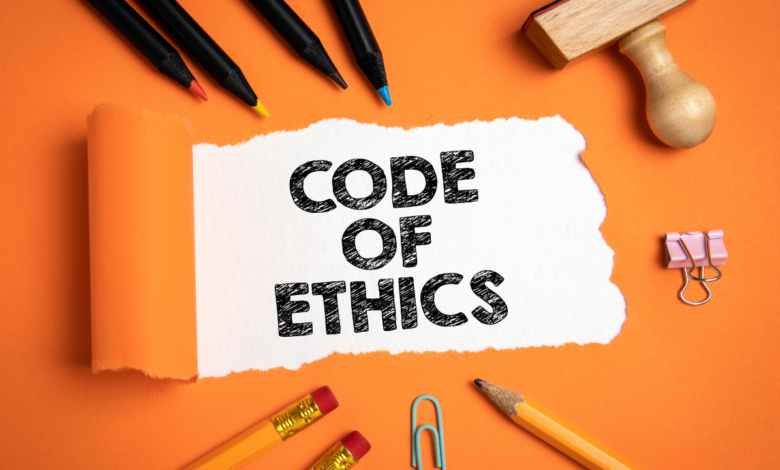
Dont Believe the Hype Mythbusting Zero Trust Marketing
Dont believe the hype myth busting zero trust marketing – Dont Believe the Hype: Mythbusting Zero Trust Marketing – we’ve all seen those flashy ads promising impenetrable security with Zero Trust. But is it all it’s cracked up to be? This post dives deep into the reality of Zero Trust, separating fact from fiction, and helping you decide if it’s the right solution for
-your* needs. We’ll explore the common misconceptions, the limitations, and the often-overlooked costs associated with implementing a Zero Trust architecture.
Get ready to ditch the hype and embrace the truth!
From overblown marketing claims to the practical challenges of implementation, we’ll examine Zero Trust from every angle. We’ll look at real-world examples of successful and, more importantly,
-unsuccessful* deployments, helping you avoid costly mistakes. We’ll also explore the different models of Zero Trust, discuss their suitability for various industries, and provide a realistic assessment of the financial and technical investment required.
By the end, you’ll have a clear understanding of what Zero Trust can—and
-cannot*—do for your organization.
Understanding the Hype Around Zero Trust
Zero Trust architecture has become a buzzword in the cybersecurity industry, often presented as a silver bullet solution to all security woes. However, the reality is far more nuanced. Many vendors and marketing materials significantly oversell its capabilities, creating unrealistic expectations and potentially leading organizations down a costly and ultimately ineffective path. This section aims to clarify the hype surrounding Zero Trust and distinguish between genuine benefits and exaggerated claims.
Common Misconceptions Surrounding Zero Trust
A common misconception is that implementing Zero Trust automatically eliminates all security risks. This is simply false. Zero Trust is a framework, not a product, and its effectiveness depends entirely on proper implementation and ongoing management. Another misconception is that Zero Trust is a simple “plug-and-play” solution. The migration to a Zero Trust model often requires significant changes to existing infrastructure, processes, and employee training, making it a complex and time-consuming undertaking.
Finally, some believe that Zero Trust is only relevant for large enterprises with extensive IT resources. While large organizations may have more complex deployments, the principles of Zero Trust are applicable to organizations of all sizes.
So, you’re hearing all this buzz about Zero Trust, right? Don’t believe the hype! Effective security needs a solid foundation, and that’s where tools like those discussed in this excellent article on bitglass and the rise of cloud security posture management come in. Understanding your cloud’s security posture is crucial before layering on Zero Trust – otherwise, you’re just building a fancy house on a shaky foundation.
Myth busting is key to real security improvements.
Overblown Promises Associated with Zero Trust Implementations
Marketing materials frequently promise complete protection from all cyber threats with Zero Trust. Such claims are misleading. While Zero Trust significantly improves security posture by reducing the attack surface and limiting lateral movement, it doesn’t provide absolute immunity. Vendors often highlight the ability to prevent all breaches, implying a foolproof system. This is an oversimplification.
So, you’re hearing all this zero-trust marketing buzz? Don’t fall for the hype! Building truly secure systems requires a practical approach, and that often means leveraging the right tools. For instance, streamlining development with platforms like those discussed in this article on domino app dev the low code and pro code future can free up your security team to focus on the real threats.
Ultimately, effective security isn’t about flashy marketing; it’s about smart solutions and well-executed strategies.
Sophisticated attackers can still find vulnerabilities to exploit, even within a Zero Trust environment. Some marketing campaigns suggest seamless and effortless implementation, ignoring the substantial effort and resources required for successful deployment.
Examples of Exaggerated Marketing Claims, Dont believe the hype myth busting zero trust marketing
Consider marketing materials that use phrases like “eliminate all breaches” or “unbreakable security” in relation to Zero Trust. These statements are hyperbole and lack the necessary nuance to accurately represent the technology. Another common exaggeration is the portrayal of Zero Trust as a quick fix, promising rapid deployment and immediate results. This ignores the significant planning, configuration, and ongoing maintenance required for a successful Zero Trust implementation.
Furthermore, some marketing campaigns showcase impressive-sounding statistics about threat reduction without providing context or methodology, leading to misleading interpretations.
Comparison of Realistic Zero Trust Benefits and Exaggerated Claims
Realistic Benefit | Exaggerated Claim |
---|---|
Reduced attack surface by limiting network access | Eliminates all security risks and prevents all breaches |
Improved visibility into user activity and data access | Provides complete and foolproof protection against all threats |
Enhanced ability to detect and respond to threats | Guarantees immediate and effortless implementation |
Stronger protection against lateral movement within the network | Offers instant and seamless protection without any ongoing effort |
Mythbusting Common Zero Trust Narratives: Dont Believe The Hype Myth Busting Zero Trust Marketing
Zero Trust is rapidly becoming the security standard, but the hype often overshadows the practical realities. Many organizations jump on the bandwagon without fully understanding the complexities and potential pitfalls, leading to disillusionment and, worse, security vulnerabilities. This section will dissect some prevalent myths surrounding Zero Trust, examining real-world challenges and highlighting the crucial need for careful planning and execution.Zero Trust isn’t a magic bullet; it’s a sophisticated framework requiring significant investment and expertise.
Understanding its limitations and potential risks is just as important as appreciating its benefits.
Real-World Challenges in Zero Trust Deployments
Implementing Zero Trust often encounters unexpected hurdles. For example, a large financial institution attempting a complete Zero Trust migration faced significant delays integrating their legacy mainframe systems. The mainframe’s outdated architecture and lack of robust APIs presented a major obstacle, requiring extensive custom development and testing to achieve the necessary level of granular access control. Another example involves a global retailer that struggled with the scalability of their Zero Trust architecture.
The sheer volume of users and devices accessing their systems worldwide strained their infrastructure and required significant investment in enhanced capacity and optimized network design. These examples demonstrate that a “lift and shift” approach rarely works; thorough planning and phased implementation are critical.
Limitations of Zero Trust in Specific Contexts
Legacy systems pose a significant challenge to Zero Trust adoption. Many organizations rely on outdated systems lacking the necessary security features for seamless integration with a Zero Trust architecture. Retrofitting these systems can be expensive and time-consuming, often requiring significant code modifications or even complete system replacements. Similarly, remote work environments introduce new complexities. Securing a distributed workforce requires robust solutions for managing endpoint devices, ensuring secure network access from various locations, and addressing the increased attack surface associated with home networks.
Security Risks of Poorly Implemented Zero Trust Architectures
A poorly implemented Zero Trust architecture can actually increase security risks. For instance, over-reliance on complex configurations can introduce vulnerabilities. If the architecture isn’t properly designed and tested, it can create single points of failure or introduce unforeseen access control loopholes. Inadequate training for users and administrators can also lead to security breaches. Users may bypass security controls due to lack of understanding, while poorly trained administrators may misconfigure the system, creating vulnerabilities.
Finally, neglecting regular security audits and penetration testing can leave the organization vulnerable to sophisticated attacks.
Common Zero Trust Myths and Realities
Understanding the difference between the hype and reality is crucial for successful Zero Trust implementation.
- Myth: Zero Trust eliminates all security risks. Reality: Zero Trust significantly reduces risks but doesn’t eliminate them entirely. It’s a framework, not a guarantee.
- Myth: Zero Trust is a simple “plug-and-play” solution. Reality: Zero Trust requires significant planning, implementation, and ongoing management.
- Myth: Zero Trust is only for large enterprises. Reality: While the complexity scales with size, Zero Trust principles can be applied to organizations of all sizes, adapting the implementation to their specific needs.
- Myth: Zero Trust is solely about technology. Reality: Zero Trust requires a holistic approach encompassing technology, processes, and people.
- Myth: Implementing Zero Trust is a one-time project. Reality: Zero Trust is an ongoing process requiring continuous monitoring, adaptation, and improvement.
Analyzing the Practical Applications of Zero Trust
Zero Trust architecture isn’t a one-size-fits-all solution; its effectiveness hinges on careful consideration of an organization’s specific needs and context. Understanding where Zero Trust shines brightest and how different models apply to various scenarios is crucial for successful implementation. This section dives into the practical aspects of Zero Trust, exploring its applicability across industries and providing a roadmap for its adoption.Zero Trust’s effectiveness varies significantly across different sectors.
Its inherent security benefits, particularly its focus on least privilege access and continuous verification, make it particularly valuable in industries handling sensitive data or facing high-risk threats.
Zero Trust’s Effectiveness Across Industries
Industries with stringent regulatory requirements, such as finance and healthcare, are prime candidates for Zero Trust. Financial institutions, for example, can leverage Zero Trust to protect sensitive customer data and prevent unauthorized access to financial systems. Similarly, healthcare organizations can use Zero Trust to safeguard patient records and comply with HIPAA regulations. Government agencies, with their need to protect national security information, also benefit greatly from the enhanced security provided by Zero Trust.
In contrast, smaller businesses with less complex IT infrastructure might find the implementation costs and complexity of a full Zero Trust deployment disproportionate to their risk profile. However, even smaller organizations can benefit from implementing certain Zero Trust principles, such as multi-factor authentication and strong password policies.
Comparing Zero Trust Models and Their Applicability
Several Zero Trust models exist, each with its own strengths and weaknesses. The “never trust, always verify” principle remains central, but the specific implementation details differ. For instance, a perimeter-based Zero Trust model focuses on securing the network perimeter, while a user-centric model prioritizes user authentication and authorization. A device-centric model emphasizes securing individual devices. The choice of model depends on factors like the organization’s size, complexity, and risk tolerance.
A large enterprise with a complex IT infrastructure might benefit from a more comprehensive, multi-layered approach, combining elements of several models. A smaller organization might find a more focused, user-centric approach sufficient.
Assessing Zero Trust Suitability for an Organization
Before embarking on a Zero Trust implementation, a thorough assessment is crucial. This involves identifying the organization’s critical assets, assessing the current security posture, and determining the level of risk tolerance. A gap analysis comparing the current security controls to the requirements of a Zero Trust architecture helps prioritize implementation steps. The assessment should also consider the organization’s budget, technical expertise, and overall business objectives.
A phased approach, starting with high-value assets and gradually expanding the Zero Trust deployment, is often recommended. This minimizes disruption and allows for iterative improvements.
Step-by-Step Guide for Implementing Zero Trust Principles
Implementing Zero Trust is an iterative process, not a single event. A phased approach is recommended, focusing on incremental improvements and continuous monitoring.
- Assessment and Planning: Conduct a thorough risk assessment to identify critical assets and vulnerabilities. Develop a detailed implementation plan outlining the phases, timelines, and resources required.
- Identity and Access Management (IAM) Overhaul: Implement strong authentication mechanisms, including multi-factor authentication (MFA), and granular access controls based on the principle of least privilege. This involves creating detailed user roles and permissions, ensuring that users only have access to the resources they absolutely need to perform their job.
- Network Segmentation: Divide the network into smaller, isolated segments to limit the impact of a security breach. This involves using technologies such as virtual LANs (VLANs) and micro-segmentation to create secure zones within the network.
- Data Security and Encryption: Implement robust data encryption both in transit and at rest. This protects sensitive data even if it is compromised. Consider using encryption technologies such as TLS/SSL and AES.
- Continuous Monitoring and Security Information and Event Management (SIEM): Implement continuous monitoring and logging to detect and respond to security threats in real-time. A SIEM system can help consolidate security logs from various sources, providing a centralized view of the security posture.
- Regular Security Audits and Updates: Regularly audit the Zero Trust implementation to identify and address any gaps or weaknesses. Keep all software and systems up-to-date with the latest security patches.
The Cost and Complexity of Zero Trust
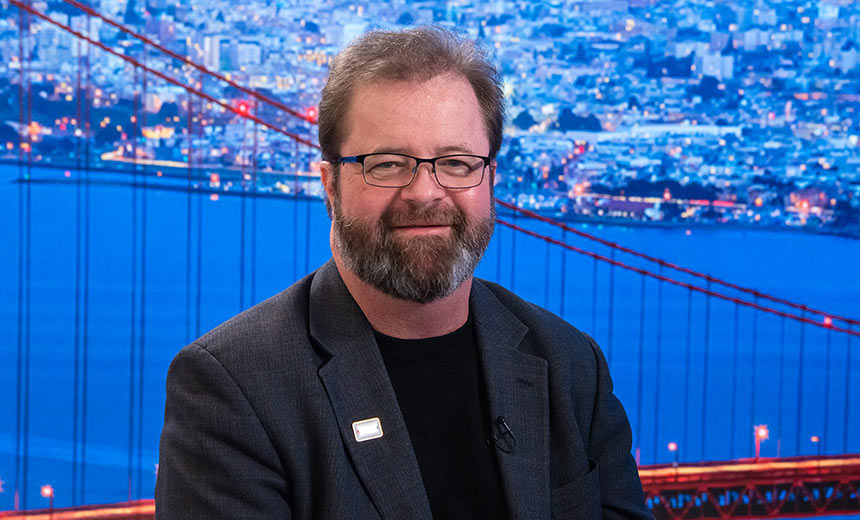
Embracing Zero Trust is not a simple switch-flip; it’s a significant undertaking that demands careful consideration of both financial investment and technical expertise. Many organizations are lured by the promise of enhanced security, only to find themselves grappling with unforeseen costs and complexities during implementation. Understanding these challenges upfront is crucial for a successful deployment.The financial commitment required for a Zero Trust architecture varies significantly depending on the organization’s size, existing infrastructure, and the scope of the implementation.
It’s not just about purchasing new software; it involves a holistic review and potential overhaul of existing systems and processes. This often includes significant professional services fees for planning, implementation, and ongoing maintenance.
Financial Investment in Zero Trust
Implementing Zero Trust necessitates a multifaceted financial investment. Initial costs include the procurement of new security tools such as Software Defined Perimeter (SDP) solutions, multi-factor authentication (MFA) systems, and cloud access security brokers (CASBs). Ongoing expenses encompass licensing fees, maintenance contracts, staff training, and potentially the need for additional IT personnel to manage the increased complexity. A realistic budget should account for these upfront and recurring costs.
For example, a mid-sized company might expect to invest anywhere from $100,000 to $500,000 or more depending on their scale and requirements. Larger enterprises could see investments reaching millions of dollars. These figures are estimates, and the actual cost will be highly dependent on the specific needs of each organization.
Technical Expertise Required for Zero Trust Deployment
Successful Zero Trust deployment hinges on a skilled team possessing a wide range of expertise. This includes network engineers proficient in configuring and managing firewalls, VPNs, and other network security devices; security architects capable of designing and implementing a robust Zero Trust framework; and security analysts skilled in threat detection and incident response. Furthermore, expertise in cloud security, identity and access management (IAM), and data security is essential.
Organizations often need to invest in training existing staff or hire new personnel with the necessary skills. The lack of sufficient in-house expertise often leads to reliance on external consultants, further adding to the overall cost.
Examples of Underestimated Costs and Complexities
Several organizations have learned the hard way about the challenges of underestimating Zero Trust implementation. One notable example is a large financial institution that initially underestimated the integration complexity with their legacy systems. This resulted in significant delays and cost overruns as they struggled to reconcile their existing infrastructure with the demands of a Zero Trust model. Another case involved a healthcare provider that overlooked the need for extensive employee training, leading to user frustration and a slower-than-expected adoption rate.
These instances highlight the importance of thorough planning and realistic cost projections.
Infographic: Cost Components of a Zero Trust Architecture
The infographic would be a circular chart, divided into five colored segments representing the major cost components. The largest segment (30%) would be labeled “Software & Licensing,” depicted with icons of software packages and license keys. The next largest segment (25%) would be “Professional Services,” illustrated with images of consultants and project management tools. A smaller segment (20%) would represent “Hardware,” showing icons of servers, network devices, and endpoint protection solutions.
Another segment (15%) would depict “Training & Education,” with images of online courses and training manuals. The smallest segment (10%) would represent “Ongoing Maintenance & Support,” symbolized by a wrench and a support ticket icon. Each segment would be clearly labeled with its percentage and a brief description. The overall title of the infographic would be “Deconstructing the Cost of Zero Trust.” The circular design visually represents the interconnected nature of the different cost elements.
The Future of Zero Trust Security
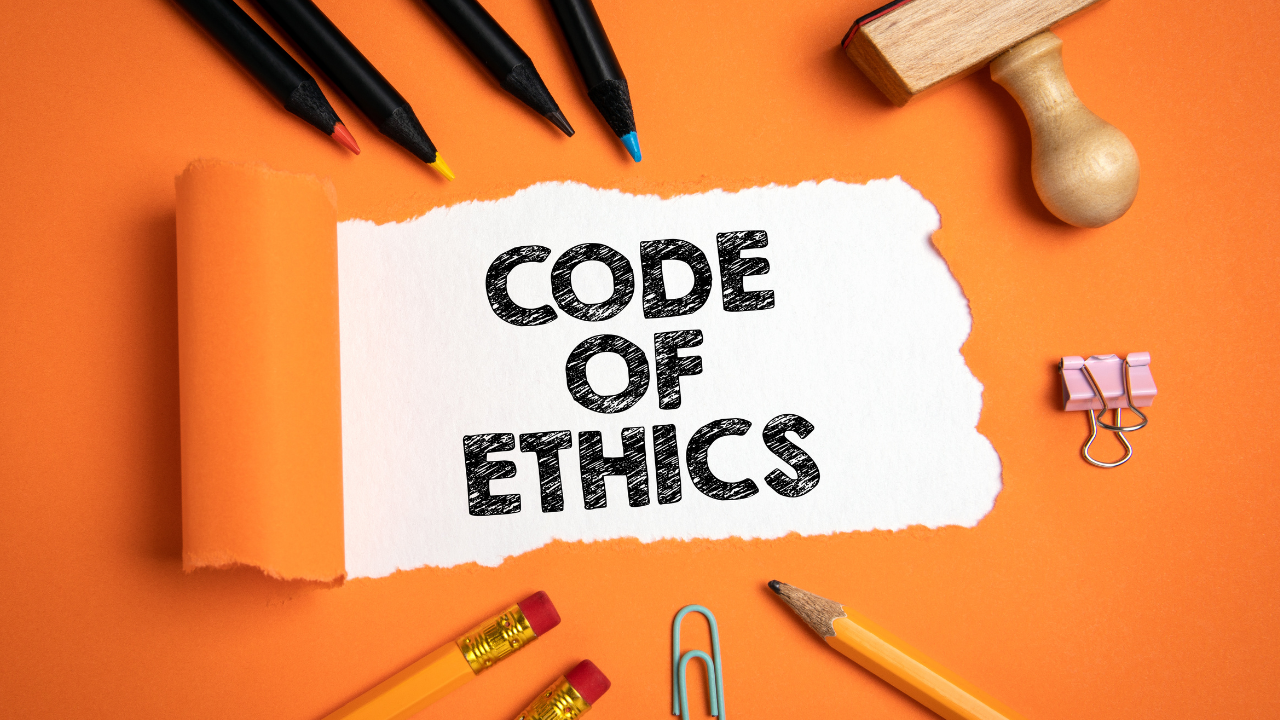
Zero Trust security is rapidly evolving, driven by the increasing sophistication of cyber threats and the ever-expanding attack surface of modern organizations. The future of Zero Trust will be shaped by advancements in several key areas, leading to more robust, adaptable, and user-friendly security frameworks. This evolution will necessitate a continuous adaptation to emerging threats and technologies.
The core principles of Zero Trust – never trust, always verify – will remain central, but the implementation and application of these principles will undergo significant transformation. We’ll see a move towards more automated and intelligent systems, leveraging AI and machine learning to enhance threat detection and response capabilities.
Emerging Technologies Impacting Zero Trust
The convergence of several technologies is poised to significantly enhance Zero Trust deployments. Artificial intelligence (AI) and machine learning (ML) will play a crucial role in automating security tasks, improving threat detection accuracy, and enabling more proactive security measures. For example, AI-powered systems can analyze vast amounts of security data to identify anomalies and potential threats in real-time, significantly reducing the response time to security incidents.
Furthermore, advancements in blockchain technology could improve the security and trustworthiness of identity and access management (IAM) systems, offering enhanced data integrity and tamper-proof authentication. The integration of these technologies into Zero Trust frameworks will lead to a more dynamic and adaptive security posture.
Future Developments Enhancing Zero Trust
We can anticipate several key developments in the coming years that will strengthen Zero Trust principles. The expansion of secure access service edge (SASE) architectures will provide a more unified and simplified approach to securing access to cloud-based resources and applications. SASE integrates network security functions such as Secure Web Gateway (SWG), Cloud Access Security Broker (CASB), and Firewall-as-a-Service (FWaaS) into a single, cloud-delivered service, simplifying management and improving security.
Furthermore, the increasing adoption of decentralized identity management solutions, such as self-sovereign identity (SSI), will empower users to control their own digital identities and enhance privacy while maintaining strong security. This move towards decentralized identity management will align with the core principles of Zero Trust by reducing reliance on centralized authorities and strengthening user control.
Areas for Improvement in Zero Trust Frameworks
While Zero Trust offers significant advantages, certain areas require refinement. One key area is improving the user experience. The strict verification processes inherent in Zero Trust can sometimes lead to friction for users. Future developments should focus on streamlining authentication processes without compromising security. Another challenge lies in the complexity of implementing and managing Zero Trust architectures, particularly in large and complex organizations.
Simplified tools and automation are crucial to making Zero Trust accessible to a wider range of organizations. Finally, addressing the skills gap in cybersecurity professionals is vital for successful Zero Trust implementation and management. Increased investment in training and education programs will be necessary to ensure that organizations have the necessary expertise to effectively manage their Zero Trust deployments.
Anticipated Evolution of Zero Trust (2024-2034)
The following timeline illustrates potential milestones in the evolution of Zero Trust over the next decade:
2024-2026: Widespread adoption of SASE architectures and increased integration of AI/ML in threat detection and response. Focus on improving user experience through streamlined authentication.
2027-2029: Maturation of decentralized identity management solutions, leading to greater user control and enhanced privacy. Increased focus on automating Zero Trust deployment and management.
2030-2034: Emergence of more sophisticated threat detection and response capabilities, leveraging advanced AI/ML and blockchain technologies. Greater emphasis on proactive security measures and continuous security posture monitoring. Ubiquitous adoption of Zero Trust principles across all aspects of IT infrastructure and applications.
Closing Summary
So, is Zero Trust the silver bullet for all your security woes? The answer, as with most things in cybersecurity, is nuanced. While the core principles of Zero Trust are sound – verifying every access request regardless of location – the implementation can be complex, costly, and require significant expertise. This post aimed to cut through the marketing noise and present a realistic picture.
By understanding the limitations and potential pitfalls, you can make an informed decision about whether Zero Trust is the right strategy for
-your* organization, and if so, how to approach it effectively. Remember, informed decisions lead to better security outcomes.
Q&A
What are the biggest risks associated with a poorly implemented Zero Trust architecture?
Poorly implemented Zero Trust can lead to increased attack surface due to misconfigurations, single points of failure, and inadequate monitoring. It can also create usability issues, hindering productivity and potentially leading to users circumventing security controls.
Can Zero Trust work with legacy systems?
Integrating Zero Trust with legacy systems can be challenging but is often possible through careful planning and the use of bridging technologies. It might require phased implementation and may not offer the same level of protection as with modern systems.
How long does it typically take to implement Zero Trust?
Implementation timelines vary greatly depending on the organization’s size, complexity, and existing infrastructure. It can range from several months to several years for large enterprises.