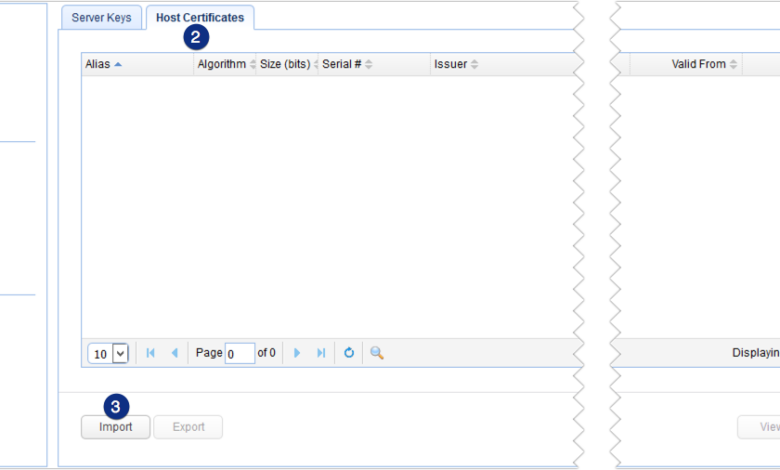
Handling Host Function Keys Externally
Handling host function keys externally: It sounds complex, right? But think about it – giving your application the power to respond to keystrokes outside its normal confines opens up a world of possibilities. Imagine custom shortcuts, seamless integration with other programs, or even creating entirely new interaction paradigms. This post dives into the exciting world of external key handling, exploring the techniques, challenges, and security implications involved.
We’ll cover everything from basic concepts to advanced implementation strategies, ensuring you’re well-equipped to harness the power of external keys.
We’ll be examining different methods for capturing these external keystrokes, comparing their pros and cons, and looking at practical examples. We’ll also address crucial security considerations to ensure your application remains robust and protected. Get ready to unlock a new level of control and flexibility in your applications!
Defining External Host Function Key Handling
Handling host function keys, those special keys often used for actions like volume control or screen brightness, typically involves either internal or external methods. Internal handling integrates the key processing directly within the application or operating system, while external handling involves a separate process or system that intercepts and manages these keys before they reach the application.External host function key handling offers several advantages over internal methods.
It provides a centralized point of control, allowing for consistent behavior across different applications. This is particularly useful for maintaining a uniform user experience or for implementing system-wide key remapping. Furthermore, external handling can improve performance by reducing the overhead on individual applications, as they no longer need to individually process each key press. Finally, it allows for greater flexibility in handling complex key combinations or sequences that might be difficult to manage internally.
Benefits of External Key Handling
External handling excels in scenarios requiring consistent behavior across multiple applications. For instance, imagine a multimedia application needing to control volume regardless of which window is active. Internal handling would require each application to individually implement volume control, leading to potential inconsistencies. External handling provides a single point of control, ensuring consistent volume adjustment regardless of the active application.
Another benefit is enhanced security. Sensitive functions triggered by host keys can be secured by the external handler, preventing unauthorized access. Finally, external handling is beneficial when dealing with legacy applications or those with limited key handling capabilities. The external handler acts as an intermediary, providing a consistent and up-to-date method of handling these keys.
Comparison of External Key Handling Approaches
Several approaches exist for external host function key handling, each with its own strengths and weaknesses. We can compare three common methods: using a dedicated driver, employing a system-wide hotkey utility, and leveraging a scripting language.
Method | Description | Advantages | Disadvantages |
---|---|---|---|
Dedicated Driver | A custom driver intercepts keyboard input at a low level, before it reaches the operating system. | High performance, system-wide control, precise key detection. | Requires driver development expertise, can be complex to install and maintain, potential for system instability if poorly implemented. |
System-wide Hotkey Utility | A software application monitors keyboard input and triggers actions based on predefined hotkeys. | Relatively easy to implement and use, widely available options, good for simple key remapping. | Can conflict with other applications, might not handle all key combinations, may have performance limitations compared to drivers. |
Scripting Language (e.g., AutoHotkey) | A scripting language is used to create scripts that intercept and handle keyboard events. | Flexible, customizable, relatively easy to learn, cross-platform compatibility (depending on the scripting language). | Requires scripting knowledge, performance can be an issue for complex scripts, may not be as reliable as dedicated drivers or utilities. |
Implementation Techniques
Intercepting and processing host function keys externally requires a multifaceted approach, leveraging operating system capabilities and programming techniques to achieve reliable and efficient key handling. The specific methods employed will depend heavily on the target operating system and the level of access required.Several common techniques exist for achieving this. These techniques range from low-level system calls to higher-level library functions, each offering a different balance between control and ease of implementation.
The choice of method depends heavily on factors such as the operating system, programming language, and the desired level of control over the key handling process.
System-Level APIs for External Key Handling, Handling host function keys externally
Operating systems provide APIs allowing applications to monitor keyboard input. On Windows, the `SetWindowsHookEx` function, with the `WH_KEYBOARD_LL` hook type, allows an application to intercept all keyboard events before they reach other applications. Similarly, on Linux, the `X11` library offers functions to monitor keyboard events, providing a way to capture keys before they reach the window manager. These APIs allow for very granular control, enabling the interception of specific keys or key combinations.
However, using these APIs often requires a deep understanding of the underlying operating system and its event handling mechanisms. Improper usage can lead to system instability.
Library-Based Approaches
Higher-level libraries can simplify the process of external key handling. These libraries often abstract away the complexities of system-level APIs, providing a more user-friendly interface. For instance, Python’s `pynput` library provides a cross-platform way to monitor keyboard and mouse input. This approach reduces development time and complexity compared to direct system-level API calls. However, the level of control offered by libraries might be less granular than that provided by direct system calls.
Challenges and Considerations
Implementing external key handling presents several challenges. One significant challenge is the potential for conflicts with other applications that might also be intercepting keyboard input. Careful design and implementation are necessary to avoid deadlocks or unexpected behavior. Another crucial consideration is security. Applications with external key handling capabilities must be carefully designed to prevent malicious actors from exploiting them.
Furthermore, ensuring compatibility across different operating systems and hardware configurations adds another layer of complexity.
Code Examples
Here are examples demonstrating external key handling using Python’s `pynput` library and a conceptual C++ example using a hypothetical OS-specific API.
Python (pynput)
from pynput import keyboarddef on_press(key): try: if key == keyboard.Key.f12: print('F12 pressed') # Perform action here except AttributeError: print('special key 0 pressed'.format(key))with keyboard.Listener(on_press=on_press) as listener: listener.join()
Conceptual C++ Example
// Hypothetical OS-specific APIbool registerKeyHook(KeyHookCallback callback);void unregisterKeyHook();// Callback functionbool keyHookCallback(int keycode);int main() if (registerKeyHook(keyHookCallback)) // Keep application running while (true); return 0;bool keyHookCallback(int keycode) if (keycode == F12_KEYCODE) // Perform action here return true; // Consume the key event return false; // Pass the event to other applications
Flowchart for External Host Function Key Handling
The flowchart would depict the following steps:
1. Register Hook
The application registers a keyboard hook with the operating system.
2. Wait for Event
The application waits for a keyboard event.
3. Event Received
A keyboard event is received.
4. Key Check
The application checks if the received event corresponds to the desired host function key (e.g., F12).
5. Action
Ever wrestled with handling host function keys externally in your applications? It’s a common challenge, especially when building complex interfaces. Understanding how to manage this effectively is key, and learning more about efficient development strategies helps. For instance, check out this insightful article on domino app dev the low code and pro code future which discusses modern approaches to app development.
This knowledge directly impacts how you design your solutions for external key handling, leading to cleaner, more robust code.
If the key matches, the application performs the predefined action.
6. Event Handling
The application decides whether to consume the key event or pass it on to other applications.
7. Loop
Steps 2-6 are repeated until the hook is unregistered.
8. Unregister Hook
The application unregisters the keyboard hook.The flowchart would visually represent this sequence using boxes and arrows, clearly indicating the flow of execution. Each step would be represented by a distinct box, and the arrows would show the transitions between steps. For example, a diamond shape could represent the “Key Check” step, with branches indicating whether the key matches or not.
Security Considerations: Handling Host Function Keys Externally
Handling host function keys externally introduces several security risks that need careful consideration. Improperly secured external access can lead to unauthorized control of the host system, data breaches, and denial-of-service attacks. Robust security measures are crucial to mitigate these threats and ensure the integrity and confidentiality of the system.
Input Validation
Input validation is the first line of defense against many security vulnerabilities. External inputs, such as keystrokes or commands received from an external source, should be rigorously validated to prevent malicious code injection or buffer overflows. This involves checking the input’s type, length, format, and range against predefined acceptable values. For example, if the external key is expected to be a numerical ID, the system should reject any non-numeric input or inputs outside the valid ID range.
Failing to perform proper input validation can lead to vulnerabilities like SQL injection, where malicious code is injected into database queries, potentially compromising sensitive data. A robust validation system should use parameterized queries or prepared statements to prevent such attacks.
Authorization Checks
Before allowing any action based on an external host function key, the system must verify the user’s or application’s authorization to perform that action. This involves checking the user’s credentials and permissions against a predefined access control list (ACL). Multi-factor authentication (MFA) can add an extra layer of security, requiring multiple forms of authentication (e.g., password and a one-time code) before granting access.
A lack of proper authorization checks allows unauthorized users to execute commands or access resources they shouldn’t have access to, leading to data breaches or system compromise. Role-based access control (RBAC) is a good approach for managing user permissions effectively.
Secure Coding Practices
Secure coding practices are paramount in preventing vulnerabilities. This includes using secure libraries and avoiding common coding errors such as buffer overflows, race conditions, and memory leaks. Regular security audits and penetration testing should be conducted to identify and address potential vulnerabilities. Following coding standards and best practices, such as using parameterized queries to prevent SQL injection, can significantly reduce the risk of attacks.
Developers should also be trained on secure coding principles and be aware of common attack vectors. Failing to follow secure coding practices can result in vulnerabilities that attackers can exploit to gain unauthorized access or control.
Vulnerabilities and Mitigations
One example of a vulnerability is a buffer overflow, where an external input exceeds the allocated buffer size, potentially overwriting adjacent memory regions and leading to system crashes or arbitrary code execution. Mitigation involves careful input validation to ensure that the input length does not exceed the buffer size and using safe string handling functions that prevent buffer overflows.
Another example is cross-site scripting (XSS), where malicious JavaScript code is injected into the system and executed by the user’s browser. Mitigation involves proper input sanitization and output encoding to prevent malicious scripts from being executed.
Best Practices for Secure External Key Handling
- Implement robust input validation to prevent malicious code injection.
- Enforce strict authorization checks using multi-factor authentication and role-based access control.
- Adhere to secure coding practices and use secure libraries.
- Regularly conduct security audits and penetration testing.
- Employ encryption to protect keys both in transit and at rest.
- Use a strong key management system to generate, store, and rotate keys securely.
- Monitor system logs for suspicious activity.
- Implement appropriate logging and auditing mechanisms to track key usage and access attempts.
Integration with Applications
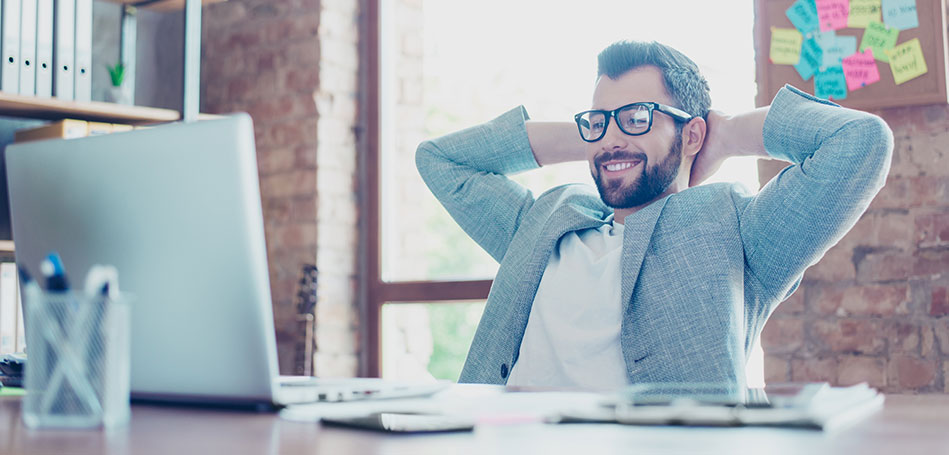
Integrating external host function key handling into various applications presents unique challenges and opportunities depending on the application’s architecture and environment. The core concept remains the same – intercepting and processing keystrokes before they reach the application’s internal event loop – but the implementation details vary significantly. This section explores how to integrate this functionality into different application types and address potential conflicts.
The key to successful integration lies in understanding the application’s event handling mechanism. Desktop applications typically rely on operating system APIs for event processing, while web applications use browser-based event listeners. Embedded systems often involve direct hardware interaction. Each requires a different approach to intercept and manage external key events.
Desktop Application Integration
Desktop applications, built using frameworks like Qt, Electron, or native APIs (like Win32 or Cocoa), usually provide mechanisms for registering custom event handlers. These handlers can intercept keystrokes before the application’s internal logic processes them. For example, a Qt application might use the `QEvent` system to intercept `QKeyEvent` objects. The external key handling module would need to register itself as an event filter, allowing it to examine and potentially modify or consume keyboard events before they reach the application’s widgets.
A similar approach applies to other frameworks, leveraging their respective event handling systems. Proper error handling is crucial, ensuring graceful degradation if the external module fails to function correctly.
Web Application Integration
Integrating external key handling into web applications requires a different strategy. Since web applications run within a browser’s sandboxed environment, direct access to the operating system’s keyboard input is limited. The solution involves using JavaScript event listeners, specifically the `keydown` and `keyup` events. These events provide information about the pressed key. However, the external key handling would need to be implemented as a separate JavaScript library or extension, communicating with a server-side component (potentially using WebSockets or Server-Sent Events) to perform actions that require operating system-level access.
This server-side component would then act as an intermediary, translating browser-based key events into system-level commands. Security is paramount in this architecture, requiring careful consideration of data transmission and authentication mechanisms.
Embedded System Integration
Embedded systems present a unique set of challenges. Direct hardware interaction is often necessary, requiring a deep understanding of the system’s architecture and peripherals. External key handling might involve interacting with low-level hardware drivers to intercept keystrokes before they reach the embedded application’s main loop. The implementation will depend heavily on the specific hardware and the operating system (or real-time operating system – RTOS) used.
Consideration must be given to real-time constraints and resource limitations, as embedded systems often have limited processing power and memory.
Scenario: Integrating External Key Handling into a Media Player
Imagine a media player application that needs to support custom hotkeys for controlling playback (e.g., pausing, skipping tracks). The application could integrate an external key handling module that listens for specific key combinations. When a predefined hotkey is pressed, the module sends a message to the media player application (using inter-process communication, like named pipes or sockets), instructing it to perform the corresponding action.
The media player application’s internal key handling would ignore these keys, preventing conflicts. This module would need error handling to gracefully manage scenarios such as the media player application not responding or the external module itself failing.
Handling Conflicts Between External and Internal Key Handling
Conflicts between external and internal key handling mechanisms can arise when both try to process the same keystrokes. The solution is careful design and prioritization. The external key handling module should have higher priority, consuming events that it recognizes and preventing them from reaching the application’s internal event handlers. This can be achieved by using techniques like event filtering (in desktop applications) or event capturing (in web applications).
A well-defined protocol for communication between the external module and the application is crucial to avoid race conditions and unexpected behavior. Clear documentation of which keys are handled externally and which are handled internally is essential for developers and users.
Troubleshooting and Debugging
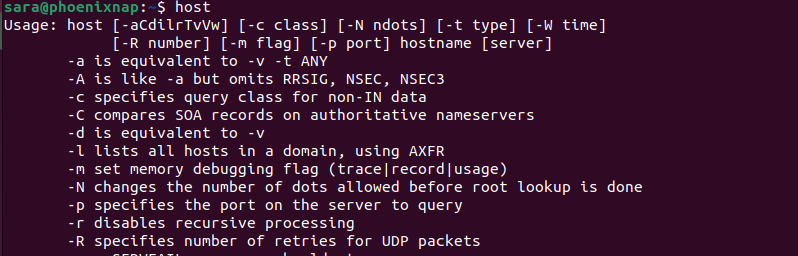
Implementing external host function key handling can be tricky, and you’ll likely encounter some snags along the way. This section dives into common problems, offering solutions and debugging strategies to get your system running smoothly. We’ll cover identifying issues, using debugging tools, and effectively logging events for easier troubleshooting.
Common Problems and Solutions
Several issues frequently arise when managing external keystrokes. These range from simple configuration errors to more complex integration problems. Understanding these common pitfalls is crucial for efficient debugging.
Error | Cause | Solution |
---|---|---|
Keys not registered | Incorrect configuration of the external key handling system; missing or improperly formatted key mappings; driver issues. | Verify all configuration files for accuracy; check key mapping definitions against the intended behavior; ensure the appropriate drivers are installed and functioning correctly. Reinstall drivers if necessary. |
Keystrokes delayed or dropped | Network latency; buffer overflow in the external key handling system; conflicts with other applications accessing the keyboard input. | Investigate network connectivity; increase buffer size if applicable; check for conflicting applications and prioritize the external key handling system. Implement error handling and retry mechanisms. |
System instability or crashes | Memory leaks; race conditions in the external key handling code; improperly handled exceptions. | Use memory profiling tools to detect and address memory leaks; carefully review code for potential race conditions and implement appropriate synchronization mechanisms; implement robust exception handling to prevent crashes. |
Security vulnerabilities | Insufficient input validation; lack of authentication or authorization mechanisms; insecure communication channels. | Implement robust input validation to prevent injection attacks; use secure authentication and authorization protocols; encrypt communication channels using secure protocols like TLS/SSL. |
Debugging Tools and Techniques
Effective debugging requires the right tools and techniques. Utilizing debuggers, loggers, and system monitors can significantly reduce troubleshooting time.Debugging tools such as those built into IDEs (Integrated Development Environments) allow you to step through code line by line, inspect variables, and set breakpoints. This allows for granular examination of the key handling process. For example, setting breakpoints at key registration points can help identify if keys are registered correctly or if there are issues with the registration process.
System monitors can help identify performance bottlenecks or resource exhaustion, which could be contributing to key handling problems.
Logging and Monitoring External Key Handling Events
Comprehensive logging is essential for debugging. Implement logging mechanisms that record key events, timestamps, and relevant system information. This detailed record allows for easier identification of patterns, anomalies, and the root cause of errors. For example, logging could include the key pressed, the timestamp, the application receiving the keystroke, and any errors encountered during processing. This detailed logging can be invaluable in pinpointing the source of issues, particularly in complex systems or when dealing with intermittent problems.
Consider using structured logging formats like JSON for easier parsing and analysis of the logs. This structured data can then be used for automated analysis or integrated with monitoring dashboards.
Wrap-Up
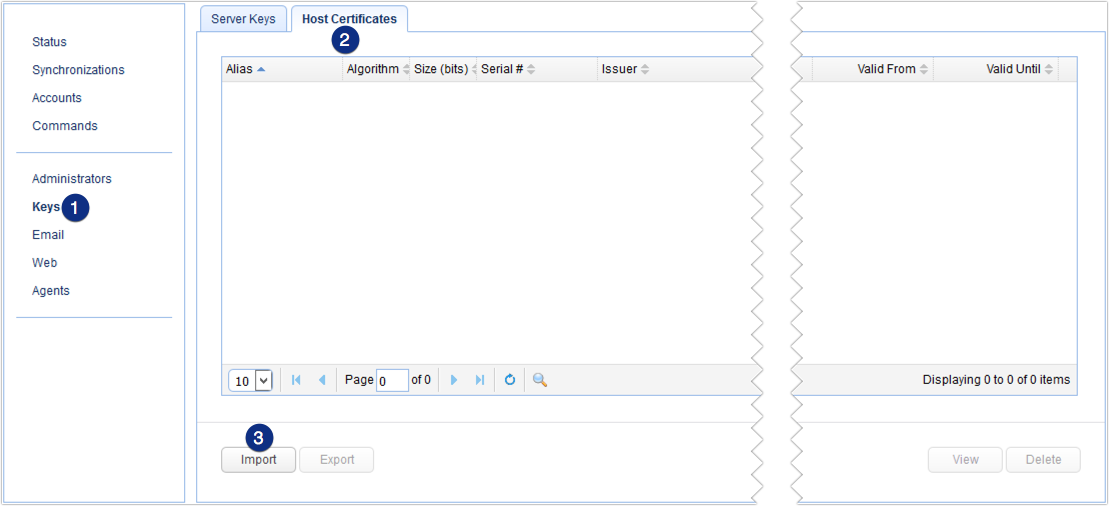
Mastering external host function key handling is about more than just intercepting keystrokes; it’s about crafting a more intuitive and powerful user experience. By understanding the various techniques, security implications, and integration strategies discussed here, you can unlock new levels of functionality and create applications that are both efficient and secure. Remember to prioritize security best practices and thoroughly test your implementation to ensure a smooth and reliable user experience.
So, go forth and build something amazing!
Key Questions Answered
What are the potential performance implications of external key handling?
External key handling can introduce some performance overhead, especially if implemented poorly. Efficient code and optimized system calls are crucial to minimize this impact. Careful consideration of the chosen method and its resource consumption is key.
How do I handle conflicts between multiple applications trying to capture the same keystrokes?
Prioritizing key handling is essential. Operating systems typically have mechanisms for managing such conflicts (like focus management). You might need to implement a system to check if another application already registered for a particular key combination before registering your own handler.
What programming languages are best suited for external key handling?
Languages like C++, Python (with appropriate libraries), and others offering low-level system access are well-suited. The best choice depends on your application’s needs and your familiarity with the language.