many Requests” response.
Strategies for Optimizing API Calls
Several techniques can significantly improve API call performance. Caching frequently accessed data is a powerful method, reducing the load on the server and speeding up response times. Batching multiple requests into a single call whenever possible also minimizes overhead. Efficient data filtering and pagination on the server-side minimize the amount of data transferred, further enhancing speed. Finally, using appropriate HTTP methods (GET for retrieval, POST for creation, PUT for updates, DELETE for removal) helps maintain efficiency and clarity.
Handling Rate Limit Exceedances
When a rate limit is exceeded, the API will return a 429 response with information about the remaining requests and the reset time. Robust applications should implement retry mechanisms with exponential backoff. This involves waiting an increasing amount of time between retries after each failed attempt, helping to avoid overwhelming the server. Implementing a queue to manage requests exceeding the rate limit is also a good practice.
Informative error messages to the end-user are also important, explaining the reason for the delay and estimated time of resolution.
Expected Response Times
The following table Artikels the expected response times for various API endpoints in both API versions. These times are averages under normal load conditions and may vary based on factors like network latency and server load.
Endpoint | API v1 (ms) | API v2 (ms) | Description |
---|---|---|---|
/users | 150 | 100 | Retrieve a list of users |
/users/id | 80 | 60 | Retrieve a specific user by ID |
/products | 200 | 150 | Retrieve a list of products |
/products/id | 120 | 90 | Retrieve a specific product by ID |
Data Structures and Response Formats
Understanding the data structures used in our Campaign REST APIs, both V1 and V2, is crucial for successful integration. Both versions primarily utilize JSON (JavaScript Object Notation) for data exchange due to its lightweight nature, human-readability, and widespread support across various programming languages. While both versions employ JSON, there are key differences in the structure and specific fields included in the responses, reflecting improvements and additions made in V2.
JSON Data Structures in API V1
The Campaign API V1 uses a relatively straightforward JSON structure. For instance, retrieving a single campaign might return a response like this: "campaignId": 123, "name": "Summer Sale Campaign", "startDate": "2024-06-15", "endDate": "2024-07-15", "budget": 10000, "status": "active"
This structure is consistent across many endpoints, with variations in fields depending on the specific request. For example, fetching a list of campaigns would return an array of such objects. Error responses follow a similar pattern, with an added “error” field containing a description of the issue.
JSON Data Structures in API V2
API V2 builds upon V1, adding more granular detail and improved organization. The same campaign data might now be represented as: "campaign": "id": 123, "name": "Summer Sale Campaign", "dates": "start": "2024-06-15", "end": "2024-07-15" , "budget": "amount": 10000, "currency": "USD" , "status": "active", "metrics": "impressions": 15000, "clicks": 500, "conversions": 100
Notice the nested structure, separating date and budget information for better organization. Crucially, V2 introduces a “metrics” object, providing campaign performance data directly in the response. This eliminates the need for separate API calls to retrieve performance statistics.
API Response Examples and Changes
The addition of the “metrics” object in V2 exemplifies a significant change. V1 required separate calls to obtain performance data, leading to more requests and potentially increased latency. V2 streamlines this by integrating performance data into the main campaign response. Furthermore, V2 uses more descriptive field names (e.g., “amount” and “currency” within the “budget” object) enhancing readability and clarity.
Parsing API Responses in Python
Python, with its extensive libraries, makes parsing JSON responses straightforward. Using the `requests` and `json` libraries, we can easily handle both V1 and V2 responses. import requestsimport json# Example for V2 (adaptable for V1)response = requests.get("https://api.example.com/v2/campaigns/123")data = response.json()campaign_name = data['campaign']['name']campaign_budget = data['campaign']['budget']['amount']campaign_impressions = data['campaign']['metrics']['impressions']print(f"Campaign Name: campaign_name")print(f"Campaign Budget: campaign_budget")print(f"Campaign Impressions: campaign_impressions")
This code snippet demonstrates how to fetch a campaign, parse the JSON response, and access specific fields. Error handling (checking `response.status_code`) should always be included in production code. The adaptability of this code to V1 is highlighted by the simple removal of nested fields, demonstrating the similar approach to both API versions despite structural differences.
Documentation and Support Resources
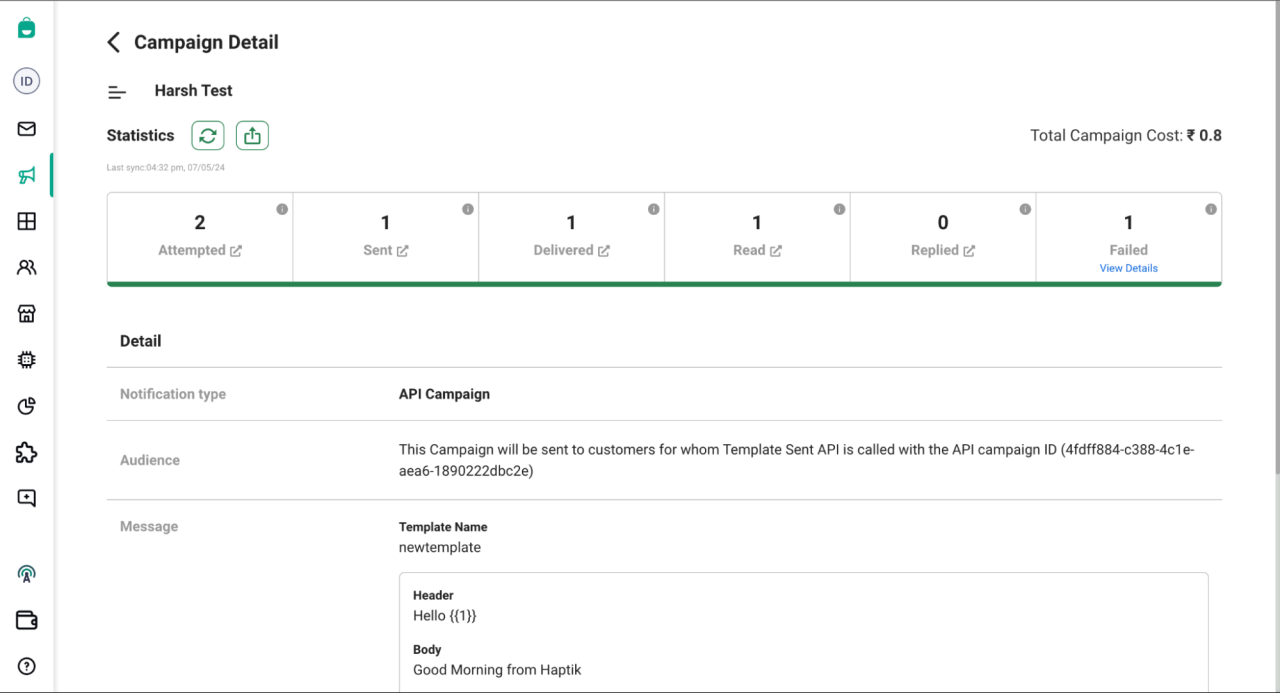
Navigating the Campaign REST API, whether version 1 or 2, requires readily available and comprehensive documentation. Understanding the available resources is crucial for developers to efficiently integrate and utilize the API’s functionalities. This section details the documentation and support channels provided to ensure a smooth developer experience.This section provides an overview of the documentation and support resources available for both API versions (v1 and v2).
We’ll cover the types of documentation provided, helpful developer resources, and the support channels offered for resolving issues. Clear and accessible documentation is vital for successful API integration.
API Documentation
The Campaign REST API v1 documentation is hosted on our internal wiki at [Internal Wiki Link Placeholder – Replace with actual link]. This wiki contains comprehensive guides, tutorials, and detailed descriptions of each endpoint, including request parameters, response codes, and example requests and responses in JSON format. The documentation includes a detailed glossary of terms used within the API, ensuring clarity for developers of all experience levels.
The v2 documentation, while maintaining similar structure and content, has been significantly enhanced with interactive API explorer and improved code samples in multiple programming languages (Python, Java, Node.js, etc.). It’s available at [Internal Wiki Link Placeholder – Replace with actual link]. Both versions offer searchable indexes and a clear hierarchical structure, enabling easy navigation to specific information. The v2 documentation also includes a dedicated section on migration from v1 to v2, highlighting key changes and providing guidance for a seamless transition.
Developer Resources
A collection of resources beyond the core API documentation is available to help developers efficiently use the Campaign REST API. These resources aim to accelerate the integration process and improve the overall developer experience.
- SDKs and Libraries: We provide client SDKs (Software Development Kits) in several popular programming languages (e.g., Java, Python, Node.js). These SDKs simplify API interaction by handling authentication, request formatting, and response parsing, reducing the amount of boilerplate code developers need to write. The SDKs are available for download from our GitHub repository: [GitHub Repository Link Placeholder – Replace with actual link].
- Code Samples and Tutorials: The documentation includes numerous code samples demonstrating how to perform common tasks using the API. These samples are available in multiple programming languages and cover various aspects of API usage, such as creating campaigns, retrieving campaign data, and managing campaign settings. Additionally, several tutorials walk developers through common use cases, providing step-by-step instructions and explanations.
- FAQ and Troubleshooting Guide: A frequently asked questions (FAQ) section addresses common issues and questions encountered by developers. This guide contains solutions to many common problems and points developers towards the appropriate documentation or support channels for more complex issues. The FAQ is updated regularly to reflect the latest changes and user feedback.
Support Channels
Several support channels are available to help developers resolve API-related issues. Choosing the appropriate channel depends on the urgency and complexity of the problem.
- Email Support: For general inquiries and non-urgent issues, developers can contact our support team via email at [Email Address Placeholder – Replace with actual email address]. Our support team aims to respond to all emails within 24-48 hours.
- Community Forum: An active community forum ([Forum Link Placeholder – Replace with actual link]) allows developers to interact with each other, share knowledge, and ask questions. Our support team actively monitors the forum and participates in discussions, providing assistance and guidance where needed. This collaborative environment fosters knowledge sharing and accelerates problem-solving.
- Premium Support (Enterprise Customers): Enterprise customers have access to premium support, offering dedicated support engineers, priority response times, and direct communication channels for critical issues. The specifics of premium support are Artikeld in the enterprise service level agreement (SLA).
Illustrative Example: Campaign Creation Workflow
This section details a step-by-step guide to creating a new marketing campaign using the V2 API. We’ll walk through each stage, outlining the required parameters, expected responses, and potential error scenarios. Understanding this workflow is crucial for effectively leveraging the API’s capabilities. This example assumes familiarity with basic HTTP requests and JSON data structures.
Campaign Creation Request
The first step involves sending a POST request to the `/v2/campaigns` endpoint. This endpoint expects a JSON payload containing all the necessary campaign details. A successful request will return a 201 (Created) status code along with a JSON representation of the newly created campaign, including a unique identifier.
Example Request Body (JSON):
"name": "Summer Sale Campaign", "startDate": "2024-06-15", "endDate": "2024-07-15", "budget": 10000, "targetAudience": "ageRange": "min": 25, "max": 45 , "location": "United States" , "channels": ["email", "socialMedia"]
Example Successful Response (JSON):
"id": "camp-12345", "name": "Summer Sale Campaign", "startDate": "2024-06-15", "endDate": "2024-07-15", "budget": 10000, "targetAudience": "ageRange": "min": 25, "max": 45 , "location": "United States" , "channels": ["email", "socialMedia"], "status": "active"
Failure to provide required fields (like `name`, `startDate`, `endDate`) will result in a 400 (Bad Request) error with a JSON response detailing the missing fields. Similarly, providing invalid data types (e.g., a string for the `budget` field) will also trigger a 400 error.
Campaign Status Check
After creating the campaign, it’s good practice to verify its status. This can be achieved by sending a GET request to the `/v2/campaigns/campaignId` endpoint, replacing `campaignId` with the unique identifier returned in the previous step. A successful request returns a 200 (OK) status code and the campaign details. A 404 (Not Found) error indicates that the campaign with the specified ID doesn’t exist.
Example GET Request: GET /v2/campaigns/camp-12345
Example Successful Response (JSON): (Same as the successful response in the previous step, showing the campaign details)
Campaign Update (Optional)
The API allows modifying existing campaigns. This can be done by sending a PUT request to the `/v2/campaigns/campaignId` endpoint with a JSON payload containing the updated campaign details. Only the fields you want to modify need to be included in the request body. A successful update returns a 200 (OK) status code and the updated campaign details. A 404 error occurs if the campaign ID is invalid.
Example PUT Request Body (JSON):
"budget": 12000
Last Word
Mastering the Campaign REST API, whether V1 or V2, hinges on a thorough understanding of its intricacies. This guide has provided a comprehensive overview, comparing and contrasting the key features, functionalities, and potential challenges of each version. By understanding the differences in data structures, authentication methods, endpoint usage, and error handling, developers can build robust and efficient applications. Remember to consult the official documentation and leverage best practices for optimal performance and security.
Happy coding!
Essential FAQs
What are the major advantages of using V2 over V1?
V2 typically offers improved performance, enhanced security features, a more streamlined data structure, and often includes new functionalities not available in V1. It’s usually better documented and supported.
Is it possible to mix V1 and V2 endpoints in a single application?
Generally, it’s not recommended to mix V1 and V2 endpoints in a single application due to potential inconsistencies and complications in data handling and authentication. It’s best practice to choose one version and stick with it for a given project.
What happens if I exceed the API rate limits?
Exceeding rate limits typically results in temporary throttling, meaning your requests will be delayed or rejected until you fall back within the allowed limits. The API documentation should specify the handling of rate limit exceedances.
Where can I find detailed error codes and their explanations?
Detailed error codes and explanations should be available in the official API documentation for both V1 and V2. Look for sections on error handling or response codes.