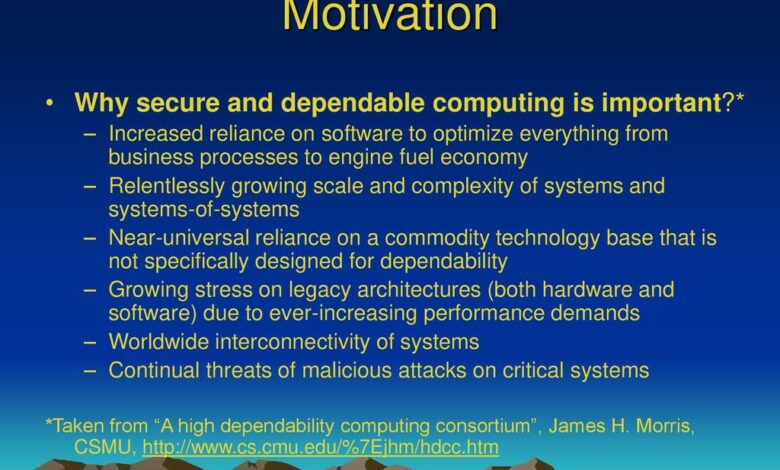
Unveiling the Pitfalls of Relying Solely on Mach Composable Solutions
Unveiling the pitfalls of relying solely on mach composable solutions isn’t just about highlighting the downsides; it’s about building a realistic understanding of their potential. While the promise of modularity and flexibility is incredibly enticing, a deep dive reveals some significant challenges. From the hidden costs of complex integrations and the increased security surface area to the potential for vendor lock-in and the skills gap needed to manage these systems effectively, the reality can be far more intricate than the initial allure.
This post will explore these critical areas, offering insights and practical advice to help you navigate the world of mach composable architectures.
We’ll examine real-world examples of projects that have stumbled, highlighting the common pitfalls that often lead to unexpected expenses, performance bottlenecks, and maintenance headaches. We’ll also discuss strategies for mitigating these risks, including best practices for security, efficient integration techniques, and planning for the long-term maintenance and scalability of your system. By the end, you’ll have a much clearer picture of whether a purely mach composable approach is the right fit for your needs.
Vendor Lock-in and Integration Challenges: Unveiling The Pitfalls Of Relying Solely On Mach Composable Solutions
The allure of MACH architecture, with its promise of flexibility and agility, is undeniable. However, a solely MACH-based approach can introduce significant challenges, particularly concerning vendor lock-in and the complexities of integrating disparate components. While the modularity offers benefits, it also presents a new set of hurdles that need careful consideration before fully embracing this approach. Ignoring these potential pitfalls can lead to unforeseen costs and delays in the long run.
One major concern is vendor lock-in. Choosing best-of-breed solutions from different vendors, while seemingly offering optimal functionality, can create a dependency on specific providers. Migrating away from a particular vendor becomes significantly more difficult and expensive if their APIs change, their company is acquired, or they discontinue support for a crucial component. This can leave your business vulnerable and significantly impact your ability to adapt to evolving market needs or technological advancements.
Integration Complexities of MACH Components
Integrating various MACH components from different vendors is rarely a seamless process. Each vendor has its own unique APIs, data formats, and integration protocols. This necessitates extensive custom development, potentially requiring specialized skills and resources that may not be readily available in-house. The process often involves intricate mapping of data between different systems, meticulous testing to ensure compatibility, and ongoing maintenance to address any integration issues that arise.
The lack of standardized interfaces and the potential for conflicting functionalities further complicate matters.
Examples of Integration Failures and Costs
Imagine a scenario where an e-commerce company uses a MACH architecture. They choose a headless CMS from Vendor A, a commerce platform from Vendor B, and a personalization engine from Vendor C. During the initial integration, unforeseen compatibility issues between the CMS and the commerce platform cause significant delays in the launch of a new product line. The cost of resolving these issues, including developer time, testing, and potential loss of revenue due to delayed launch, could run into tens of thousands of dollars.
Furthermore, ongoing maintenance and updates require constant attention, adding to the overall cost of ownership. Another example might involve a failure in data synchronization between the personalization engine and the commerce platform, leading to inaccurate product recommendations and impacting customer experience and sales.
Comparison of Integration Complexities
Feature | Monolithic Architecture | MACH Architecture |
---|---|---|
Initial Integration | Relatively simpler, often within a single vendor’s ecosystem | Complex, requiring integration of multiple vendors’ APIs and systems |
Ongoing Maintenance | Usually handled by a single vendor | Requires coordination and maintenance across multiple vendors |
Vendor Lock-in | High, tied to a single vendor | Potentially high, depending on vendor choices and API dependencies |
Scalability | Can be challenging to scale specific components independently | Easier to scale individual components independently |
Security Risks and Data Management
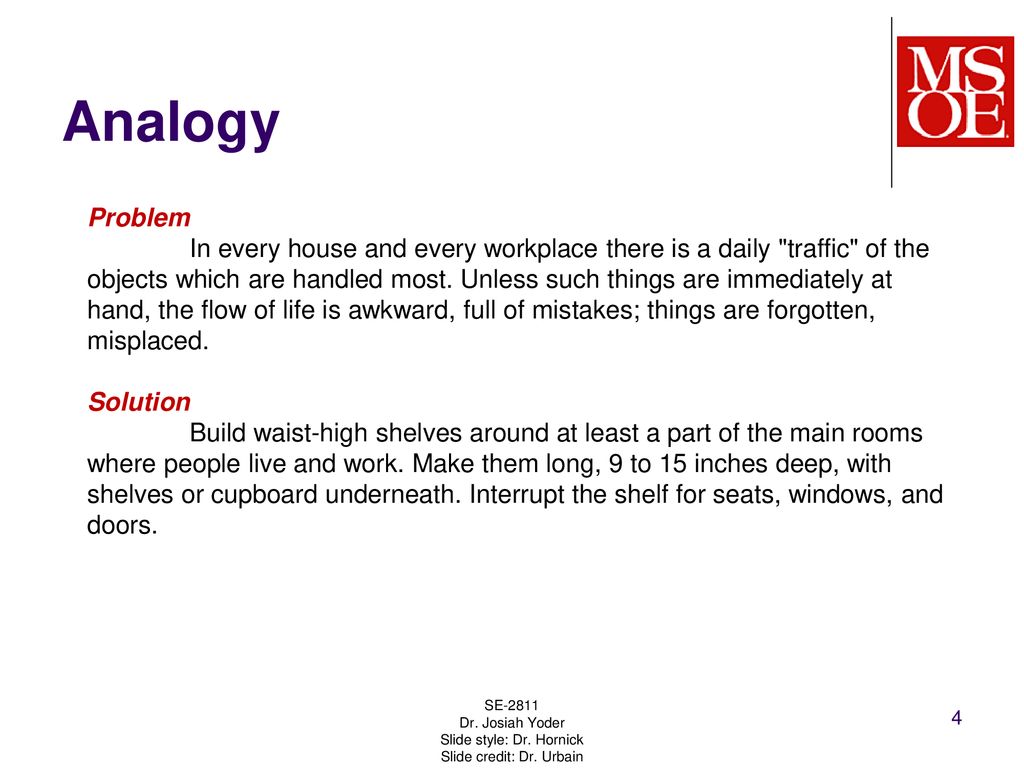
The beauty of composable architectures lies in their flexibility, but this flexibility comes at a cost. The very nature of connecting numerous independent components creates a significantly larger attack surface than a monolithic system. Data, now flowing across multiple systems and potentially residing in various locations, becomes more vulnerable, demanding a robust and comprehensive security strategy. Failing to adequately address these concerns can lead to significant breaches and reputational damage.The increased complexity inherent in mach composable architectures presents several unique security challenges.
Each microservice, API, and third-party integration point represents a potential entry point for malicious actors. A single vulnerability in one component can compromise the entire system, highlighting the importance of a holistic security approach. Furthermore, the dynamic nature of these architectures, with components constantly being added, removed, or updated, makes maintaining consistent security posture a continuous challenge.
Increased Security Surface Area and Vulnerabilities
The interconnected nature of mach composable architectures dramatically expands the attack surface. Each component, including APIs, databases, and third-party services, introduces new potential vulnerabilities. For instance, a poorly secured API could allow unauthorized access to sensitive data, while a vulnerable database could lead to a data breach. Mitigation strategies include rigorous security testing of each component, implementing robust authentication and authorization mechanisms, and utilizing strong encryption protocols for data in transit and at rest.
Regular security audits and penetration testing are crucial for identifying and addressing vulnerabilities before they can be exploited. Employing a zero-trust security model, which assumes no implicit trust, is also a vital consideration.
Data Consistency and Security Across Disparate Systems
Maintaining data consistency and security across multiple systems is a significant challenge. Data may be replicated or distributed across different databases or storage solutions, making it difficult to ensure data integrity and confidentiality. Inconsistencies can lead to inaccurate reporting, flawed decision-making, and regulatory non-compliance. Implementing strong data governance policies, including data encryption, access control, and data loss prevention (DLP) measures, is crucial.
Employing a centralized data management platform can help streamline data governance and improve visibility into data flows. Furthermore, leveraging technologies like blockchain for immutable data logging can enhance data integrity and traceability.
Best Practices for Securing a Mach Composable Architecture, Unveiling the pitfalls of relying solely on mach composable solutions
Addressing the security concerns of mach composable architectures requires a proactive and multi-layered approach. Here are some best practices to consider:
- Implement robust authentication and authorization mechanisms at each integration point.
- Utilize strong encryption for data in transit and at rest.
- Conduct regular security audits and penetration testing to identify vulnerabilities.
- Employ a zero-trust security model, verifying every access request.
- Implement a centralized logging and monitoring system to detect and respond to security incidents.
- Regularly update and patch all components to address known vulnerabilities.
- Implement robust data governance policies, including data encryption, access control, and DLP measures.
- Leverage technologies like blockchain for enhanced data integrity and traceability.
- Employ a comprehensive incident response plan to effectively handle security breaches.
- Invest in security awareness training for all personnel.
Cost and Complexity of Maintenance
The allure of a microservices architecture, often touted as the backbone of “mach composable” solutions, can quickly fade when confronted with the realities of long-term maintenance. While the initial setup might seem modular and efficient, the cumulative costs and complexities can significantly outweigh the benefits if not carefully considered. This isn’t about dismissing the potential of composable systems entirely, but rather a realistic appraisal of the hidden expenses and operational challenges.The long-term cost of maintaining a mach composable system often surpasses that of a monolithic system, particularly as the number of components grows.
This is due to the increased surface area for potential issues, the need for specialized expertise across multiple technologies, and the intricate dependencies between different services. A monolithic system, while less flexible, often benefits from a simpler, more unified maintenance process.
Unexpected Maintenance Costs in Mach Composable Solutions
Unexpected expenses frequently arise from the distributed nature of mach composable architectures. For instance, a seemingly minor bug in one microservice can trigger a cascade of failures across interconnected services, requiring extensive debugging and remediation efforts. This can lead to unplanned downtime, lost productivity, and significant costs associated with emergency fixes and incident response. Consider a scenario where a payment gateway service experiences an outage, impacting the entire e-commerce platform built upon it.
The cost of resolving the issue, including developer time, potential financial losses due to downtime, and customer support, can be substantial. Another example involves third-party API dependencies. Changes in a third-party service’s API, even minor ones, can necessitate extensive code modifications across multiple microservices, leading to unexpected maintenance burdens.
Cost Breakdown of Maintaining a Mach Composable System
Let’s Artikel the various cost components involved:
A comprehensive cost breakdown should include:
- Development and Maintenance Personnel: The need for specialized expertise in various technologies increases the overall personnel costs. You’ll need developers skilled in different programming languages, cloud platforms, and API management tools. This is a significant ongoing expense.
- Infrastructure Costs: Managing numerous microservices requires a distributed infrastructure, often relying on cloud services. This incurs ongoing costs for compute, storage, networking, and monitoring tools.
- Monitoring and Logging: Effective monitoring and logging are crucial for identifying and resolving issues in a complex, distributed system. Investing in robust monitoring and logging solutions adds to the overall maintenance cost.
- Testing and Deployment: The complexity of deploying updates and patches across multiple components increases the time and resources needed for testing and deployment. This leads to increased costs associated with testing infrastructure, automation tools, and developer time.
- Security and Compliance: Securing a distributed system is inherently more challenging than securing a monolithic application. This necessitates investment in security tools, penetration testing, and compliance audits, which add to the overall maintenance cost.
Complexity of Updates and Patches
The complexity of updates and patches increases exponentially with the number of components in a mach composable system. A single bug fix might require updating dozens of individual microservices, each with its own deployment process and potential dependencies. This increases the risk of introducing new bugs during the update process and significantly extends the time required for maintenance.
Furthermore, coordinating updates across multiple teams and ensuring backward compatibility adds another layer of complexity. Imagine updating a core library used by fifty different microservices; the coordination effort and testing required are considerable. This complexity translates directly into increased maintenance costs and potential downtime.
Performance and Scalability Issues
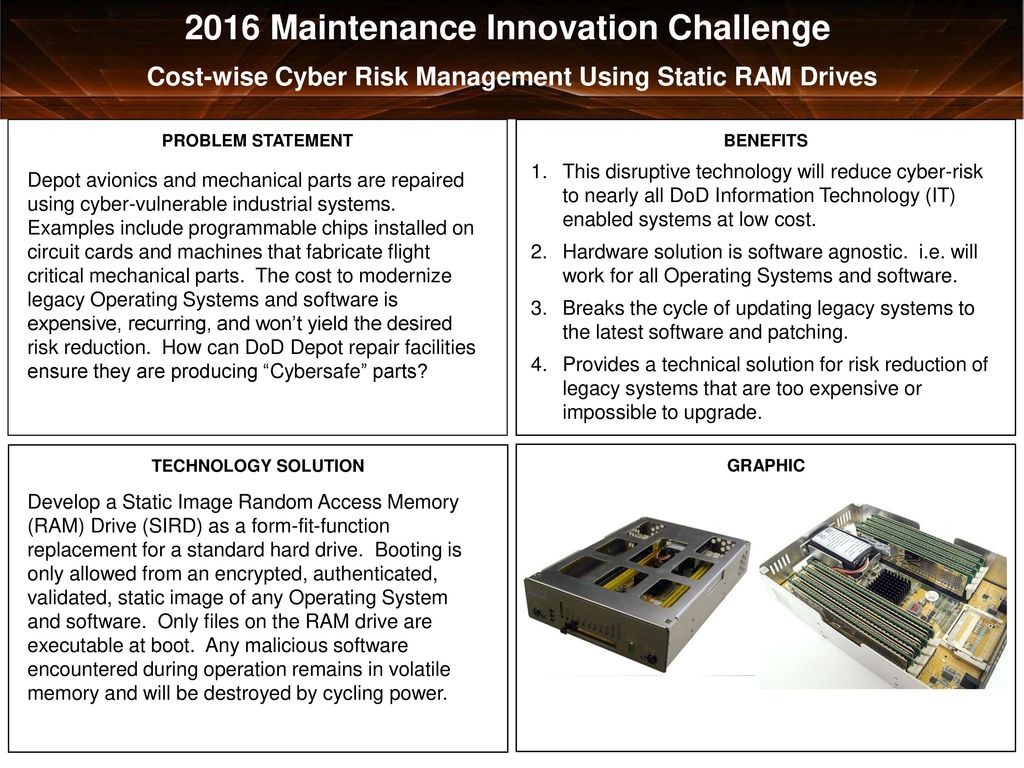
The allure of a modular, composable architecture often overshadows potential performance pitfalls. While the flexibility of Mach composable solutions is undeniable, the very nature of integrating numerous independent components can introduce unexpected bottlenecks and limit scalability in ways that are not immediately apparent during the initial design phase. Understanding these potential issues is crucial for building robust and performant applications.The inherent complexity of a Mach composable architecture, where multiple services interact over networks, can lead to performance degradation.
Each component, regardless of its individual efficiency, contributes to the overall latency. Network communication overhead, serialization/deserialization of data, and the time spent in context switching between different services all add up. Furthermore, the scalability of the entire system is constrained by the least scalable component; a single slow or poorly designed microservice can bring the whole system to its knees.
Performance Bottlenecks in Component Integration
Integrating multiple components inevitably introduces points of potential failure. Network latency between components, for example, becomes a significant factor. If components are geographically dispersed or rely on slow network connections, the overall response time of the application can suffer significantly. Database interactions, especially if multiple components access the same database, can also create bottlenecks. Inefficient data transfer between services, such as using overly verbose data formats or inefficient communication protocols, further exacerbate these issues.
A poorly designed API gateway can also introduce significant latency and become a single point of failure. Consider a scenario where a customer relationship management (CRM) system needs to interact with an inventory management system and a payment gateway. If the network connection between these systems is slow or unreliable, the entire order processing workflow will be hampered.
Scalability Limitations Due to Component Constraints
The scalability of a Mach composable solution is not simply the sum of the scalability of its individual components. The interactions between components introduce dependencies that can limit the overall scalability. For instance, a single component with a limited throughput capacity can become a bottleneck, preventing the entire system from scaling beyond a certain point. Consider a scenario where a high-traffic e-commerce website uses a Mach composable architecture.
If the payment gateway component cannot handle a high volume of transactions, the entire website will become unresponsive during peak hours, even if other components are highly scalable. Similarly, if the database used by multiple components lacks sufficient capacity, it will become a major bottleneck hindering the overall scalability.
Real-World Examples of Performance Issues
While specific details of internal performance issues are often kept confidential, numerous case studies demonstrate the challenges in scaling microservices architectures. One common problem is the “distributed tracing” problem – identifying the source of performance bottlenecks across numerous services. Debugging and optimizing such systems often requires specialized tooling and expertise. Many organizations have reported difficulties in scaling their microservices architectures to handle sudden spikes in traffic, leading to degraded performance or complete outages.
In some cases, poorly designed inter-service communication protocols have caused significant latency and reduced throughput. These examples highlight the importance of careful planning, rigorous testing, and robust monitoring to mitigate performance issues in Mach composable deployments.
Component Interaction and Performance Impact
The following flowchart illustrates how different components interact and potentially impact overall system performance.[Imagine a flowchart here: The flowchart would depict several boxes representing different components (e.g., API Gateway, User Interface, CRM, Inventory, Payment Gateway, Database). Arrows would show data flow between these components. Bottlenecks would be highlighted by thicker arrows or different colors indicating slow processing or high latency.
For example, a thick arrow from the API Gateway to the Database would represent a potential bottleneck due to database overload.]
Lack of Standardization and Interoperability
The promise of Mach composable architectures hinges on the ability of different components, from various vendors, to work seamlessly together. However, the current landscape is far from this ideal. A significant hurdle is the lack of widely adopted standards, leading to significant interoperability challenges and hindering the true potential of this innovative approach.The difficulties stem from the diverse approaches taken by different vendors in designing and implementing their Mach composable solutions.
This lack of standardization manifests in various ways, from incompatible APIs and data formats to differing security protocols and deployment models. Consequently, integrating components from different vendors often becomes a complex and time-consuming process, requiring extensive custom development and potentially compromising the intended agility and efficiency of the architecture.
API and Data Format Incompatibilities
Different vendors often employ unique APIs and data formats for their components. This means that connecting two components from different vendors may require significant custom coding to translate data between incompatible formats. For example, one vendor might use JSON while another uses XML, necessitating the creation of custom translation layers. This adds to development costs and increases the risk of errors and inconsistencies.
The absence of a common, standardized API drastically increases integration complexity and significantly slows down deployment.
Security Protocol Discrepancies
Security is paramount in any system, and the Mach composable space is no exception. However, a lack of standardization in security protocols makes it challenging to ensure consistent security across a system built from multiple vendor components. Each vendor may have its own authentication and authorization mechanisms, leading to security gaps and potential vulnerabilities if these mechanisms are not carefully integrated and managed.
A lack of a standardized approach increases the risk of breaches and complicates the task of maintaining a secure and reliable system.
Deployment Model Differences
Further compounding the interoperability challenges are differences in deployment models. Some vendors may offer cloud-native solutions, while others might focus on on-premises deployments. Integrating these diverse deployment models can be complex, requiring careful consideration of network configurations, security policies, and data transfer mechanisms. This lack of standardization in deployment strategies makes it difficult to achieve a consistent and efficient deployment process across the entire system.
Illustrative Representation of Interoperability Challenges
Imagine a complex system built using components from three different vendors (Vendor A, Vendor B, and Vendor C). Each vendor’s components have unique APIs and data formats. To connect Vendor A’s component to Vendor B’s, a custom integration layer (Layer AB) needs to be developed. Similarly, another custom integration layer (Layer BC) is needed to connect Vendor B’s component to Vendor C’s.
This creates a “chain” of integration layers, each adding complexity and potential points of failure. The more vendors involved, the longer and more complex this chain becomes, exponentially increasing the integration effort and the risk of errors. This scenario highlights the significant challenges posed by the lack of standardization in the Mach composable ecosystem.
Skills Gap and Expertise Requirements
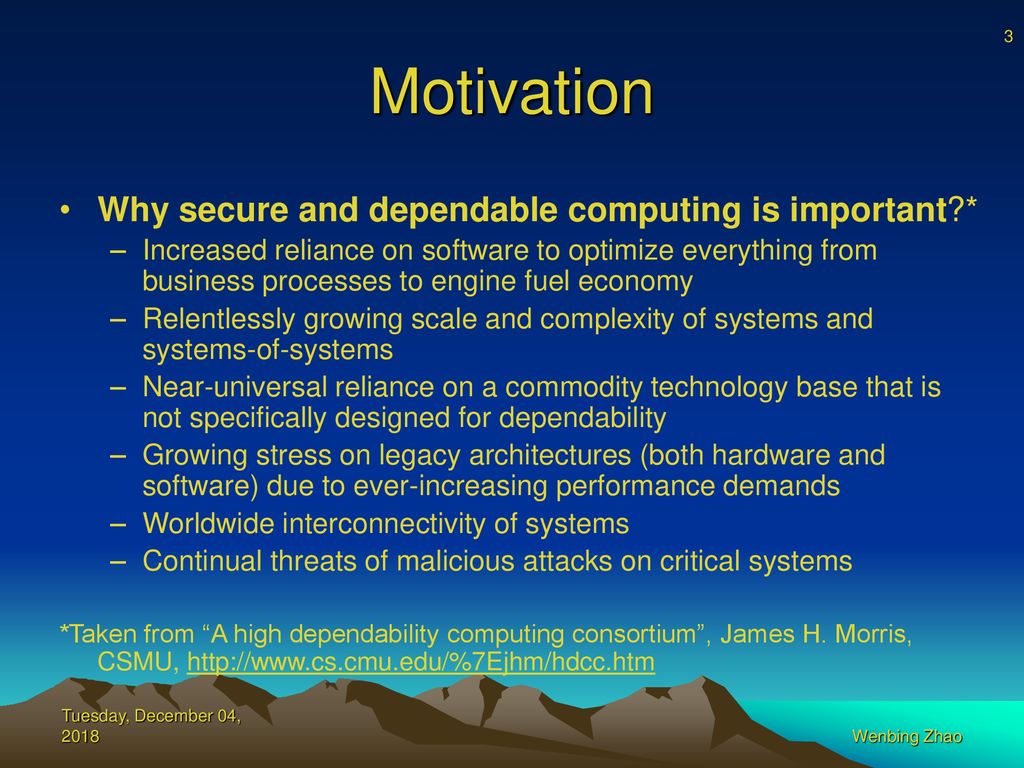
The shift towards MACH (Microservices-based, API-first, Cloud-native, Headless) architectures presents a significant challenge: a widening skills gap. Building and maintaining these complex systems requires a unique blend of expertise that’s currently in short supply, creating hurdles for organizations looking to embrace this approach. Finding and retaining talent with the necessary skills is becoming a critical factor in determining the success or failure of MACH adoption.The complexity of MACH architectures necessitates a diverse and highly skilled team.
It’s not simply about assembling a group of developers; it demands specialists capable of navigating the intricacies of microservices, API management, cloud infrastructure, and headless CMS systems. This multi-faceted requirement makes recruitment a significant undertaking.
Specialized Skills Needed for MACH Composable Systems
The successful implementation of a MACH architecture relies on a team possessing a diverse skillset spanning several key areas. These skills aren’t easily found in a single individual, necessitating collaborative teamwork and potentially specialized roles.
- Microservices Architecture Expertise: Deep understanding of designing, developing, deploying, and managing microservices, including experience with containerization (Docker, Kubernetes), service mesh technologies (Istio, Linkerd), and API gateways.
- API Design and Management: Proficiency in designing RESTful APIs, implementing API security measures (OAuth 2.0, OpenID Connect), and managing API lifecycles using tools like Apigee or Kong.
- Cloud Native Development: Extensive experience with cloud platforms (AWS, Azure, GCP), serverless functions, and cloud-native principles like scalability, resilience, and observability. This includes familiarity with IaC (Infrastructure as Code) tools like Terraform or CloudFormation.
- Headless CMS Expertise: Understanding of headless CMS architectures, content modeling, content delivery networks (CDNs), and integration with other systems through APIs. Experience with specific headless CMS platforms (e.g., Contentful, Strapi, Sanity) is highly beneficial.
- DevOps and CI/CD: Strong understanding of DevOps principles, including continuous integration and continuous delivery (CI/CD) pipelines, automated testing, and infrastructure monitoring.
Challenges in Finding and Retaining MACH Expertise
The demand for professionals with these specialized skills significantly outpaces the supply. Many universities and training programs haven’t yet caught up with the rapid evolution of MACH technologies. This creates a competitive landscape, driving up salaries and making it difficult for organizations to attract and retain top talent. Further complicating the matter is the rapid pace of technological change within the MACH ecosystem; continuous learning and upskilling are essential for staying current.
Strategies for Addressing the Skills Gap
Addressing the skills gap requires a multi-pronged approach:
- Invest in Training and Development: Organizations should invest in internal training programs to upskill existing employees and bridge the gap in specific areas. This could involve workshops, online courses, and mentorship programs.
- Strategic Partnerships: Collaborating with universities and training institutions to develop specialized curricula aligned with MACH architecture requirements can help cultivate a future talent pool.
- Competitive Compensation and Benefits: Offering competitive salaries, benefits packages, and opportunities for professional growth is crucial for attracting and retaining skilled professionals.
- Open Source Contributions and Community Engagement: Encouraging employees to contribute to open-source projects related to MACH technologies enhances their skills and visibility, fostering a culture of continuous learning.
Ideal Skillset for a MACH Composable Architect
The ideal MACH composable architect possesses a blend of technical expertise, strategic thinking, and leadership qualities. They should be able to translate business requirements into technical specifications, design robust and scalable architectures, and guide development teams through the implementation process. Their expertise extends beyond individual technologies to encompass the holistic design and management of the entire MACH ecosystem.
This includes a strong understanding of security, performance, and scalability considerations, along with the ability to effectively communicate technical concepts to both technical and non-technical stakeholders. Experience with various MACH platforms and a proven track record of successful MACH implementations are essential. Furthermore, a deep understanding of API-led connectivity and integration strategies is critical. Finally, a proactive approach to staying abreast of the ever-evolving MACH landscape is paramount for continued success.
Final Thoughts
So, is a fully mach composable architecture always the best solution? The answer, as with most things in tech, is a nuanced “it depends.” While the potential benefits are undeniably attractive, the reality is often more complex. Ignoring the potential pitfalls—vendor lock-in, security vulnerabilities, and escalating maintenance costs—can lead to significant problems down the line. By carefully weighing the pros and cons, understanding the inherent complexities, and proactively addressing the challenges discussed, you can make an informed decision that best suits your specific project needs and long-term goals.
Remember, informed choices lead to successful implementations.
FAQ Compilation
What are some common signs that a mach composable solution is failing?
Increased downtime, slow performance, escalating maintenance costs, difficulty integrating new components, and security breaches are all red flags.
How can I avoid vendor lock-in with mach composable solutions?
Choose open standards and APIs, favor solutions with good documentation and community support, and build modularity into your design from the start.
Are there any open-source alternatives to proprietary mach composable platforms?
Yes, several open-source projects provide building blocks for creating mach composable systems, offering greater flexibility and control.
What kind of expertise is needed to effectively manage a mach composable architecture?
A skilled team with expertise in DevOps, cloud computing, security, and specific component technologies is essential for successful implementation and maintenance.