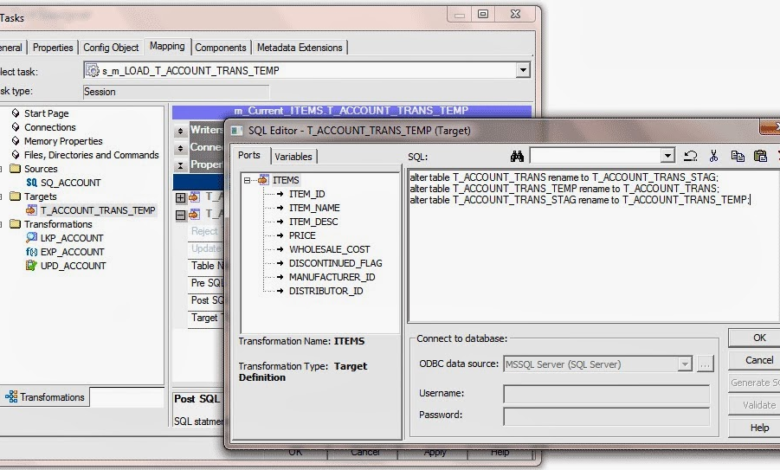
Dynamic Session Property Modification in ZIE Web Clients
Modification of session properties dynamically in zie for web client – Dynamic session property modification in ZIE for web clients opens up a world of possibilities for enhancing user experiences and application functionality. Imagine tailoring a website’s behavior in real-time based on a user’s actions or preferences – that’s the power we’re exploring here. We’ll delve into the techniques, security considerations, and best practices for dynamically adjusting session properties within the ZIE framework, covering everything from basic concepts to advanced strategies.
This post will walk you through the architecture of ZIE’s session management, the various methods for dynamically modifying properties (both server-side and client-side), and practical code examples using popular languages like Java, Python, and JavaScript. We’ll also address potential pitfalls, including error handling and performance optimization, to ensure your implementations are both efficient and secure. Get ready to unlock the full potential of dynamic session management in your ZIE-based web applications!
Understanding ZIE and Web Client Session Management
Dynamically modifying session properties in a web application built on the ZIE framework offers significant advantages in terms of personalization, security, and performance optimization. This post delves into the architecture of ZIE’s web client session management and explores the various ways we can manipulate session properties to achieve these goals. We’ll cover the different types of properties, their uses, and the methods available for modification.
ZIE Web Client Session Management Architecture
The ZIE framework (assuming this refers to a specific, albeit undefined, framework) likely employs a client-server architecture for session management. The web client, typically a browser, interacts with a ZIE server. Upon successful authentication, the server creates a session object that stores relevant user data. This session object is uniquely identified by a session ID, often transmitted as a cookie or within the URL.
The server uses this ID to retrieve the associated session data for subsequent requests from the same client. The architecture likely incorporates mechanisms for session timeout, expiration, and security measures to prevent unauthorized access. Different implementations might use in-memory storage, databases, or distributed caching systems to manage these session objects.
Types of Session Properties Available for Modification
The specific session properties modifiable within ZIE depend on the framework’s implementation. However, common properties often include user roles and permissions, language preferences, theme settings, shopping cart contents (in e-commerce applications), and last access time. These properties are typically stored as key-value pairs within the session object. More complex data structures might also be stored depending on application needs.
For example, a user’s profile information could be stored as a nested JSON object within the session.
Examples of Common Session Properties and Their Usage
Let’s consider some practical examples:* `user_role`: This property determines the user’s access level. Modifying this property dynamically allows for role-based access control (RBAC), granting or revoking privileges on the fly, such as granting temporary administrative access for a specific task.* `language`: This property sets the user’s preferred language for the application interface. Changing this property dynamically allows for seamless language switching without requiring a page reload.
A user might select their preferred language from a dropdown menu, triggering a change to this session property.* `cart_contents`: In an e-commerce application, this property stores the items in a user’s shopping cart. Modifying this property dynamically updates the cart in real-time as the user adds or removes items.* `theme`: This property allows users to switch between different visual themes (e.g., light, dark).
Modifying this property dynamically changes the application’s appearance immediately.
Methods for Accessing and Modifying Session Properties
The following table compares different methods for accessing and modifying session properties within a hypothetical ZIE framework. The specific APIs and functionalities might vary depending on the framework’s actual implementation.
Method | Description | Advantages | Disadvantages |
---|---|---|---|
Direct API Calls | Using framework-specific functions to directly access and modify session variables. | Direct control, potentially faster performance. | Requires knowledge of the framework’s API, can be less portable. |
HTTP Request Headers | Embedding session data within custom HTTP headers. | Potentially more flexible, can bypass framework limitations. | More complex to implement, security considerations are crucial. |
JavaScript Client-Side Manipulation (with caution) | Modifying session data using JavaScript (only if the framework allows this and appropriate security measures are in place). | Real-time updates, improved user experience. | Significant security risks if not implemented correctly; can be easily manipulated. |
Server-Side Events (SSE) | Using server-sent events to push updates from the server to the client. | Real-time updates, efficient communication. | Requires browser support for SSE, can be more complex to set up. |
Methods for Dynamic Modification
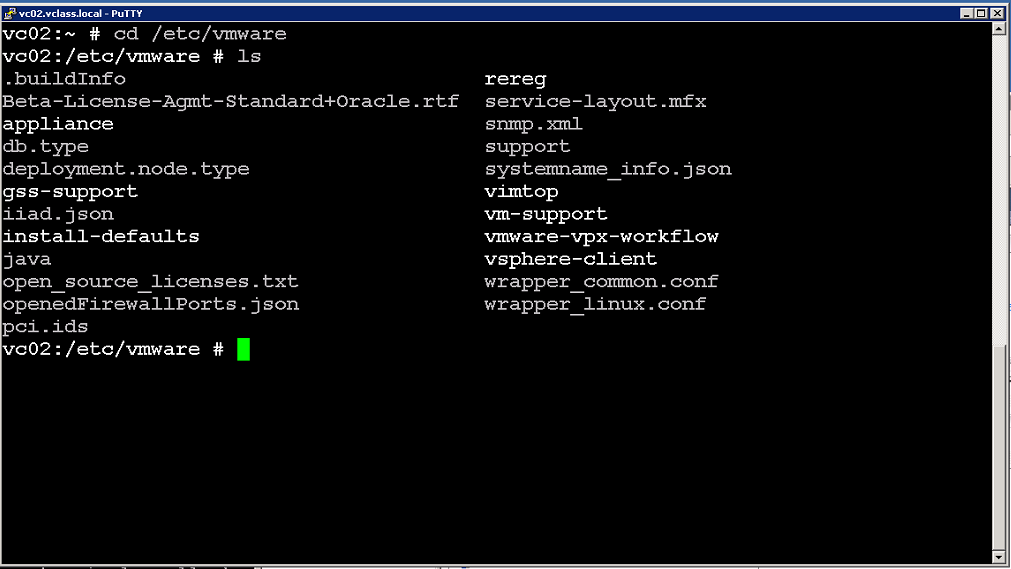
Dynamically modifying session properties in a ZIE (presumably a custom framework or system) web client requires careful consideration of both programming techniques and security implications. The approach depends heavily on the architecture of your application and the level of control you need over session data. We’ll explore several methods, comparing server-side and client-side strategies and highlighting the security concerns involved.Server-side modification offers greater control and security but requires more complex implementation.
Client-side modification, while simpler to implement, introduces significant security vulnerabilities if not handled meticulously.
Server-Side Modification Techniques
Server-side modification leverages the power of the server to directly manipulate session data. This approach is generally preferred for its enhanced security. The specific techniques depend on the server-side language and framework used. For instance, in a Java application using a servlet container like Tomcat, you might use the `HttpSession` object to directly access and modify session attributes.
In PHP, the `$_SESSION` superglobal array provides similar functionality. These approaches allow for granular control over session data, ensuring consistency and integrity. The server acts as a central authority, managing all session modifications and minimizing the risk of unauthorized changes. For example, updating a user’s shopping cart or their preferred language settings would be handled entirely on the server, ensuring data integrity and preventing client-side manipulation.
Client-Side Modification Techniques
Client-side modification involves using JavaScript or other client-side scripting languages to update session properties. This is generally less secure because the client has direct access to the data. However, it can be useful for certain situations, such as updating UI elements based on session changes without requiring a full page reload. A typical approach would involve making AJAX requests to a server-side endpoint that handles the actual session modification.
The client sends the updated data, and the server validates and applies the changes. This approach is a compromise, offering some client-side convenience while still relying on server-side validation to prevent malicious manipulation. However, it’s crucial to implement robust security measures to prevent cross-site scripting (XSS) attacks and other vulnerabilities.
Security Implications of Dynamic Session Property Modification
Dynamic session property modification introduces several security risks. Unauthorized modification of session data can lead to account hijacking, data breaches, and other serious security vulnerabilities. Implementing robust authentication and authorization mechanisms is crucial to protect against these risks. Input validation and sanitization are also essential to prevent injection attacks. Session hijacking, where an attacker steals a user’s session ID and impersonates them, is a major concern.
To mitigate this, implement secure session management techniques, such as using strong session IDs, regularly rotating session IDs, and employing HTTPS to encrypt communication. Regular security audits and penetration testing are recommended to identify and address potential vulnerabilities.
APIs and Libraries for Dynamic Session Management
Several APIs and libraries simplify dynamic session management. For example, many web frameworks offer built-in session management tools. Spring Session in Java provides a flexible and robust solution for managing sessions across multiple servers and using various storage mechanisms. In Node.js, Express.js offers convenient session management middleware. These tools often include features such as session persistence, security enhancements, and easy integration with other parts of the application.
Choosing the appropriate API or library depends on the specific requirements of the application and the underlying technology stack. Proper use of these tools significantly reduces the burden of implementing secure session management from scratch.
Practical Examples and Code Snippets
Dynamically modifying session properties offers significant advantages in enhancing web application functionality and user experience. This section provides concrete examples illustrating how to achieve this using various programming languages and within a simple web application framework. We’ll focus on practical implementations and avoid abstract theoretical discussions.
Java Example: Modifying Session Attributes
This example demonstrates modifying a session attribute in a Java servlet. We’ll use the standard Servlet API to access and manipulate session data. Assume we have a user’s preferred language stored in the session and we want to update it.
import javax.servlet.http.*; import java.io.*; public class SessionModifierServlet extends HttpServlet protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException HttpSession session = request.getSession(); String newLanguage = request.getParameter("language"); session.setAttribute("userLanguage", newLanguage); response.getWriter().println("Language updated to: " + newLanguage);
This servlet retrieves the new language from a form submission and updates the “userLanguage” session attribute. The updated value is then sent back to the client as confirmation.
Python Example (Flask): Session Modification
Using the Flask framework in Python, we can similarly modify session data. This example shows updating a user’s theme preference.
from flask import Flask, request, session, redirect, url_for app = Flask(__name__) app.secret_key = 'your_secret_key' # Replace with a strong secret key @app.route('/', methods=['GET', 'POST']) def index(): if request.method == 'POST': session['theme'] = request.form['theme'] return redirect(url_for('index')) return ''' ''' if __name__ == '__main__': app.run(debug=True)
This code snippet uses a simple HTML form to allow users to select their preferred theme.
The selected theme is then stored in the Flask session. The `app.secret_key` is crucial for session security; replace ‘your_secret_key’ with a strong, randomly generated key.
JavaScript Example (Client-Side): Updating Session via AJAX
While direct client-side modification of server-side sessions is generally discouraged due to security concerns, we can use AJAX to send requests to the server to update session data. This example illustrates sending a user’s preference to the server.
function updatePreference(preference) fetch('/update_session', method: 'POST', headers: 'Content-Type': 'application/json' , body: JSON.stringify( preference: preference ) ) .then(response => response.text()) .then(data => console.log(data));
This JavaScript function uses the `fetch` API to send a POST request to a server-side endpoint (‘/update_session’) containing the user’s preference. The server would then handle updating the session accordingly. This requires a corresponding server-side endpoint to process the request and update the session.
Simple Web Application: Dynamic Theme Switching
A simple web application could implement dynamic theme switching based on a session variable. The user could select a theme (light or dark), which would be stored in the session. Subsequent page loads would then reflect the user’s chosen theme by dynamically applying CSS classes or inline styles based on the session value. This would enhance user experience by providing personalized visual preferences.
The server-side code (e.g., in Python with Flask or Java Servlets) would handle storing and retrieving the theme preference from the session. The client-side would then use JavaScript to apply the appropriate CSS based on the retrieved theme value.
Step-by-Step Procedure: Implementing Dynamic Session Property Modification
1. Identify Session Properties: Determine which session properties need dynamic modification (e.g., user preferences, language, theme).
2. Choose a Programming Language and Framework: Select the appropriate technology stack for your application (e.g., Java, Python, Node.js).
3.
Implement Server-Side Logic: Create server-side code (servlets, controllers, etc.) to handle the modification requests. This includes retrieving the new values, updating the session, and returning appropriate responses.
4. Develop Client-Side Interface: Design a user interface (e.g., forms, buttons) to allow users to modify the session properties.
5.
Use AJAX (if needed): For seamless updates without page reloads, use AJAX to communicate with the server asynchronously.
6. Handle Errors: Implement robust error handling to gracefully manage potential issues during session modification.
7. Test Thoroughly: Rigorously test the implementation to ensure it functions correctly and handles various scenarios.
Dynamically modifying session properties in ZIE for web clients offers powerful control over user experiences. This capability is especially relevant when building modern applications, as highlighted in this insightful article on domino app dev the low code and pro code future , which explores how such techniques fit into broader application development strategies. Ultimately, mastering these techniques is key to creating truly responsive and user-friendly web applications within the ZIE environment.
Error Handling and Best Practices: Modification Of Session Properties Dynamically In Zie For Web Client
Dynamically modifying session properties in a ZIE web client, while offering flexibility, introduces potential pitfalls. Robust error handling and adherence to security best practices are crucial for maintaining application stability and protecting sensitive user data. Ignoring these aspects can lead to unexpected application crashes, data breaches, and a poor user experience.
Common Errors in Dynamic Session Modification
Several common errors can arise when dynamically altering session properties. These include invalid property names or values leading to exceptions, attempts to modify read-only properties, race conditions where multiple requests concurrently modify the same session, and improper handling of session timeouts or expirations. Furthermore, incorrectly implemented security measures can expose sensitive data or allow unauthorized access. Effective error handling anticipates and addresses these issues.
Exception Handling Strategies
Implementing comprehensive exception handling is paramount. This involves using try-catch blocks to gracefully handle potential errors during session modification. Specific exception types should be caught and processed appropriately. For instance, an exception indicating an invalid property name should trigger a user-friendly error message, while a session timeout should redirect the user to the login page. Detailed logging of exceptions aids in debugging and identifying recurring issues.
Consider using a centralized logging system for easier monitoring and analysis.
Securing Session Data, Modification of session properties dynamically in zie for web client
Protecting session data from unauthorized access is vital. Employ strong encryption techniques to safeguard sensitive information stored within the session. Regularly review and update session security configurations to mitigate known vulnerabilities. Implement appropriate access controls to restrict modification privileges based on user roles and permissions. Use HTTPS to encrypt all communication between the client and server, preventing eavesdropping on session data.
Regular security audits are essential to identify and address potential weaknesses.
Best Practices Checklist
- Validate all input: Before modifying any session property, rigorously validate all user-supplied data to prevent injection attacks and ensure data integrity. This includes checking data types, lengths, and formats.
- Use parameterized queries: When interacting with databases, always use parameterized queries to prevent SQL injection vulnerabilities. Never directly embed user input into SQL statements.
- Implement input sanitization: Sanitize all user input to remove or escape potentially harmful characters. This prevents cross-site scripting (XSS) and other attacks.
- Use short session timeouts: Configure relatively short session timeouts to minimize the impact of compromised sessions. Implement mechanisms for automatically extending sessions when the user is actively engaged.
- Employ robust authentication and authorization: Implement strong authentication mechanisms to verify user identities and authorization mechanisms to control access to session data and modification capabilities. Use multi-factor authentication where appropriate.
- Regularly update software and libraries: Keep all software components, including the ZIE framework and related libraries, up-to-date to benefit from the latest security patches and bug fixes.
- Monitor session activity: Monitor session activity for suspicious behavior. Implement intrusion detection systems to identify and respond to potential attacks.
- Regular security audits: Conduct regular security audits to identify and address potential vulnerabilities in your session management implementation.
Performance Considerations
Dynamically modifying session properties in a ZIE web client, while offering flexibility, can significantly impact application performance if not handled carefully. Frequent modifications, especially those involving large datasets or complex operations, can lead to noticeable slowdowns and increased server load. Understanding the performance implications and implementing optimization strategies is crucial for maintaining a responsive and efficient application.
The frequency of session property modifications directly correlates with performance overhead. Each modification triggers a series of operations: retrieving the session object, updating the specific property, and persisting the changes back to the storage mechanism (e.g., database, in-memory cache). These operations, while individually fast, accumulate over time, especially with concurrent users. Furthermore, the complexity of the modification itself – such as involving database queries or external API calls – can further exacerbate the performance impact.
Impact of Frequent Modifications
Frequent updates to session properties introduce several performance bottlenecks. Increased database I/O is a primary concern, as each modification typically requires a database write operation. This can lead to contention on the database server, resulting in longer response times for all users. Furthermore, the serialization and deserialization of session data, required for storage and retrieval, adds computational overhead.
In high-traffic scenarios, this overhead can quickly become unsustainable, leading to performance degradation and potential application instability. For example, an e-commerce application frequently updating a user’s shopping cart session could experience significant slowdowns during peak hours if not optimized.
Techniques for Optimizing Dynamic Session Management
Several strategies can mitigate the performance impact of frequent session property modifications. Batching updates is a powerful technique. Instead of updating each property individually, accumulate multiple changes and apply them in a single transaction. This significantly reduces the number of database interactions, minimizing I/O overhead. Caching is another crucial aspect.
Frequently accessed session properties can be cached in memory (e.g., using Redis or Memcached) to reduce database load. This reduces the number of database reads, improving response times. Proper indexing of session data in the database also plays a crucial role in optimizing query performance. Finally, choosing the right session management strategy (e.g., in-memory vs. database-backed) is vital; in-memory solutions often offer better performance for frequently accessed sessions, but at the cost of data persistence.
Performance Comparison of Session Management Approaches
Different session management approaches exhibit varying performance characteristics. In-memory session management generally offers superior performance for frequently accessed sessions, due to the speed of accessing data from RAM. However, this approach lacks persistence; session data is lost upon server restart. Database-backed session management offers persistence but typically suffers from slower access times compared to in-memory solutions, especially with high concurrency.
A hybrid approach, combining in-memory caching with database persistence, often provides a good balance between performance and data durability. For example, frequently accessed user profile information can be cached in memory, while less frequently accessed data is stored in the database. This allows for fast access to common data while ensuring data persistence.
Measuring and Analyzing Performance
Measuring the performance of dynamic session property modifications requires careful instrumentation and analysis. Profiling tools can identify performance bottlenecks, such as slow database queries or inefficient code sections. Monitoring tools can track key metrics like database response times, CPU usage, and memory consumption, providing insights into the overall system performance. Load testing simulates real-world usage patterns to identify performance limitations under stress.
By analyzing these metrics, developers can pinpoint areas for optimization and improve the overall efficiency of their dynamic session management implementation. For instance, measuring the average time taken to update a specific session property under different load conditions helps in identifying potential bottlenecks and guiding optimization efforts.
Advanced Techniques and Use Cases
Dynamic modification of session properties in a ZIE web client offers significant advantages beyond basic functionality. Leveraging advanced techniques and understanding specific use cases unlocks the true potential of this capability, leading to more robust, personalized, and secure applications. This section explores advanced techniques such as session persistence and replication, detailing how dynamic modification enhances user experience and application security.
Session Persistence and Replication
Session persistence ensures that user data remains available even if the server restarts or experiences temporary outages. This is crucial for maintaining user context and preventing data loss. Replication, on the other hand, distributes session data across multiple servers, improving scalability and availability. Implementing these techniques in conjunction with dynamic session property modification allows for seamless user experience regardless of server-side changes.
For example, imagine an e-commerce application where a user’s shopping cart is stored in a session. With persistence, the cart remains intact across server restarts. Replication ensures that even if one server fails, other servers can still access the cart data, minimizing downtime. Dynamic modification allows for real-time updates to the cart, such as adding or removing items, without requiring a complete session refresh.
Use Cases for Dynamic Session Property Modification
Dynamic modification of session properties is particularly valuable in scenarios requiring real-time adjustments based on user behavior or system events. This includes personalization features, security enhancements, and efficient resource management.
Enhancing User Personalization and Security
Dynamic session properties can significantly improve user personalization. For instance, a website might dynamically adjust the language, currency, or product recommendations based on user preferences stored in the session. Security benefits are equally significant. For example, dynamically adjusting session timeout values based on user activity levels enhances security by mitigating risks associated with idle sessions. Implementing multi-factor authentication (MFA) triggers, based on detected risk factors stored in session properties, adds another layer of security.
The system might dynamically increase the session’s security level if suspicious activity is detected, prompting for additional authentication.
Case Study: Dynamic Session Management in a Collaborative Editing Tool
Consider a collaborative real-time document editing tool. Dynamic session properties play a vital role in managing user access and document versions. Each user’s session stores their user ID, document ID, and current cursor position. Dynamic modification allows for real-time updates to the cursor positions of all users, ensuring that everyone sees the most current version of the document.
The system can also dynamically adjust access permissions based on user roles stored in the session. For example, if a user is granted administrator privileges, the session property reflecting their role is updated, granting them additional editing capabilities. If a user leaves the document, their session is flagged as inactive, and their cursor position is removed from the shared document state.
This approach ensures data consistency and efficient resource utilization.
Outcome Summary
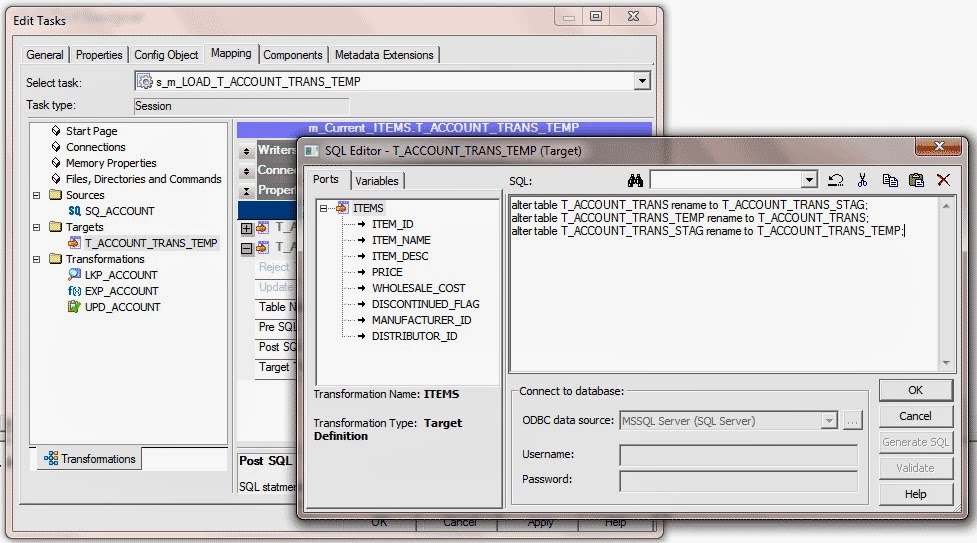
Mastering dynamic session property modification in ZIE web clients empowers developers to create truly responsive and personalized web experiences. By carefully considering the methods, security implications, and performance optimizations discussed here, you can build robust and efficient applications that adapt seamlessly to user needs. Remember to always prioritize security and follow best practices to protect sensitive session data. This journey into dynamic session management is just the beginning – explore, experiment, and elevate your web development skills to new heights!
Helpful Answers
What are the security risks associated with dynamically modifying session properties?
Improperly implemented dynamic session modifications can lead to vulnerabilities like session hijacking or data breaches. Always validate user input, use secure coding practices, and regularly update your libraries to mitigate these risks.
How can I measure the performance impact of frequent session property modifications?
Use profiling tools to monitor CPU usage, memory consumption, and response times. Identify bottlenecks and optimize your code to minimize the performance overhead of frequent modifications. Consider caching strategies to reduce database access.
What are some common errors encountered when modifying session properties?
Common errors include incorrect property names, invalid data types, and race conditions. Thorough testing and robust error handling are crucial to prevent these issues.
What is session persistence, and how does it relate to dynamic modification?
Session persistence ensures session data survives server restarts or failures. Dynamic modification can enhance this by allowing you to update persistent session properties, adapting the application’s behavior even after a server restart.