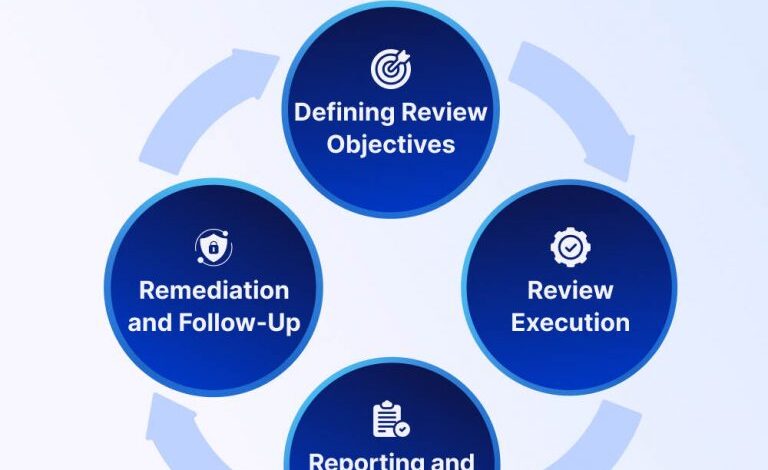
How to Deliver Secure Code More Frequently
How to deliver secure code more frequently? It’s the question on every developer’s mind, especially in today’s fast-paced software development world. We’re constantly battling deadlines, striving for faster releases, but security can’t be an afterthought. This post dives into practical strategies to integrate security seamlessly into your workflow, allowing you to ship secure code faster without compromising quality.
We’ll explore techniques ranging from shifting security left in the development lifecycle to leveraging automated testing and DevSecOps principles.
This isn’t just about ticking boxes; it’s about building a culture of security. We’ll look at how to empower developers with the knowledge and tools to write secure code from the start, streamlining processes, and fostering collaboration between development and security teams. Get ready to revolutionize your approach to secure software delivery!
Shifting Left
The key to delivering secure code more frequently isn’t just about faster testing; it’s about fundamentally changing how we approach security. Instead of treating it as an afterthought, bolted on at the end of the development process, we need to integrate security considerations from the very beginning – a concept known as “shifting left.” This proactive approach significantly reduces vulnerabilities, lowers remediation costs, and ultimately enables faster, more secure releases.Shifting left means embedding security into every stage of the software development lifecycle (SDLC), starting with the initial design phase.
This prevents security issues from becoming deeply ingrained in the codebase, making them far more expensive and time-consuming to fix later. By proactively identifying and addressing potential risks early, we can significantly improve the overall security posture of our applications.
Security Risk Assessments During Planning
Security risk assessments should be conducted early in the project planning stages, even before the first line of code is written. This involves identifying potential threats and vulnerabilities, analyzing their likelihood and impact, and prioritizing mitigation strategies. For example, a risk assessment for a new e-commerce platform might identify risks associated with data breaches, insecure payment processing, and denial-of-service attacks.
This analysis would then inform decisions about security architecture, technologies, and processes. The output of this assessment should be a prioritized list of security controls to be implemented throughout the project.
Integrating Security Testing into Agile Sprints
In an agile development environment, security testing needs to be integrated into each sprint. This can be achieved by incorporating security tasks into the sprint backlog, alongside development and testing tasks. For instance, a sprint might include tasks such as conducting static code analysis, performing dynamic application security testing (DAST), and reviewing security configurations. This iterative approach ensures that security vulnerabilities are identified and addressed early and frequently, preventing them from accumulating and becoming major problems later in the development cycle.
This approach allows for continuous feedback and improvement, enabling the team to adapt and refine their security practices over time.
Security Considerations Checklist for Code Reviews
A crucial element of shifting left is incorporating security into code reviews. Developers should be equipped with a checklist to guide their review process. This checklist should cover aspects such as:
- Input validation: Are all inputs properly validated to prevent injection attacks (SQL injection, cross-site scripting)?
- Authentication and authorization: Are authentication mechanisms secure and are access controls properly implemented?
- Session management: Are session management practices secure, preventing session hijacking?
- Error handling: Does the application handle errors securely, preventing the disclosure of sensitive information?
- Data protection: Is sensitive data properly encrypted both in transit and at rest?
- Third-party libraries: Are all third-party libraries up-to-date and free from known vulnerabilities?
- Logging and monitoring: Does the application provide adequate logging and monitoring capabilities to detect and respond to security incidents?
By consistently applying this checklist during code reviews, developers can proactively identify and address security vulnerabilities before they reach production. This process significantly improves the overall security of the application and reduces the risk of exploitation.
Secure Coding Practices and Techniques
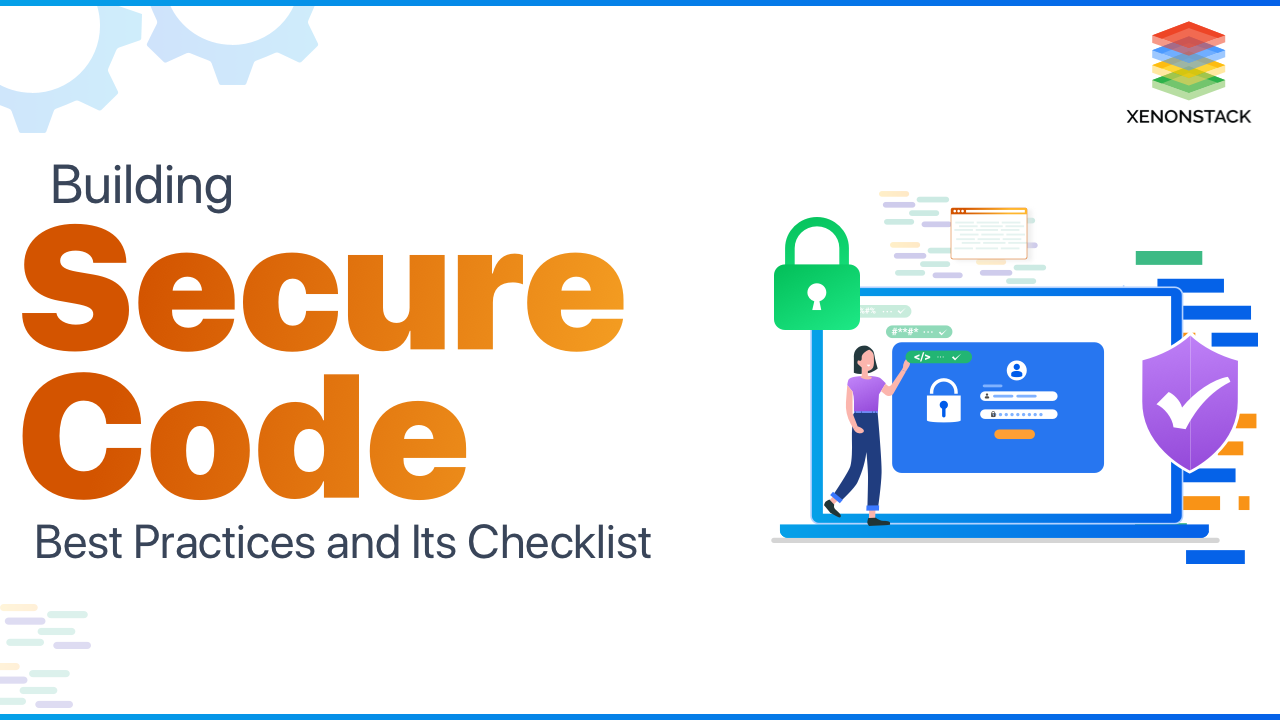
Building secure applications isn’t just about adding security features at the end; it’s about weaving security into the very fabric of your code from the start. This involves adopting secure coding practices and techniques throughout the development lifecycle. This section dives into essential guidelines, patterns, and methods to help you write more secure code.
Secure Coding Guidelines for Common Vulnerabilities
Secure coding necessitates a proactive approach to mitigating common vulnerabilities. Ignoring these risks can lead to significant security breaches and data loss. The following guidelines address some of the most prevalent threats.
Vulnerability | Description | Secure Coding Guideline | Example (Python) |
---|---|---|---|
SQL Injection | Malicious SQL code injected into input fields to manipulate database queries. | Use parameterized queries or prepared statements to prevent direct SQL string concatenation. | cursor.execute("SELECT
|
Cross-Site Scripting (XSS) | Injection of malicious scripts into websites viewed by other users. | Encode user-supplied data before displaying it on a webpage. Use appropriate escaping mechanisms for the target context (HTML, JavaScript, etc.). | print(html.escape(user_input)) |
Cross-Site Request Forgery (CSRF) | Tricking a user into performing unwanted actions on a web application in which they’re currently authenticated. | Use CSRF tokens to verify that requests originate from the legitimate user’s browser. | (Implementation requires a framework-specific approach; generally involves generating a unique token and verifying it on subsequent requests.) |
Authentication Bypass | Exploiting weaknesses in the authentication process to gain unauthorized access. | Implement strong password policies, use multi-factor authentication (MFA), and regularly audit authentication mechanisms. | (Implementation is highly dependent on the chosen authentication framework.) |
Insecure Direct Object References (IDOR) | Accessing resources directly through URLs or other means without proper authorization. | Always validate user permissions before granting access to resources. | (Implementation requires careful authorization checks before accessing any data based on user ID or other identifiers.) |
Secure Coding Patterns
Adopting secure coding patterns promotes consistency and reduces the likelihood of introducing vulnerabilities. These patterns offer reusable solutions to common security challenges.
Examples vary widely based on the programming language and framework. For instance, in Java, using Spring Security provides a robust framework for authentication and authorization. In Python, libraries like `bcrypt` for password hashing and `SQLAlchemy` with parameterized queries contribute to secure coding.
Authentication and Authorization Methods
Authentication verifies the identity of a user, while authorization determines what actions a user is permitted to perform. Different methods offer varying levels of security and complexity.
Method | Description | Strengths | Weaknesses |
---|---|---|---|
Password-Based Authentication | Verifying user identity using a username and password. | Simple to implement. | Vulnerable to brute-force attacks and credential stuffing if passwords are weak or stored insecurely. |
Multi-Factor Authentication (MFA) | Requiring multiple forms of authentication (e.g., password and one-time code). | Significantly enhances security. | Can be more inconvenient for users. |
OAuth 2.0 | Delegating authentication to a third-party provider (e.g., Google, Facebook). | Leverages existing user accounts and simplifies the authentication process. | Relies on the security of the third-party provider. |
API Keys | Using unique keys to authenticate API requests. | Suitable for machine-to-machine communication. | Requires careful management to prevent unauthorized access. |
Input Validation and Sanitization
Input validation and sanitization are crucial steps in preventing many security vulnerabilities. Validation checks if the input conforms to expected formats and constraints, while sanitization removes or neutralizes potentially harmful characters.
Thorough input validation is paramount. Failing to validate input opens the door to various attacks, including SQL injection, XSS, and command injection. Sanitization ensures that even if validation fails, the harmful elements are neutralized before being processed.
Automated Security Testing and Tools
Automating security testing is crucial for shifting security left and achieving faster, more secure software delivery. By integrating automated checks into your CI/CD pipeline, you can catch vulnerabilities early, reducing the cost and effort of fixing them later. This section will explore common tools and techniques for automated security testing, focusing on static and dynamic analysis.
Automated security testing encompasses various approaches, each with its own strengths and weaknesses. The choice of tools and techniques will depend heavily on the specific project, its technology stack, and the level of security risk tolerance.
Static Application Security Testing (SAST) Tools
SAST tools analyze the source code of an application without actually executing it. They examine the code for potential security vulnerabilities based on pre-defined rules and patterns. Popular SAST tools include SonarQube, Checkmarx, and Coverity. These tools excel at identifying vulnerabilities like SQL injection, cross-site scripting (XSS), and buffer overflows, even before the code is compiled or deployed.
Advantages of SAST include early detection of vulnerabilities, reduced costs associated with fixing vulnerabilities later in the development lifecycle, and improved code quality. Disadvantages include high false positive rates, the inability to detect runtime vulnerabilities, and the need for deep understanding of the application’s codebase for effective configuration and interpretation of results.
Dynamic Application Security Testing (DAST) Tools, How to deliver secure code more frequently
Unlike SAST, DAST tools analyze the running application by simulating attacks. They test the application’s response to various inputs and identify vulnerabilities in its behavior. Examples of DAST tools include OWASP ZAP, Burp Suite, and Acunetix. These tools are particularly effective at finding runtime vulnerabilities such as insecure authentication mechanisms, cross-site request forgery (CSRF), and insecure session management.
DAST offers the advantage of identifying runtime vulnerabilities that SAST cannot detect. It also provides a more realistic assessment of the application’s security posture. However, DAST tools can be slow, require a running application, and may produce a large number of false positives, needing careful analysis of reports.
Integrating SAST/DAST into a CI/CD Pipeline
Integrating SAST and DAST tools into a CI/CD pipeline automates security testing as part of the build and deployment process. This ensures that security is not an afterthought but a core component of the development lifecycle. The integration process generally involves configuring the chosen tools to run automatically at specific stages of the pipeline (e.g., after the code is built or deployed to a testing environment).
The results of the security scans are then analyzed, and the pipeline can be configured to fail if critical vulnerabilities are detected.
This automated approach ensures consistent security testing across all builds and deployments, helping prevent vulnerable code from reaching production. Successful integration requires careful planning and configuration, including setting appropriate thresholds for acceptable vulnerability levels and handling false positives effectively. Many CI/CD platforms like Jenkins, GitLab CI, and Azure DevOps offer plugins or integrations to simplify the process.
Setting up Automated Security Testing: A Step-by-Step Guide
Let’s illustrate a simplified example using SonarQube for SAST and OWASP ZAP for DAST within a hypothetical Java project using a Jenkins pipeline. This example is for illustrative purposes and may need adjustments based on your specific tools and environment.
- Project Setup: Create a simple Java project with some intentionally vulnerable code (e.g., a vulnerable servlet with SQL injection).
- SonarQube Setup: Install and configure SonarQube. Create a SonarQube project and obtain the necessary tokens.
- OWASP ZAP Setup: Install and configure OWASP ZAP. You might need to set up a proxy for ZAP to intercept traffic to your application.
- Jenkins Pipeline Configuration: Create a Jenkins pipeline job. This job should include stages for building the project, running SonarQube analysis, deploying the application to a testing environment, running OWASP ZAP scans, and reporting the results. The pipeline should fail if critical vulnerabilities are detected.
- SonarQube Integration: Use the SonarQube Scanner for Jenkins to integrate SonarQube into your pipeline. The scanner will analyze your code and report vulnerabilities.
- OWASP ZAP Integration: Use a ZAP plugin or script to integrate OWASP ZAP into your pipeline. The plugin will launch ZAP, configure it to scan your deployed application, and generate a report.
- Result Analysis and Reporting: The pipeline should consolidate the results from SonarQube and OWASP ZAP, providing a comprehensive security report. This report should be easily accessible to developers and security teams.
Remember, this is a simplified illustration. Real-world implementations might involve more complex configurations, integration with other security tools, and sophisticated vulnerability management systems.
Code Reviews and Security Audits
Integrating security into the code review process is crucial for delivering secure code more frequently. Effective code reviews, combined with regular security audits, act as a powerful safety net, catching vulnerabilities before they reach production and minimizing the risk of costly breaches. This process not only improves the overall security posture of your applications but also fosters a culture of security awareness within the development team.
Effective Strategies for Conducting Thorough Security Code Reviews
Thorough security code reviews require a structured approach. Reviewers should possess a solid understanding of common vulnerabilities and coding best practices. Using a checklist, like the one provided below, ensures consistency and completeness. Furthermore, pairing junior developers with senior developers or security experts during reviews is beneficial for knowledge transfer and identifying potential issues. The review process should also consider the context of the code within the larger application architecture, examining potential interactions and dependencies that could introduce vulnerabilities.
Finally, leveraging static and dynamic analysis tools alongside manual reviews enhances the effectiveness of the process.
Security Audit Checklist Template
A comprehensive security audit checklist should cover various aspects of application security. The following template provides a starting point, and specific items should be tailored based on the application’s context and criticality.
Category | Item | Pass/Fail | Notes |
---|---|---|---|
Authentication & Authorization | Secure password storage | ||
Proper authorization checks | |||
Input Validation | Sanitization of all user inputs | ||
Protection against injection attacks (SQL, XSS, etc.) | |||
Data Protection | Encryption of sensitive data at rest and in transit | ||
Data loss prevention mechanisms | |||
Session Management | Secure session handling | ||
Protection against session hijacking | |||
Error Handling | Secure error handling to prevent information leakage | ||
Prevention of exception handling vulnerabilities | |||
Third-Party Libraries | Regular updates and security checks for external libraries | ||
Deployment | Secure deployment practices |
Best Practices for Providing Constructive Feedback During Security Code Reviews
Constructive feedback is key to a successful security code review. Instead of simply pointing out flaws, reviewers should explain the vulnerabilities, their potential impact, and suggest concrete remediation steps. Using clear and specific language, avoiding accusatory tones, and focusing on the code rather than the developer fosters a collaborative environment. For example, instead of saying “This code is insecure,” a more constructive approach would be “This code is vulnerable to SQL injection because user input is not properly sanitized.
Consider using parameterized queries or input validation techniques to mitigate this risk.” Documenting findings clearly and providing references to relevant security standards and guidelines further enhances the feedback’s value.
Role of Security Experts in Code Review Processes
Security experts play a vital role in code review processes. Their specialized knowledge of security vulnerabilities, attack vectors, and mitigation techniques ensures a more comprehensive and effective review. They can identify subtle vulnerabilities that might be missed by developers, provide guidance on secure coding practices, and help establish a strong security culture within the development team. Their involvement in the review process ensures that security is not an afterthought but an integral part of the software development lifecycle.
Their expertise also ensures that the organization is complying with relevant security standards and regulations.
Infrastructure as Code (IaC) and Security: How To Deliver Secure Code More Frequently
Infrastructure as Code (IaC) is a revolutionary approach to managing and provisioning infrastructure. Instead of manually configuring servers, networks, and other resources, IaC uses code to define and automate these processes. This not only speeds up deployments but also significantly enhances security by promoting consistency, repeatability, and auditable configurations. By treating infrastructure as code, we can apply the same rigorous security practices we use for application code, resulting in a more secure and robust system.IaC principles significantly enhance the security of the development infrastructure by automating the creation and management of resources, reducing the risk of human error and misconfigurations.
This automated approach ensures consistent application of security policies across all environments, from development to production. Furthermore, version control systems allow for tracking changes, enabling rollback capabilities in case of security breaches or unintended configuration drifts. This level of control and traceability is crucial for maintaining a secure infrastructure.
Securing IaC Templates and Configurations
Best practices for securing IaC templates and configurations focus on minimizing attack surfaces and enforcing security policies. This includes using principle of least privilege, regularly updating dependencies, and employing strong authentication and authorization mechanisms. Sensitive information, such as passwords and API keys, should never be hardcoded directly into templates. Instead, utilize secure secrets management solutions integrated with your IaC workflow.
Regularly scanning templates for vulnerabilities using static analysis tools is also critical. This proactive approach helps identify and remediate potential security risks before they can be exploited.
Designing Secure IaC Templates for Common Cloud Platforms
Secure IaC templates for AWS, Azure, and GCP share many common principles but differ in their specific implementation details. For example, in AWS, using IAM roles instead of hardcoded access keys is paramount. This limits the permissions granted to instances and other resources, reducing the impact of potential compromises. In Azure, leveraging Azure Role-Based Access Control (RBAC) achieves similar results.
In GCP, using service accounts with carefully defined permissions is crucial. Across all platforms, network security groups (NSGs) or security groups should be implemented to control inbound and outbound traffic, minimizing the attack surface of your infrastructure. Furthermore, regularly reviewing and updating security policies in your IaC templates is essential to address emerging threats and vulnerabilities.
Implementing Security Policies and Controls Using IaC
Implementing security policies and controls within IaC involves leveraging built-in features of the cloud platforms and incorporating security best practices directly into the code. For instance, enabling encryption at rest and in transit is critical for protecting sensitive data. This can be achieved through IaC by configuring encryption for storage services (like S3 buckets in AWS, Blob storage in Azure, or Cloud Storage in GCP) and utilizing VPNs or other secure network configurations.
Implementing intrusion detection and prevention systems, logging and monitoring capabilities, and automated security patching should also be defined and managed through IaC to ensure consistency and automation. For example, an IaC script could automatically deploy and configure a security information and event management (SIEM) system to collect and analyze security logs from various infrastructure components. This integrated approach ensures that security is not an afterthought but a fundamental part of the infrastructure deployment process.
DevSecOps Implementation and Best Practices
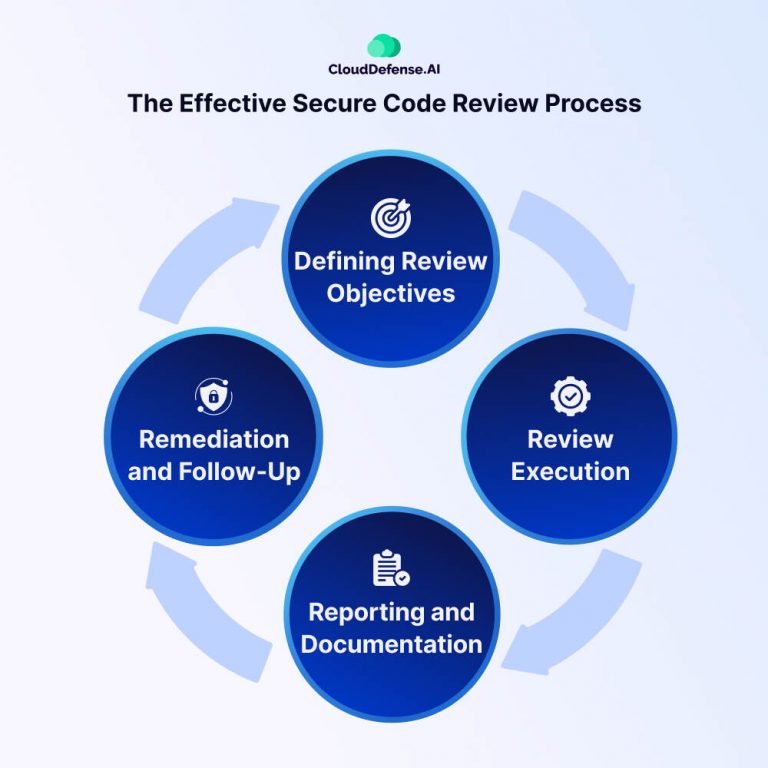
DevSecOps represents a significant evolution in software development, integrating security practices throughout the entire software delivery lifecycle. This approach isn’t merely about adding security as an afterthought; it’s about fundamentally shifting the mindset to proactively embed security into every stage, from initial planning to deployment and beyond. This holistic approach dramatically improves both the speed and security of software delivery, minimizing vulnerabilities and accelerating time-to-market.The core principle of DevSecOps is to automate security testing and integrate it seamlessly into the CI/CD pipeline.
This eliminates the traditional security bottleneck often experienced in traditional development methodologies. By automating security checks early and often, developers can identify and resolve vulnerabilities quickly, preventing them from propagating through the development process. This proactive approach reduces the overall cost and effort required to secure software.
DevSecOps Team Roles and Responsibilities
Effective DevSecOps relies on clear roles and responsibilities across development, security, and operations teams. Development teams are responsible for writing secure code, utilizing automated security tools, and participating in code reviews. Security teams focus on defining security policies, providing security expertise, and integrating security tools into the CI/CD pipeline. Operations teams ensure the secure deployment and maintenance of applications, managing infrastructure security and monitoring for vulnerabilities.
Collaboration and shared responsibility are key; a siloed approach undermines the effectiveness of DevSecOps.
DevSecOps Implementation Plan for a Medium-Sized Organization
A phased approach is recommended for implementing DevSecOps in a medium-sized organization. Phase 1 focuses on establishing a security baseline by conducting a security assessment of existing systems and applications. This includes identifying existing vulnerabilities and establishing security policies and standards. Phase 2 involves integrating automated security testing tools into the CI/CD pipeline. This could start with static application security testing (SAST) and dynamic application security testing (DAST) tools, gradually adding other security checks as the team gains experience.
Phase 3 concentrates on training and education, equipping development and operations teams with the necessary skills and knowledge to effectively implement DevSecOps. Continuous improvement and monitoring are crucial throughout the entire process. Regular security assessments and audits should be conducted to identify areas for improvement and ensure the effectiveness of implemented security measures.
Examples of Successful DevSecOps Implementations and Lessons Learned
Many organizations have successfully implemented DevSecOps, significantly improving their security posture and software delivery speed. For example, a financial institution that implemented DevSecOps saw a 50% reduction in security vulnerabilities and a 20% increase in deployment frequency. This success was attributed to a strong focus on automation, collaboration between teams, and a culture of security. However, challenges remain.
One common lesson learned is the importance of strong leadership support and a commitment to cultural change. Organizations need to foster a culture where security is everyone’s responsibility, not just the security team’s. Another key takeaway is the need for iterative implementation. DevSecOps is a journey, not a destination, and organizations should focus on continuous improvement and adaptation.
Finally, effective communication and collaboration are essential for success. Teams need to be able to effectively communicate security risks and vulnerabilities and collaborate to resolve them quickly.
Managing Security Vulnerabilities and Remediation
Finding and fixing security flaws is a crucial part of building secure software. It’s not a one-time event; it’s an ongoing process that needs to be integrated into every stage of the software development lifecycle (SDLC). Effective vulnerability management significantly reduces the risk of exploitation and protects your users and your organization.A structured approach to vulnerability management involves several key steps, from initial identification through to final resolution and verification.
This requires a combination of automated tools, manual processes, and a well-defined workflow to ensure that vulnerabilities are addressed promptly and efficiently.
Vulnerability Identification and Prioritization
Identifying vulnerabilities requires a multi-faceted approach. Static and dynamic application security testing (SAST and DAST) tools automatically scan code and running applications for common weaknesses. Penetration testing, performed by security experts, simulates real-world attacks to uncover vulnerabilities that automated tools might miss. Regular security audits provide a comprehensive overview of the security posture of the system. Prioritization is often based on factors such as severity (critical, high, medium, low), exploitability (how easy it is to exploit), and impact (the potential damage caused).
A common vulnerability scoring system like CVSS (Common Vulnerability Scoring System) helps quantify these factors and allows for objective prioritization.
Vulnerability Tracking and Management
Tracking and managing vulnerabilities throughout the SDLC requires a dedicated system, often a vulnerability management platform. This system allows for the central logging and tracking of all discovered vulnerabilities, assigning them to responsible teams, monitoring their remediation status, and generating reports. The platform should integrate with other development tools to provide a seamless workflow. For instance, a vulnerability found during SAST could automatically create a task in the project management system, assigned to the relevant developer.
Regular reporting on the status of vulnerabilities provides valuable insights into the effectiveness of the security program.
Vulnerability Disclosure and Communication
Responsible disclosure of vulnerabilities is crucial for maintaining trust and protecting users. This involves establishing a clear process for reporting vulnerabilities, whether discovered internally or by external security researchers. A well-defined vulnerability disclosure program Artikels how vulnerabilities should be reported, how they will be investigated, and how affected users will be notified. Transparency and timely communication are key to building a positive reputation and fostering collaboration within the security community.
For instance, a company might have a dedicated security email address or a bug bounty program to encourage ethical hackers to report vulnerabilities.
Vulnerability Management Process
Effective vulnerability management requires a structured process. Here’s a breakdown of the key steps:
- Identify Vulnerabilities: Employ automated tools (SAST/DAST), penetration testing, and manual code reviews to uncover vulnerabilities.
- Assess and Prioritize: Evaluate the severity, exploitability, and impact of each vulnerability using a scoring system like CVSS.
- Remediate Vulnerabilities: Assign vulnerabilities to developers and track their progress through the remediation process.
- Verify Remediation: After a fix is implemented, retest to ensure the vulnerability has been successfully addressed.
- Document and Report: Maintain detailed records of all vulnerabilities, their remediation status, and any relevant communication.
- Continuous Monitoring: Regularly scan for new vulnerabilities and monitor for any signs of exploitation.
Security Awareness Training for Developers
Developing secure code is paramount, but technical skills alone aren’t enough. Developers need a strong understanding of security threats and vulnerabilities to effectively build robust applications. A comprehensive security awareness training program bridges this gap, empowering developers to proactively integrate security into their daily workflow. This program goes beyond simply delivering information; it aims to cultivate a security-conscious mindset within the development team.Security awareness training for developers should be a continuous process, not a one-time event.
It needs to be engaging, relevant, and tailored to the specific technologies and challenges faced by the development team. Regular updates and reinforcement are crucial to maintain awareness of emerging threats and best practices. This approach helps foster a culture of security within the organization, leading to more secure and reliable software.
A Model Security Awareness Training Program
This program comprises several interactive modules delivered through various methods, including online courses, workshops, and hands-on exercises. The goal is to create a dynamic learning experience that keeps developers engaged and encourages active participation. The curriculum should cover a range of topics, from fundamental security concepts to specific coding vulnerabilities and mitigation techniques.
Interactive Training Modules for Developers
Several interactive modules can be implemented. One module could be a series of interactive coding challenges where developers are presented with vulnerable code snippets and tasked with identifying and fixing the security flaws. Another module could use a simulated phishing attack to demonstrate the real-world consequences of social engineering. A third module could involve a capture-the-flag (CTF) style exercise, where developers work collaboratively to solve security-related puzzles.
These interactive approaches significantly improve knowledge retention compared to passive learning methods. For instance, a module simulating a SQL injection attack, showing how malicious input can compromise a database, followed by a hands-on exercise fixing the vulnerability in sample code, would be far more effective than a simple lecture.
Assessing the Effectiveness of Security Awareness Training
Measuring the success of security awareness training is crucial. This can be done through various methods, including pre- and post-training assessments, knowledge checks integrated into the training modules, and tracking the number of security vulnerabilities reported and fixed by developers after the training. Analyzing the results of these assessments provides valuable insights into the effectiveness of the training program and areas needing improvement.
For example, a significant decrease in the number of vulnerabilities found in code reviews post-training would indicate a positive impact. Conversely, consistent issues in specific areas highlight the need for focused retraining or curriculum adjustments.
Incorporating Security Awareness Training into Onboarding
New developers should receive security awareness training as part of their onboarding process. This ensures that they understand security best practices from day one. The training can be integrated into the existing onboarding program, making it a seamless part of the new hire experience. This early exposure to security concepts helps build a strong security foundation and sets the tone for secure coding throughout their employment.
Including a security awareness module within the initial onboarding materials and assigning relevant online training modules in the first week can ensure new hires start their journey with a solid understanding of security principles.
Final Thoughts
Ultimately, delivering secure code more frequently isn’t about sacrificing speed for safety; it’s about finding the perfect balance. By integrating security into every stage of the development lifecycle, automating testing, and fostering a culture of shared responsibility, you can significantly reduce vulnerabilities and accelerate your release cycles. Remember, it’s a journey, not a destination – continuously refining your processes and learning from each iteration will be key to your success.
So, embrace the challenge, implement these strategies, and watch your secure code deployments soar!
Expert Answers
What are the biggest security risks in modern software development?
Common risks include SQL injection, cross-site scripting (XSS), cross-site request forgery (CSRF), insecure authentication, and improper input validation. These vulnerabilities often stem from insecure coding practices and inadequate testing.
How can I get buy-in from my team on DevSecOps?
Start by demonstrating the benefits – faster releases, reduced costs from fixing vulnerabilities later, and improved team morale. Focus on making the process as streamlined and integrated as possible to minimize disruption to their workflow. Highlight successes and address concerns proactively.
What if I don’t have a dedicated security team?
Many resources are available to help. Start with online training courses for your developers, utilize automated security testing tools, and engage external security consultants for periodic audits and reviews.
How often should I conduct security audits?
The frequency depends on your risk tolerance and the complexity of your application. Regular audits (e.g., quarterly or annually) are generally recommended, along with continuous monitoring and vulnerability scanning.