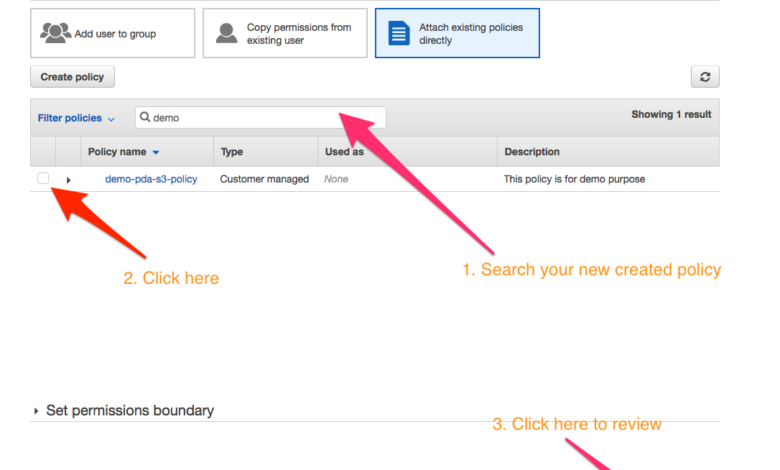
Check Your Amazon S3 Permissions Someone Will
Check your Amazon S3 permissions someone will – that’s the chilling warning echoing through the cloud computing world. Leaving your Amazon S3 buckets improperly configured is like leaving your front door unlocked – a wide-open invitation for trouble. This isn’t just about a minor inconvenience; we’re talking about potentially catastrophic data breaches, hefty fines, and irreparable damage to your reputation.
Let’s dive into why securing your S3 buckets is crucial and how to avoid becoming the next headline in a data breach story.
This post breaks down the security risks associated with misconfigured S3 permissions, offering practical steps to secure your data. We’ll cover best practices, tools to help you audit your setup, and a clear explanation of why that warning, “Check your Amazon S3 permissions someone will,” should send shivers down your spine (and spur you into action!). We’ll also tackle the nuances of Access Control Lists (ACLs) and bucket policies, helping you understand which tools are best for your specific needs.
Security Risks Associated with Improper S3 Permissions
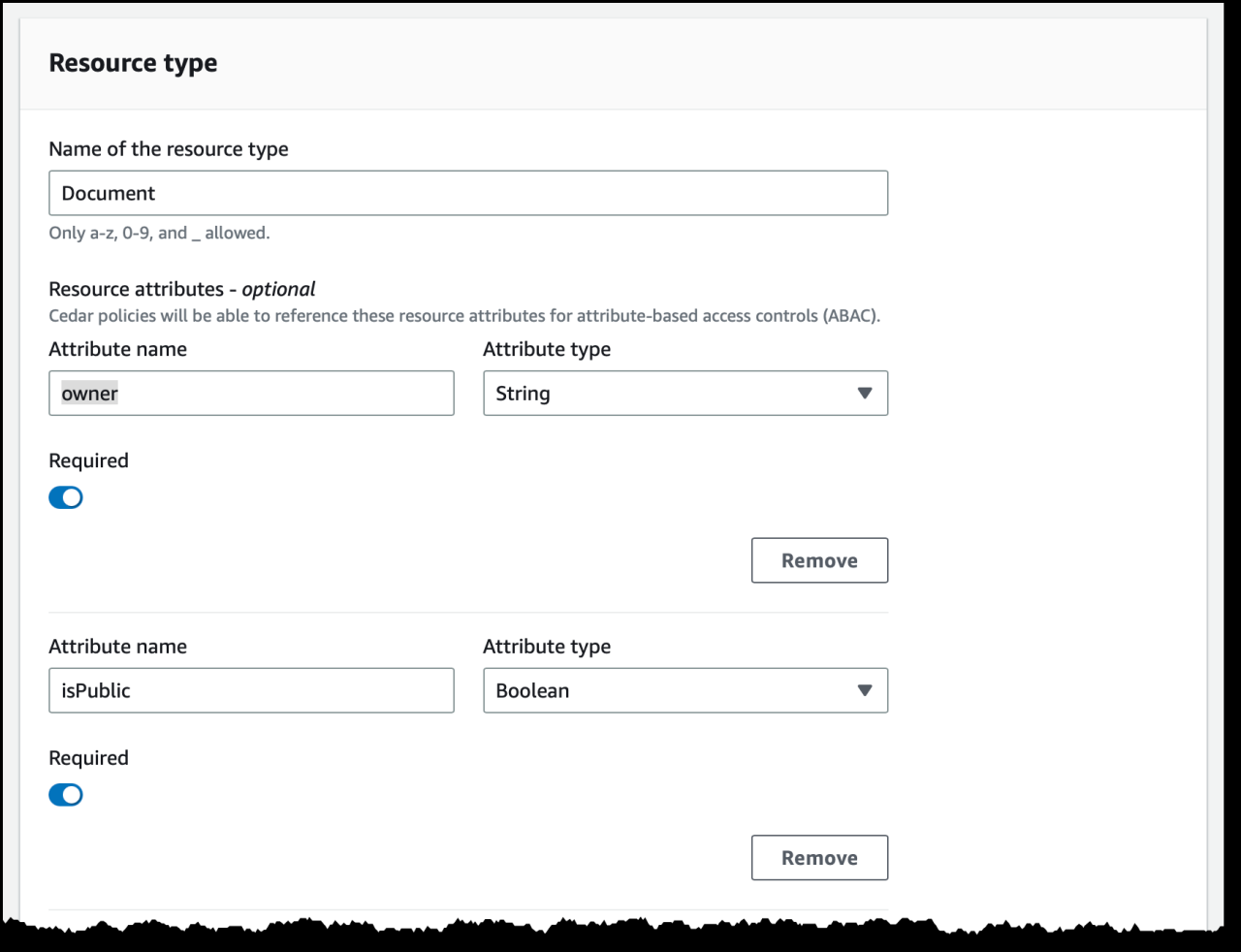
Improperly configured Amazon S3 permissions represent a significant security vulnerability, exposing organizations to data breaches and reputational damage. Understanding the risks associated with these misconfigurations is crucial for maintaining data security and compliance. This discussion will explore the common vulnerabilities, potential impacts, and real-world consequences of insecure S3 bucket configurations.
Common S3 Permission Vulnerabilities
Misconfigured Amazon S3 bucket policies are a primary source of data breaches. Common vulnerabilities stem from overly permissive access controls, allowing unauthorized individuals or applications to access sensitive data. This can range from accidentally making a bucket public to granting excessive permissions to internal users or third-party applications. The lack of proper authentication and authorization mechanisms further exacerbates these risks.
Regularly reviewing and updating bucket policies, alongside implementing the principle of least privilege, is essential to mitigate these risks.
Impact of Overly Permissive S3 Bucket Policies, Check your amazon s3 permissions someone will
Overly permissive S3 bucket policies significantly increase the attack surface. When buckets are configured with public read access, any internet user can access the data stored within. This could lead to exposure of confidential customer data, intellectual property, source code, or other sensitive information. Even seemingly minor misconfigurations, such as granting access to a specific user group without sufficient restrictions, can create vulnerabilities that attackers can exploit.
The consequences can range from financial losses and legal penalties to severe reputational damage.
Consequences of Unauthorized Access to Sensitive Data
Unauthorized access to sensitive data stored in S3 buckets can have far-reaching consequences. Data breaches can lead to significant financial losses due to regulatory fines, legal fees, and the cost of remediation. Beyond the financial impact, reputational damage can be substantial, leading to loss of customer trust and business disruption. Depending on the type of data compromised, organizations may face legal repercussions, including lawsuits and investigations from regulatory bodies.
The long-term effects of a data breach can significantly impact an organization’s ability to operate effectively.
Examples of Real-World Security Breaches Caused by S3 Permission Issues
Numerous high-profile data breaches have been attributed to misconfigured S3 permissions. The following table illustrates some notable examples:
Breach Date | Company Affected | Type of Data Breached | Root Cause |
---|---|---|---|
2017 | Verizon | Customer data, including names, addresses, and phone numbers | Misconfigured S3 bucket |
2018 | Capital One | 100 million customer records, including personal information and financial data | Misconfigured S3 bucket and web application firewall misconfiguration |
2019 | GoDaddy | Customer data, including names, addresses, and email addresses | Misconfigured S3 bucket |
2023 | Various Companies (multiple incidents) | Varies (customer data, internal documents, etc.) | Improperly configured S3 bucket permissions, lack of proper access controls |
Best Practices for Securing Amazon S3 Buckets
Securing your Amazon S3 buckets is paramount to protecting your data from unauthorized access and potential breaches. Implementing robust security measures is crucial, not just for compliance reasons, but also to maintain the integrity and confidentiality of your valuable information. This involves a multi-layered approach encompassing bucket policies, IAM roles, encryption, and regular audits. Let’s delve into some best practices.
Appropriate S3 Bucket Policies
Well-defined bucket policies are the cornerstone of S3 security. These policies dictate who can access your buckets and what actions they can perform. Instead of relying on the default settings, which often grant overly permissive access, you should craft granular policies that explicitly define allowed actions for specific users or groups. This minimizes the attack surface and ensures only authorized entities can interact with your data.
For instance, a policy might grant read-only access to a specific user group for a particular folder within a bucket, while restricting write access entirely to a select few administrators. Avoid using wildcard characters (*) excessively, as this can significantly broaden access beyond what’s intended.
Effective Use of IAM Roles and Policies
IAM (Identity and Access Management) roles and policies provide a more sophisticated way to manage access control than bucket policies alone. IAM roles allow you to grant temporary permissions to applications or services without needing to share long-term credentials. This enhances security by reducing the risk of compromised credentials. Policies associated with these roles specify exactly what actions the role can perform on your S3 buckets.
For example, a Lambda function processing images in S3 could be assigned a role that only permits read access to a specific bucket and write access to a designated output bucket. This prevents the function from accessing other sensitive data or performing unintended actions.
Least Privilege Access Control
The principle of least privilege dictates that users and applications should only be granted the minimum necessary permissions to perform their tasks. This dramatically reduces the impact of a potential security breach. If an account is compromised, the damage is limited to the specific actions permitted by its assigned permissions. Avoid granting broad permissions like full control; instead, grant only the specific read, write, or list permissions needed for each user or application.
For example, a data analyst might only need read access to a specific dataset, not write access or the ability to delete files. This fine-grained control is critical for mitigating risks.
Regular Auditing and Review of S3 Permissions
Regularly auditing and reviewing your S3 permissions is essential to identify and address potential vulnerabilities. This involves systematically examining your bucket policies, IAM roles, and access logs to ensure that permissions remain appropriate and aligned with your security posture. Regular reviews can uncover outdated permissions, misconfigurations, or unauthorized access attempts. Automated tools can help streamline this process by generating reports on access patterns and potential security risks.
A well-defined audit schedule, perhaps monthly or quarterly, depending on your sensitivity levels, is recommended.
Data Encryption at Rest and in Transit
Encrypting your data both at rest (while stored in S3) and in transit (while being transferred) adds an extra layer of security. S3 Server-Side Encryption (SSE) offers various options, including SSE-S3 (managed by AWS), SSE-KMS (using your own AWS KMS keys), and SSE-C (using your own encryption keys). Choosing the appropriate option depends on your security requirements and compliance needs.
For data in transit, using HTTPS ensures that your data is encrypted during communication between your application and S3. These encryption methods provide protection even if a breach occurs, making it significantly harder for attackers to access your sensitive information.
Identifying and Addressing Vulnerable S3 Permissions
So, you’ve secured your S3 buckets (or at least, youthink* you have). But how confident are you? Even with best practices in place, overlooked permissions or poorly configured policies can leave your data vulnerable. This section dives into the practical steps of identifying and rectifying these vulnerabilities, turning proactive security from a theory into a tangible reality.Identifying S3 buckets with overly permissive access controls requires a multi-pronged approach.
It’s not enough to simply hope for the best; a systematic review is crucial. This involves a combination of automated tools, manual checks, and a keen eye for detail. Failure to properly secure your S3 buckets can lead to data breaches, hefty fines, and reputational damage – consequences far outweighing the effort required for diligent security audits.
Techniques for Identifying Overly Permissive Access Controls
Several techniques can help pinpoint S3 buckets with lax security. One effective method involves leveraging AWS’s own security tools, such as the IAM Access Analyzer. This service identifies resources that are publicly accessible and provides detailed reports on permissions granted to users and roles. Another strategy involves using automated security scanning tools (discussed further below) which can proactively identify misconfigurations and vulnerabilities within your S3 infrastructure.
Finally, regular manual reviews of bucket policies and access control lists (ACLs) are essential, as automated tools might not catch every nuance. Remember, a layered approach is always the most robust.
Step-by-Step Procedure for Reviewing Existing S3 Bucket Policies
Let’s walk through a systematic review of an existing S3 bucket policy.
1. Access the Bucket Policy
Navigate to your S3 bucket in the AWS Management Console. Select the bucket and then choose the “Permissions” tab. You’ll find the bucket policy here. If no policy exists, that’s a good starting point – it means everyone has potentially very open access.
2. Analyze the Policy Statement
The policy is written in JSON. Carefully examine each statement, paying close attention to the `Action`, `Resource`, `Principal`, and `Condition` elements. The `Action` specifies the permitted operations (e.g., `s3:GetObject`, `s3:PutObject`). The `Resource` defines the specific S3 objects or buckets affected. The `Principal` identifies who has these permissions (e.g., specific users, groups, or AWS accounts).
`Condition` elements add further restrictions, based on factors like IP address or time.
3. Identify Overly Permissive Actions
Look for actions that grant broader access than necessary. For example, `s3:*` grants full control – a huge security risk unless absolutely justified. Similarly, be wary of `Principal` elements that are too broad, such as `*` (everyone) or `AWS` (all AWS accounts).
4. Verify Resource Specificity
Ensure that the `Resource` element is specific enough. Avoid using wildcards like `arn:aws:s3:::*` unless absolutely required.
5. Review Conditions
Analyze any conditions applied to the policy. Are they sufficient to mitigate potential risks?
6. Update the Policy
Based on your review, modify the policy to restrict access to only what’s absolutely necessary. Remember the principle of least privilege – grant only the minimum permissions required for a given task.
Checklist for Ensuring Secure S3 Bucket Configurations
Before deploying any S3 bucket, use this checklist to ensure its security:
A robust security posture is paramount. This checklist is designed to guide you through crucial configurations to ensure your data remains protected.
- Enable Server-Side Encryption (SSE): Protect data at rest using either AWS-managed keys (SSE-S3 or SSE-KMS) or your own customer-managed keys (SSE-C).
- Restrict Public Access: Explicitly deny public access to your bucket and objects. Use bucket policies and ACLs to control access meticulously.
- Use Versioning: Enable versioning to protect against accidental data deletion or corruption.
- Implement Access Control Lists (ACLs): Use ACLs to manage access at the object level, complementing bucket policies. However, bucket policies are generally preferred for better control and management.
- Regularly Audit and Review Policies: Establish a schedule for reviewing your S3 bucket policies and configurations to identify and address potential vulnerabilities.
- Enable MFA Delete: Require multi-factor authentication (MFA) for deleting objects to prevent accidental or malicious deletion.
- Monitor Access Logs: Enable S3 access logging to track all access requests to your buckets, facilitating security analysis and incident response.
Tools and Services for S3 Security Auditing
Several tools and services assist in S3 security auditing:
Automated tools are essential for efficient and comprehensive S3 security audits. These tools provide automated scanning capabilities, enabling early detection and remediation of potential vulnerabilities.
- AWS IAM Access Analyzer: Identifies publicly accessible resources.
- AWS Config: Monitors and assesses the configurations of your AWS resources, including S3 buckets, for compliance with predefined rules.
- CloudTrail: Records AWS API calls, providing a comprehensive audit trail of all activity within your AWS environment, including actions performed on S3.
- Third-party security scanning tools: Many third-party tools offer specialized S3 security assessments, providing in-depth analysis and reporting.
The Role of Access Control Lists (ACLs) in S3 Security
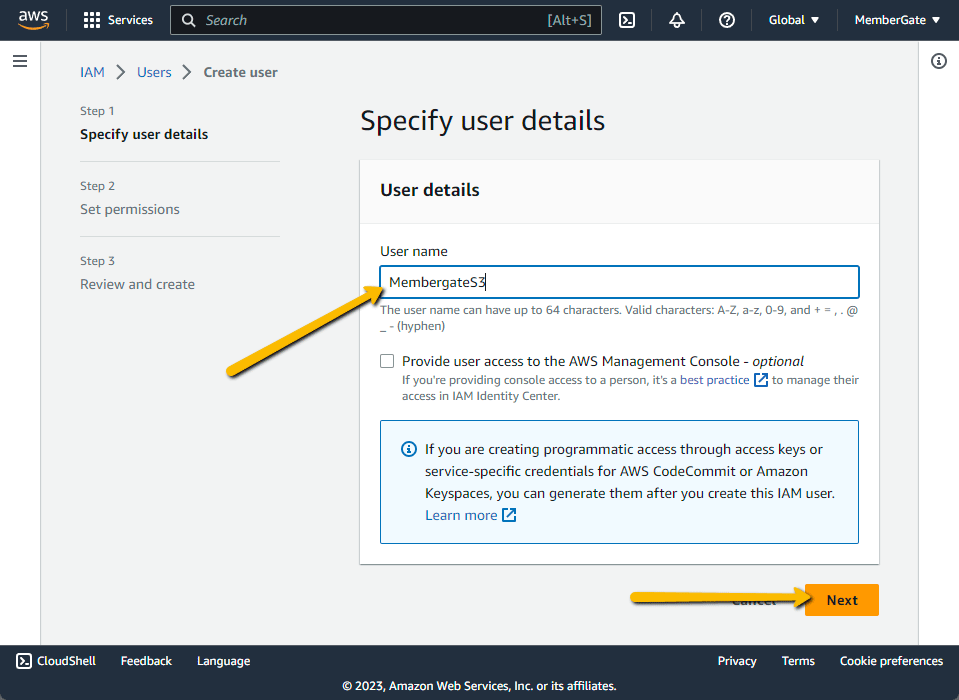
Amazon S3 offers multiple ways to control access to your buckets and objects, and understanding the nuances of each is crucial for robust security. Access Control Lists (ACLs) are one such mechanism, but their role and effectiveness are often misunderstood. This post delves into the specifics of ACLs, comparing them to other methods and highlighting their strengths and weaknesses.ACLs, at their core, grant permissions to individual AWS accounts or groups of accounts.
They work by associating specific access rights (read, write, or full control) with individual identities, allowing granular control—in theory. However, this granular control comes with significant limitations when compared to more modern access control methods.
ACLs versus Bucket Policies
ACLs and bucket policies both manage access, but they differ significantly in their scope and functionality. ACLs control access to individual objects or the bucket itself, while bucket policies control access at the bucket level, offering broader control over actions performed on the objects within. A bucket policy can be far more comprehensive, enabling conditions and fine-grained rules based on various factors like IP address or request origin.
In contrast, ACLs are simpler to understand and implement but lack the flexibility and scalability of bucket policies. Consider a scenario where you need to restrict access based on the source IP address; ACLs cannot handle this, while a bucket policy can effortlessly achieve this.
Limitations of ACLs
The primary limitation of ACLs is their lack of scalability and complexity management. As the number of users and objects grows, managing individual ACLs becomes increasingly cumbersome and error-prone. Furthermore, ACLs cannot enforce conditions; they grant permissions based solely on identity. This lack of conditional access control makes them unsuitable for scenarios requiring sophisticated access management. Another significant drawback is the difficulty in auditing and tracking access changes.
Tracing changes made using ACLs is much more challenging compared to auditing changes in a centrally managed bucket policy. Finally, ACLs can lead to inconsistencies if not meticulously managed, potentially creating security vulnerabilities.
Appropriate and Inappropriate Use Cases for ACLs
ACLs can be appropriate for simple use cases involving a small number of users and objects. For instance, sharing a single object with a limited number of known individuals might be effectively managed with ACLs. However, for any complex environment, particularly those involving a large number of users, objects, or needing conditional access, ACLs should be avoided. Using ACLs in complex scenarios can lead to misconfigurations, inconsistencies, and ultimately, security breaches.
Seriously, check your Amazon S3 permissions; someone will try to exploit a misconfiguration. Proper cloud security is paramount, and understanding tools like those discussed in this excellent article on bitglass and the rise of cloud security posture management is key. Ignoring this could lead to a major headache, so make sure your S3 buckets are locked down tight!
The complexity and limitations of ACLs make them unsuitable for production environments with dynamic access requirements.
Advantages and Disadvantages of ACLs
Before implementing ACLs, carefully consider the following:
- Advantages:
- Simple to understand and implement for basic scenarios.
- Provides granular control at the object level (though limited).
- Disadvantages:
- Difficult to manage and scale for large numbers of users and objects.
- Lack of conditional access control.
- Difficult to audit and track changes.
- Can lead to inconsistencies and security vulnerabilities if not meticulously managed.
- Less flexible and powerful than bucket policies.
Practical Steps to Remediate Insecure S3 Permissions
So, you’ve audited your Amazon S3 buckets and found some permissions that are, shall we say, less than ideal. Don’t panic! Fixing insecure S3 permissions is a manageable process, and taking proactive steps now can save you significant headaches (and potential data breaches) down the line. This guide Artikels a practical, step-by-step approach to tightening your S3 security.This section details the process of identifying and fixing insecure S3 permissions, focusing on concrete actions you can take to improve your security posture.
We’ll cover revoking unnecessary access, configuring bucket policies effectively, and implementing multi-factor authentication (MFA).
Revoking Unnecessary Access Rights
Identifying and removing unnecessary access is crucial. Start by reviewing your bucket policies and Access Control Lists (ACLs). Look for any users, groups, or accounts that have broader permissions than they actually require. For example, if a user only needs to read data, ensure they don’t have write or delete access. The principle of least privilege should always guide your decisions.
Revoking excess permissions reduces your attack surface and minimizes the potential damage from a compromised account. Use the AWS Management Console or the AWS CLI to remove unnecessary permissions. Remember to thoroughly test after making changes to ensure functionality remains intact.
Seriously, check your Amazon S3 permissions; someone will inevitably try to exploit a weakness. Building secure apps is crucial, and that’s why I’ve been diving into the world of Domino app development – learning about the exciting possibilities explored in this article on domino app dev the low code and pro code future has really helped me understand the importance of robust security from the ground up.
Getting those S3 permissions right is just one piece of the puzzle, but a vital one for any application, no matter how it’s built.
Configuring S3 Bucket Policies for Different Use Cases
Bucket policies are JSON documents that define access control at the bucket level. Properly configuring these policies is paramount. Here are examples for common use cases:
Public Website
For a public website, you’ll need to grant public read access to specific objects. This doesn’t mean making the entire bucket public; instead, use a policy that allows public read access only to the objects you intend to be publicly accessible. For example:
“Version”: “2012-10-17”, “Statement”: [ “Sid”: “PublicReadGetObject”, “Effect”: “Allow”, “Principal”: “*”, “Action”: “s3:GetObject”, “Resource”: “arn:aws:s3:::your-bucket-name/*” ]
This policy allows anyone (`Principal: “*” `) to read (`Action: s3:GetObject`) objects within your bucket. Replace `”your-bucket-name”` with your actual bucket name. Remember, this only grants read access; any other actions (writing, deleting) require explicit permissions.
Private Data Storage
For private data storage, you should restrict access to only authorized users or groups. A typical policy would look like this:
“Version”: “2012-10-17”, “Statement”: [ “Sid”: “AllowSpecificAccess”, “Effect”: “Allow”, “Principal”: “AWS”: “arn:aws:iam::your-account-id:user/your-user” , “Action”: [“s3:GetObject”, “s3:PutObject”, “s3:DeleteObject”], “Resource”: “arn:aws:s3:::your-bucket-name/*” ]
This policy grants specific actions (`Action`) to a specific IAM user (`Principal`). Replace `”your-account-id”` and `”your-user”` with your actual account ID and IAM user ARN. This ensures only the specified user can access the bucket.
Implementing Multi-Factor Authentication (MFA)
MFA adds an extra layer of security by requiring a second factor of authentication, such as a code from an authenticator app, in addition to your password. Enabling MFA for all users with access to your S3 buckets significantly reduces the risk of unauthorized access, even if credentials are compromised. This should be a mandatory security measure for all users with administrative privileges.
You can enable MFA through the AWS IAM console. It’s a simple yet highly effective step that significantly strengthens your overall security posture.
Understanding the Warning “Check Your Amazon S3 Permissions Someone Will”
That ominous warning, “Check your Amazon S3 permissions; someone will,” isn’t just a scare tactic. It’s a stark reminder of the potentially devastating consequences of misconfigured access controls on your Amazon S3 buckets. This warning signals a critical vulnerability, leaving your sensitive data exposed to unauthorized access and potentially significant financial and reputational damage.This warning highlights the fact that your S3 bucket’s permissions are too open, allowing anyone – from opportunistic hackers to malicious actors – to access your data.
The implications are far-reaching, extending beyond simple data breaches to encompass legal liabilities, financial losses, and damage to your organization’s credibility. Ignoring this warning is akin to leaving your front door unlocked – it’s an invitation for trouble.
Potential Consequences of Ignoring the Warning
The consequences of ignoring the “Check your Amazon S3 permissions” warning can be severe and far-reaching. A data breach resulting from insecure S3 permissions could lead to the exposure of confidential customer information, intellectual property, financial records, or other sensitive data. This exposure could result in significant financial penalties due to regulatory non-compliance (like GDPR or CCPA violations), legal action from affected individuals or businesses, and reputational damage that could harm your brand and customer trust.
In some cases, a breach could even lead to operational disruption if critical systems or data are compromised. For example, a company might face significant downtime and repair costs if its production databases are leaked and corrupted.
Examples of Actors Who Might Exploit Insecure S3 Permissions
Several types of individuals or entities might exploit insecure S3 permissions. These range from casual script kiddies looking for easy targets to highly organized cybercriminal groups seeking valuable data. Consider these examples:* Opportunistic Hackers: These individuals often use automated tools to scan the internet for misconfigured S3 buckets, looking for easy access to data they can exploit or sell.
Competitors
A competitor might access sensitive business information, such as marketing strategies or product development plans, to gain a competitive advantage.
Malicious Insiders
Employees with malicious intent could exploit weak permissions to steal data or cause damage to the organization.
Organized Crime Groups
These groups often target sensitive data for financial gain, such as credit card numbers, personal identifiable information (PII), or intellectual property.
Nation-State Actors
In some cases, nation-state actors might target sensitive government or corporate data for espionage or sabotage.
Illustrative Representation of Damage from Insecure S3 Permissions
Imagine a visual representation: a brightly lit, unlocked treasure chest (your S3 bucket) overflowing with gold coins (your sensitive data) sitting in the middle of a busy marketplace (the internet). Anyone can walk by, easily open the chest, and take whatever they want. This represents the vulnerability created by insecure S3 permissions. The loss of the gold coins represents the various types of damage – financial losses from data breaches, reputational damage from compromised customer trust, and the costs associated with remediation and legal battles.
The bustling marketplace symbolizes the ease with which malicious actors can find and exploit these vulnerabilities. The lack of security measures, like a lock on the chest (proper S3 permissions), is the root cause of the problem.
Ultimate Conclusion
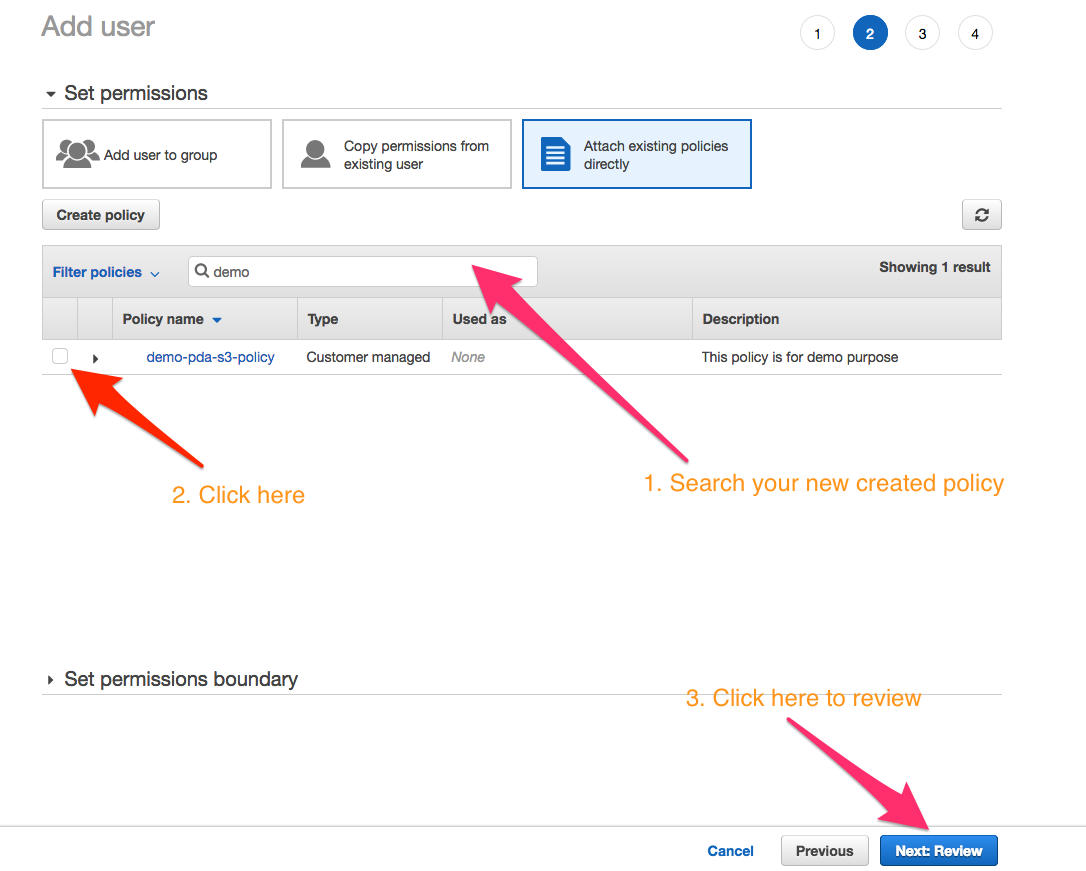
Ultimately, securing your Amazon S3 buckets isn’t just a technical task; it’s a fundamental responsibility. Ignoring the warning “Check your Amazon S3 permissions someone will” can have devastating consequences. By implementing the best practices Artikeld here – from setting appropriate bucket policies and using IAM roles effectively to regularly auditing your permissions and encrypting your data – you can significantly reduce your risk of a costly and damaging breach.
Remember, proactive security is always cheaper (and less stressful!) than reactive damage control.
FAQ
What happens if someone accesses my S3 bucket without permission?
The consequences can range from data theft and financial loss to reputational damage and legal repercussions, depending on the sensitivity of the data compromised.
How often should I review my S3 bucket permissions?
Regularly auditing your S3 permissions is vital. Aim for at least a quarterly review, but more frequent checks are recommended if you make frequent changes to your bucket configurations or access controls.
Are ACLs completely obsolete?
While bucket policies are generally preferred for their superior flexibility and management, ACLs still have some limited use cases, primarily for granting granular permissions within a single bucket. However, they should be used cautiously and only when absolutely necessary.
What are some free tools to help me audit my S3 permissions?
AWS provides several free tools within the AWS Management Console that allow you to review your bucket policies and IAM configurations. There are also several open-source and third-party tools available, though some may require paid subscriptions for advanced features.