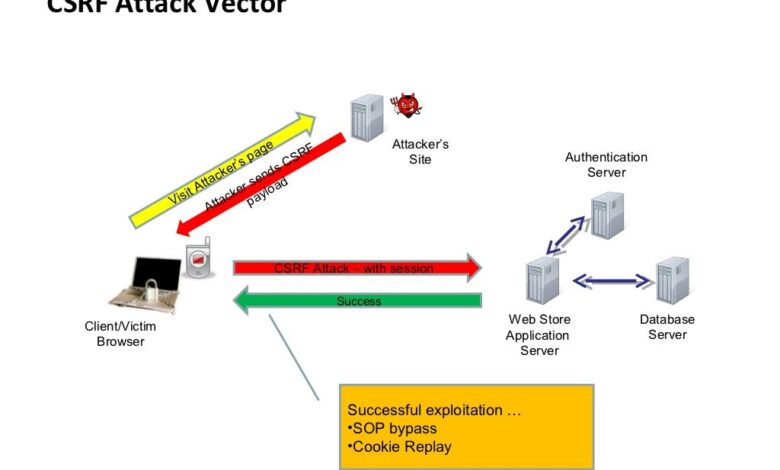
Enabling CSRF and XSS Protections in Zietrans
Enabling CSRF and XSS protections in Zietrans is crucial for building a secure web application. These vulnerabilities, Cross-Site Request Forgery (CSRF) and Cross-Site Scripting (XSS), represent significant threats that can compromise user data and system integrity. This post dives deep into understanding these attacks within the context of Zietrans, exploring practical methods for implementing robust protection measures. We’ll cover everything from understanding the mechanics of these attacks to implementing specific security controls, and finally, combining these strategies for a comprehensive security approach.
We’ll explore best practices, code examples, and different techniques to secure your Zietrans application. Think of this as your comprehensive guide to bolstering your application’s defenses against these pervasive online threats. Get ready to level up your Zietrans security game!
Understanding CSRF in Zietrans
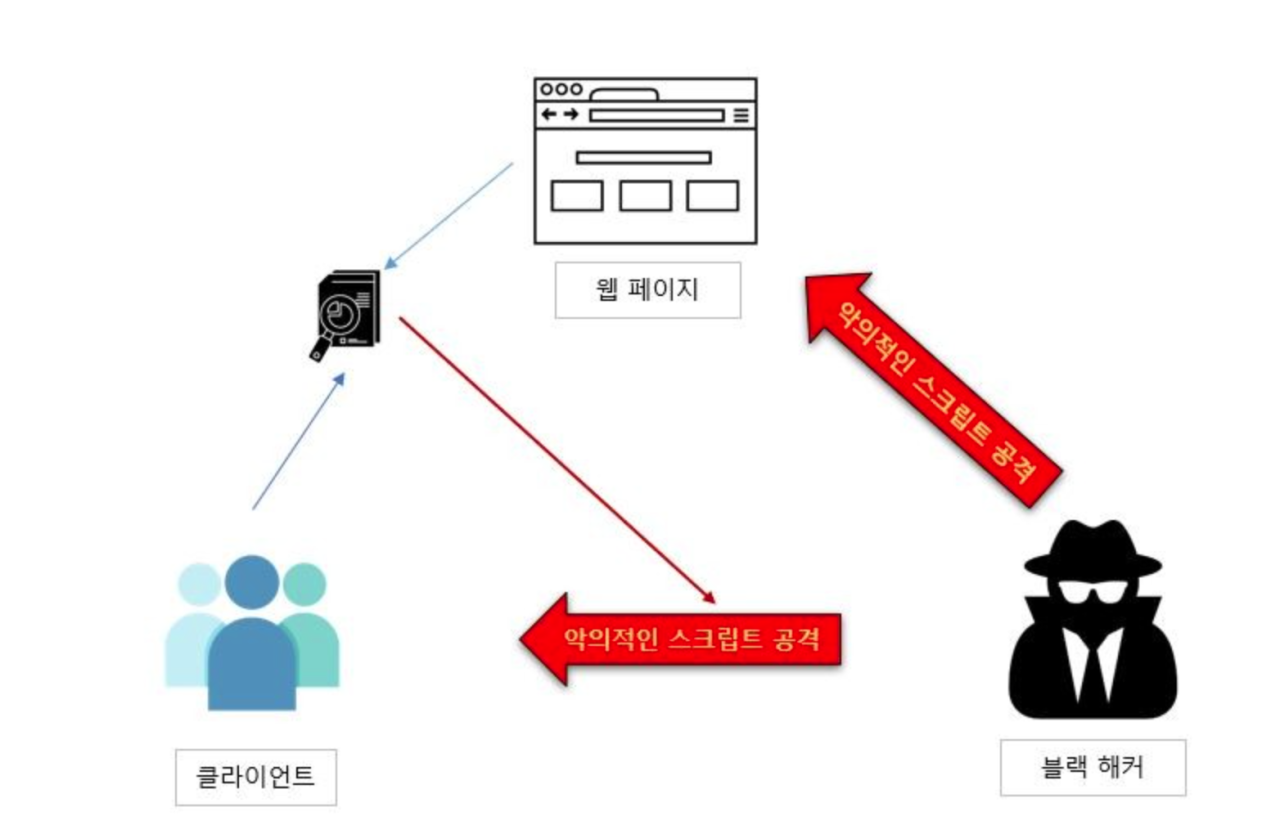
Cross-Site Request Forgery (CSRF) attacks represent a significant threat to web applications, and Zietrans, with its potential for user-initiated actions and sensitive data handling, is not immune. Understanding the mechanics of CSRF and common vulnerabilities within Zietrans is crucial for implementing effective preventative measures. This section will delve into the specifics of CSRF attacks within the context of Zietrans, highlighting potential vulnerabilities and illustrating real-world parallels.CSRF attacks exploit the trust a website has in its users’ browsers.
A malicious website can trick a logged-in Zietrans user into unknowingly submitting a forged request to Zietrans, performing actions they did not intend. This happens because the user’s browser automatically includes authentication cookies (session tokens) with every request to the Zietrans domain, even if the request originates from a different site. The attacker doesn’t need to know the user’s password; they only need to craft a malicious request that leverages the existing session.
Zietrans Vulnerabilities Exploitable via CSRF
Many Zietrans features involving state changes or sensitive data manipulation could be vulnerable to CSRF. For example, features allowing users to update their profiles, change passwords, transfer funds, or modify order details are prime targets. Any action that can be performed with a simple HTTP POST or GET request without additional authentication checks beyond cookies is susceptible. These vulnerabilities stem from a lack of proper CSRF protection mechanisms, such as the use of anti-CSRF tokens or the verification of the HTTP Referer header.
Real-World CSRF Attack Examples
Numerous real-world examples demonstrate the impact of CSRF attacks on similar systems. Consider a banking application where a malicious website embeds a hidden form that initiates a funds transfer. When a logged-in user visits the malicious website, their browser unknowingly sends the transfer request to the bank, transferring funds without their explicit knowledge or consent. Similarly, an e-commerce platform could be targeted, allowing an attacker to place orders or modify existing orders on behalf of unsuspecting users.
These attacks often go unnoticed until the victim checks their account and discovers unauthorized transactions or modifications.
Hypothetical CSRF Attack Scenario Against Zietrans
Imagine a hypothetical scenario where a malicious website contains an image with an embedded form that targets a Zietrans feature allowing users to change their email address. This form is cleverly disguised and automatically submits a POST request to Zietrans’s email update endpoint, changing the user’s email address to one controlled by the attacker. Because the request originates from the user’s browser and includes their valid session cookie, Zietrans would process the request without suspicion.
The attacker gains control of the user’s account, potentially leading to further compromises. This scenario highlights the critical need for robust CSRF protection in Zietrans.
Implementing CSRF Protection in Zietrans
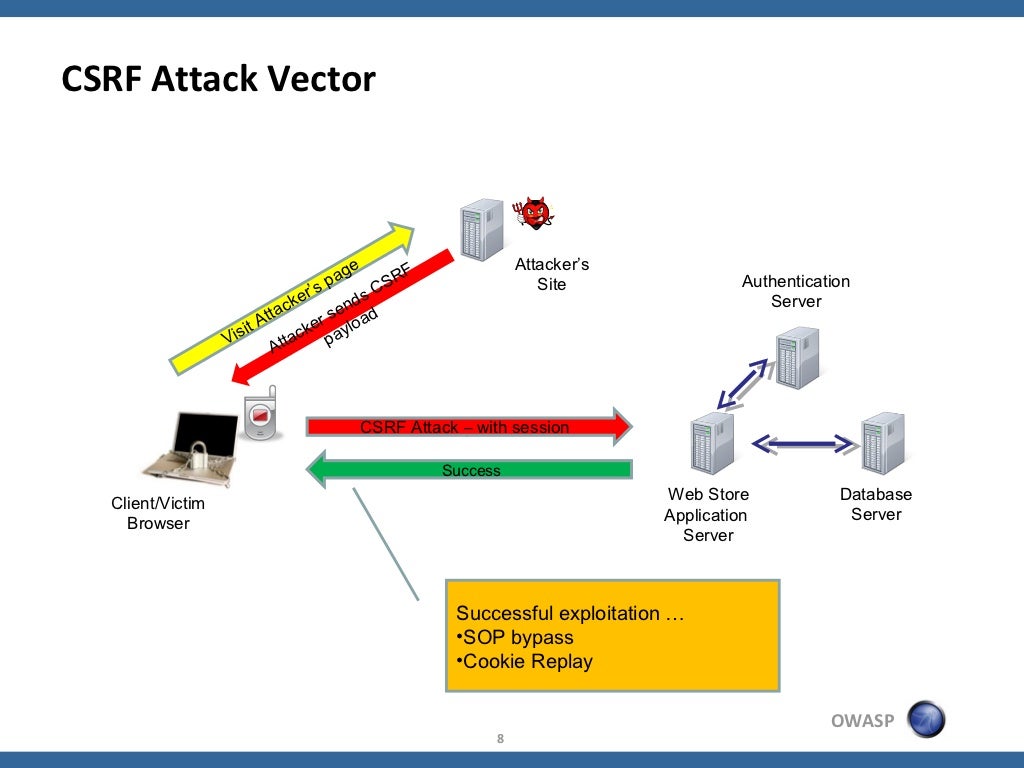
Protecting against Cross-Site Request Forgery (CSRF) attacks is crucial for any web application handling sensitive user data, and Zietrans is no exception. This section delves into practical strategies for implementing robust CSRF protection within the Zietrans framework. We’ll explore the use of CSRF tokens, the role of HTTP methods, and compare various protection techniques.
CSRF Token Implementation
The most effective method for preventing CSRF attacks is the use of synchronizer tokens, often called CSRF tokens. These are unique, unpredictable values generated by the server and included in forms submitted by the user. The server then verifies that the token submitted matches the one it generated, ensuring the request originated from the legitimate Zietrans application and not a malicious third-party site.
This verification process effectively prevents CSRF attacks by confirming that the user initiated the request.
HTTP Method Considerations
HTTP methods play a significant role in CSRF mitigation. GET requests, which are typically used for retrieving data, are inherently more vulnerable to CSRF attacks because they can be easily embedded in malicious links or scripts. POST requests, used for submitting data, are generally safer because they require a more explicit action from the user. In Zietrans, we should prioritize using POST requests for actions that modify data, such as updating user profiles or making purchases.
For read-only operations, GET requests are acceptable, but extra precautions, such as input validation and authorization checks, are still necessary.
CSRF Protection Techniques Comparison
Several techniques exist for implementing CSRF protection, each with its own strengths and weaknesses. While CSRF tokens are the most widely accepted and effective method, other approaches include using the HTTP Referer header or relying on double submit cookies. However, these alternative methods are less reliable and can be easily bypassed. The Referer header is not always reliable, as it can be easily manipulated or omitted by the attacker.
Double submit cookies, while offering an additional layer of security, are more complex to implement and can still be vulnerable if not implemented correctly. For Zietrans, the use of synchronizer tokens offers the best balance of security and ease of implementation.
CSRF Token Implementation in Zietrans Controller
The following code snippet demonstrates a basic implementation of CSRF token generation and verification within a hypothetical Zietrans controller using a fictional framework. Remember that the specific implementation will depend on your chosen framework and its security features. This example focuses on the core principles.
“`java// Controller method to generate and include CSRF token in the formpublic String showForm(HttpServletRequest request, HttpServletResponse response) String csrfToken = generateCsrfToken(); // Generate a unique token request.getSession().setAttribute(“csrfToken”, csrfToken); // Store token in session // … other code to render the form including the hidden csrfToken field … return “myForm”;// Controller method to verify the CSRF token on form submissionpublic String processForm(HttpServletRequest request, HttpServletResponse response) String submittedToken = request.getParameter(“csrfToken”); // Retrieve token from form String sessionToken = (String) request.getSession().getAttribute(“csrfToken”); // Retrieve token from session if (submittedToken != null && submittedToken.equals(sessionToken)) // Token verified, proceed with form processing // … your form processing logic … else // Token mismatch, handle CSRF attack // … error handling, logging, etc. … return “success”;//Helper method to generate a cryptographically secure random tokenprivate String generateCsrfToken() //Implementation using a secure random number generator. This is a simplified example. //In a production environment, use a robust library for token generation. SecureRandom random = new SecureRandom(); byte[] bytes = new byte[32]; random.nextBytes(bytes); return Base64.getEncoder().encodeToString(bytes);“`
This example illustrates the fundamental process. In a real-world scenario, error handling, logging, and more robust token generation are crucial. Always utilize a well-tested and secure library for token generation within your chosen framework to avoid vulnerabilities.
Understanding XSS in Zietrans
Cross-Site Scripting (XSS) vulnerabilities are a serious threat to web applications like Zietrans, allowing attackers to inject malicious scripts into the application’s output, which is then executed by unsuspecting users’ browsers. Understanding potential entry points and the different types of XSS attacks is crucial for implementing effective security measures.
Potential XSS Entry Points in Zietrans, Enabling csrf and xss protections in zietrans
Zietrans, like many web applications, likely has several potential entry points for XSS attacks. These include user input fields (such as those for profiles, comments, or messages), dynamic content generation based on user-supplied data, and insufficiently sanitized data from external sources. For instance, if Zietrans allows users to upload profile pictures with metadata containing malicious scripts, this could be an entry point.
Similarly, if the application displays user-generated content directly without proper sanitization, an attacker could inject malicious JavaScript code within that content. Insufficient input validation in search functionalities or any dynamic form submission could also lead to vulnerabilities.
Securing Zietrans applications means prioritizing CSRF and XSS protections; it’s a crucial step in building robust and reliable systems. This ties directly into the broader conversation around modern application development, as highlighted in this excellent article on domino app dev the low code and pro code future , where efficient development practices are key to security. Getting those foundational security measures right in Zietrans, like preventing CSRF and XSS attacks, is a fundamental building block for any successful project.
Types of XSS Attacks and Their Impact on Zietrans
Three primary types of XSS attacks exist: reflected, stored, and DOM-based. Reflected XSS occurs when malicious scripts are reflected back to the user from the server, usually within the URL. Stored XSS, also known as persistent XSS, involves injecting malicious scripts into the application’s database, which are then permanently stored and served to all users. DOM-based XSS targets the Document Object Model (DOM) of the browser, manipulating the client-side JavaScript to execute malicious code.In Zietrans, a reflected XSS attack might involve an attacker crafting a malicious link that, when clicked by a user, injects a script into the application’s response.
A stored XSS attack could involve a user posting a comment containing malicious JavaScript code that is then stored and displayed to other users. A DOM-based XSS attack could manipulate the application’s JavaScript to steal user cookies or session IDs, potentially leading to account hijacking. The impact on Zietrans could range from minor annoyances (like pop-up ads) to complete account compromise and data theft.
Examples of Malicious Scripts
A simple reflected XSS example could be a link like this: `https://zietrans.com/search?query= `. When a user clicks this link, the browser will execute the `alert()` function, displaying a popup. A more sophisticated attack could steal cookies using a script like: ` `. This script would send the user’s cookies to the attacker’s server. A stored XSS attack might involve a user posting a comment containing ` `, redirecting other users to a malicious site.
Severity and Potential Impact of XSS Vulnerabilities in Zietrans
Vulnerability Type | Severity | Potential Impact | Mitigation |
---|---|---|---|
Reflected XSS | Medium | Session hijacking, phishing attacks, data theft (if sensitive data is reflected) | Input validation and encoding of all user-supplied data in URLs and responses. |
Stored XSS | High | Account compromise, data theft, website defacement, spreading malware | Strict input sanitization and output encoding for all user-generated content stored in the database. Regular security audits. |
DOM-based XSS | Medium to High | Session hijacking, data theft, redirection to malicious sites | Secure coding practices, careful use of JavaScript libraries, and regular security testing. |
Implementing XSS Protection in Zietrans: Enabling Csrf And Xss Protections In Zietrans
Protecting Zietrans from Cross-Site Scripting (XSS) attacks is crucial for maintaining the security and integrity of the application and its users’ data. XSS vulnerabilities allow attackers to inject malicious scripts into web pages viewed by other users, potentially stealing sensitive information, redirecting users to phishing sites, or defacing the website. Implementing robust XSS protection involves a multi-layered approach, combining several key strategies.Input Validation and Sanitization in ZietransInput validation and sanitization are fundamental to preventing XSS attacks.
This process involves carefully examining all user-supplied data before it’s processed by the application. Validation ensures the data conforms to expected formats and constraints, while sanitization removes or neutralizes potentially harmful characters. For example, a field expecting only numeric input should reject alphabetic characters. Sanitization might involve escaping special characters like ` <`, `>`, `”`, and `’`, preventing them from being interpreted as HTML or JavaScript code. Failing to validate and sanitize input leaves your application vulnerable to injection attacks.Output Encoding (HTML Escaping) in ZietransEven with input validation, output encoding is essential. This technique involves converting special characters into their corresponding HTML entities. For instance, the `<` character becomes `<`, preventing the browser from interpreting it as the start of an HTML tag. This is particularly crucial when displaying user-supplied data on web pages. Failing to properly encode output allows attackers to inject malicious scripts that will be executed by the user's browser. Consistent application of HTML escaping across all output points is vital. Implementing Content Security Policy (CSP) Headers in Zietrans Content Security Policy (CSP) is a powerful mechanism for mitigating XSS attacks by defining a whitelist of sources from which the browser is allowed to load resources, such as scripts, stylesheets, and images. This helps prevent the execution of malicious scripts from untrusted domains. CSP headers are added to the HTTP response, instructing the browser on the allowed sources. A well-configured CSP header can significantly reduce the impact of successful XSS attacks, even if some sanitization fails. For example, a CSP header might restrict script execution to only the application's own domain. Using a Web Application Firewall (WAF) to Protect Zietrans from XSS Attacks A Web Application Firewall (WAF) acts as a reverse proxy, inspecting incoming and outgoing traffic to filter malicious requests and responses. WAFs can detect and block XSS attacks by analyzing the content of HTTP requests and responses for malicious patterns. They can identify and block requests containing suspicious script injections, even if they bypass other security measures. While a WAF is not a replacement for proper coding practices, it provides an additional layer of defense against XSS attacks. Many cloud providers offer WAF services that can be easily integrated into your Zietrans application. Implementing Input Sanitization in a Zietrans Form Handling Function Consider a simple Zietrans form with a text input field for a user's name. A vulnerable implementation might directly insert the user's input into the database and then display it on a webpage without any sanitization. A secure implementation would involve sanitizing the input before storing and displaying it. ```python def handle_user_input(user_input): # Sanitize the input using a library like bleach sanitized_input = bleach.clean(user_input, tags=[], attributes=[], styles=[], strip=True) # Store the sanitized input in the database # ... database operations ... # Display the sanitized input on the webpage return sanitized_input
``` This example demonstrates the use of the `bleach` library (a Python library for HTML sanitization) to clean the user input before storing and displaying it. This prevents the injection of malicious HTML or JavaScript code. Similar sanitization techniques should be applied to all user inputs throughout the Zietrans application.Combining CSRF and XSS Protections in Zietrans
Protecting Zietrans from both Cross-Site Request Forgery (CSRF) and Cross-Site Scripting (XSS) attacks requires a multi-layered approach.
While these vulnerabilities are distinct, they can interact in complex ways, and a robust security strategy must address both simultaneously to minimize risk. Ignoring one while focusing on the other leaves the system vulnerable to the neglected attack vector.
A Comprehensive Security Strategy for Zietrans
A successful security strategy integrates various CSRF and XSS prevention techniques. For CSRF, this includes using the Synchronizer Token Pattern, which involves generating unique, unpredictable tokens for each request, validating these tokens on the server-side, and ensuring that the tokens are not predictable. For XSS, robust input validation and output encoding are crucial, preventing malicious scripts from being injected and executed.
Additionally, a Content Security Policy (CSP) can be implemented to further restrict the resources the browser is allowed to load, mitigating the impact of successful XSS attacks. The combination of these techniques creates a robust defense against both attack vectors.
Interactions Between CSRF and XSS Vulnerabilities
A successful XSS attack can often be leveraged to facilitate a CSRF attack. For example, an attacker might inject malicious JavaScript code via an XSS vulnerability that automatically submits a crafted form on behalf of the victim, bypassing CSRF protections that rely solely on token validation. This highlights the need for a holistic approach, considering the potential synergy between these vulnerabilities.
The impact of a combined attack can be significantly more severe than either attack in isolation, potentially leading to complete account compromise and data theft.
Security Measures Checklist for CSRF and XSS Protection
The following checklist Artikels essential security measures for both CSRF and XSS protection in Zietrans:
- CSRF Protection: Implement the Synchronizer Token Pattern for all sensitive actions. Regularly rotate tokens and ensure they are stored securely.
- CSRF Protection: Use the HTTP Strict Transport Security (HSTS) header to enforce HTTPS connections, making it more difficult for attackers to intercept requests and manipulate tokens.
- XSS Protection: Implement robust input validation and sanitization for all user-supplied data. This includes escaping special characters to prevent script injection.
- XSS Protection: Use output encoding appropriate to the context (HTML, JavaScript, etc.) to ensure that user-supplied data is rendered safely.
- XSS Protection: Implement a Content Security Policy (CSP) to control the resources the browser is allowed to load, reducing the impact of successful XSS attacks.
- XSS Protection: Regularly scan for and patch XSS vulnerabilities using automated tools and manual code reviews.
- Combined Protection: Conduct regular security audits and penetration testing to identify and address potential vulnerabilities in the interaction between CSRF and XSS protection mechanisms.
Comparing CSRF and XSS Prevention Methods
Different combinations of prevention methods offer varying levels of protection. For instance, relying solely on input validation for XSS is less effective than combining it with output encoding and a CSP. Similarly, using only HTTP Referer checks for CSRF is weak compared to employing the Synchronizer Token Pattern. A layered approach, combining multiple techniques, significantly increases the overall security posture.
The effectiveness is directly proportional to the number of layers and their robustness. For example, a system using only Synchronizer Tokens is more secure than one relying only on HTTP Referer checks.
Layered Security Approach for Zietrans
A layered security approach involves implementing multiple independent security controls to protect against both CSRF and XSS attacks. This means that even if one layer fails, others will still provide protection. This approach is crucial because attackers often attempt to bypass security mechanisms by exploiting weaknesses in a single layer. For example, a system could employ input validation, output encoding, a CSP, and the Synchronizer Token Pattern simultaneously.
If one fails, others still protect against attacks. This layered approach significantly reduces the risk of successful attacks, providing robust security for Zietrans.
Closing Notes
Securing Zietrans against CSRF and XSS attacks requires a multi-layered approach. By understanding the vulnerabilities and implementing the techniques discussed – from CSRF tokens and input sanitization to CSP headers and a WAF – you can significantly reduce your risk profile. Remember, a proactive and comprehensive security strategy is vital, not just a reactive one. Regular security audits and updates are key to maintaining a robust and secure Zietrans application.
Stay vigilant and keep your users safe!
FAQ Overview
What is the difference between a CSRF and an XSS attack?
CSRF exploits the trust a website has in a user’s browser, tricking the user into performing unwanted actions. XSS injects malicious scripts into a website, often stealing user data or redirecting them to malicious sites.
Can a WAF completely prevent all CSRF and XSS attacks?
No, a WAF is a valuable tool, but it’s not a silver bullet. It can mitigate many attacks, but it’s crucial to combine it with other security measures like input validation and output encoding for comprehensive protection.
How often should I update my Zietrans application’s security measures?
Regularly – ideally, as soon as security patches and updates are released. Staying up-to-date is critical in mitigating newly discovered vulnerabilities.
Are there any free tools to help with CSRF and XSS protection?
Many open-source tools and libraries exist to aid in input validation and output encoding, forming the core of XSS protection. For CSRF, implementing token-based systems is relatively straightforward.