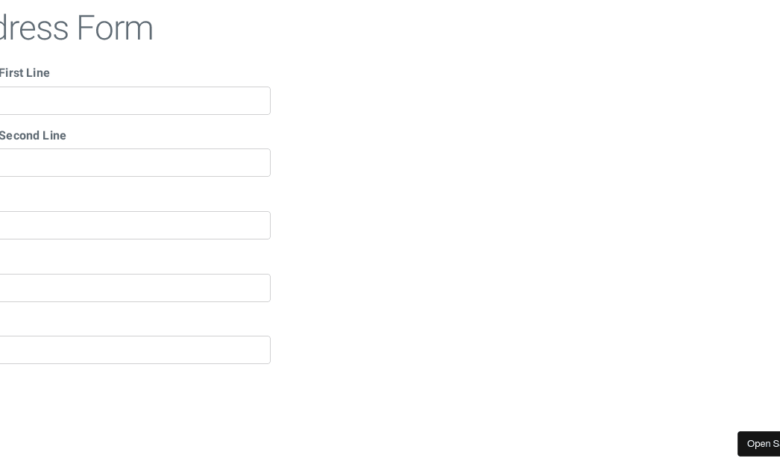
Using Codebase to Set a Custom Location
Using code base to set a custom location – Using codebase to set a custom location opens up a world of possibilities! Imagine building applications that truly understand location, going beyond simple city selection. We’re talking about precise coordinates, integrating with mapping APIs, and even designing clever algorithms to find the nearest pizza place based on your exact location. This journey will cover everything from defining locations in code to visualizing them on a map, and even the crucial security considerations involved.
We’ll explore different ways to represent locations (latitude/longitude, addresses, postal codes), the best data structures to use, and how to seamlessly integrate this data with popular mapping services like Google Maps and Mapbox. We’ll also tackle the challenges of handling errors, updating data efficiently, and building location-based features like proximity searches and targeted advertising. Get ready to dive into the fascinating world of location-based programming!
Defining Custom Location within Code
Defining custom locations within a codebase is crucial for many applications, from mapping and navigation to location-based services and geographical information systems (GIS). The way you represent and manage this location data significantly impacts the efficiency and accuracy of your application. Choosing the right data structure and validation methods is key to building a robust and reliable system.Representing Custom LocationsDifferent applications require different levels of precision and detail when representing a location.
Therefore, several methods exist for encoding this information.
Location Representation Methods
We can represent a custom location using various methods, each with its own strengths and weaknesses. The choice depends heavily on the specific application and the required level of accuracy.
- Latitude/Longitude Coordinates: This is the most common and precise method. Latitude and longitude are expressed in decimal degrees, providing a globally unique identifier for any point on Earth. For example, the coordinates for the Eiffel Tower in Paris might be represented as 48.8584° N, 2.2945° E. This method is ideal for applications requiring high accuracy, such as mapping and navigation systems.
- Address String: This is a less precise but more human-readable method. An address string typically includes street number, street name, city, state/province, and postal code. For example: “123 Main Street, Anytown, CA 91234”. The accuracy depends on the level of detail in the address and the ability of geocoding services to translate it into coordinates.
- Postal Code: This is the least precise method, representing a broad geographical area rather than a specific point. For example, “90210” represents a large area of Beverly Hills, California. It’s suitable for applications where only a general region is needed.
Data Structures for Custom Location Data
Efficiently storing and managing location data requires a well-designed data structure. Several options exist, each with trade-offs in terms of storage space, retrieval speed, and complexity.
- Simple Structures (e.g., Dictionaries/Objects): For simple applications, a dictionary or object can suffice. This approach is straightforward, especially when dealing with only a few locations. For example, a Python dictionary might look like:
'name': 'Eiffel Tower', 'latitude': 48.8584, 'longitude': 2.2945
. However, managing and querying large datasets becomes inefficient with this approach. - Spatial Databases: For applications requiring complex spatial queries and analysis (e.g., finding locations within a certain radius), spatial databases are highly recommended. These databases are optimized for storing and querying geographical data, providing efficient search capabilities. PostGIS (a PostgreSQL extension) and MongoDB are examples of popular spatial databases.
- Custom Classes: Defining a custom class can improve code organization and maintainability, especially when dealing with complex location data that includes additional attributes beyond coordinates (e.g., altitude, address, point of interest type). This allows for better encapsulation and data validation.
Advantages and Disadvantages of Data Structures
The choice of data structure depends on the application’s needs and scale. Simple structures are easy to implement but lack scalability. Spatial databases excel at spatial queries but require more setup and expertise. Custom classes provide better organization but add development overhead.
Custom Location Data Validation
Validating custom location data is crucial to ensure data accuracy and prevent errors. A validation function should check for correct data formats and reasonable values.
- Latitude/Longitude Validation: Latitude values should be between -90 and +90 degrees, and longitude values between -180 and +180 degrees. Additionally, you might want to check if the coordinates fall within a specific region of interest.
- Address String Validation: This is more complex and often relies on external geocoding services to verify if the address exists and obtain its coordinates. Basic checks could include verifying the format of the address components.
- Postal Code Validation: Postal code validation usually involves checking against a database of valid postal codes for a specific country or region.
Integrating Custom Location Data into Existing Systems
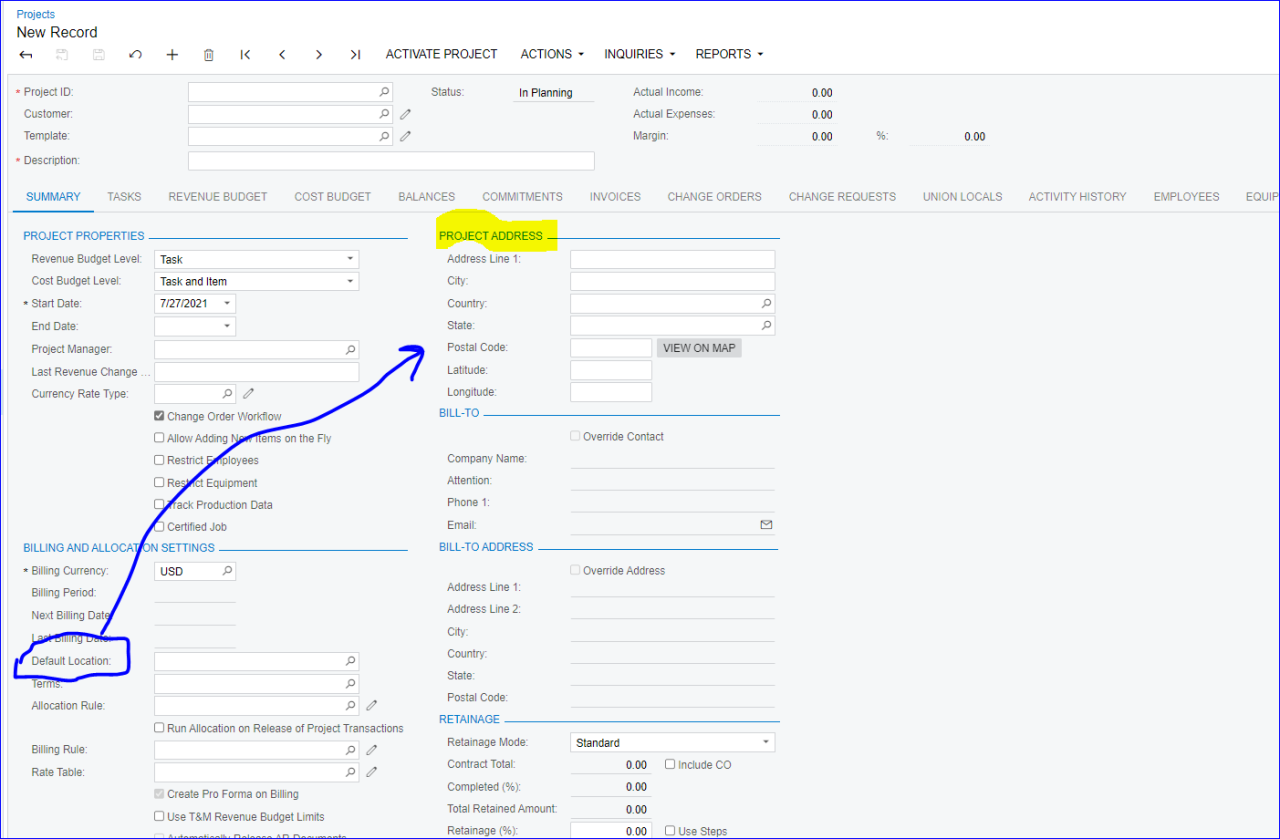
So, you’ve defined your custom location data. Fantastic! Now the real work begins: integrating this data into your existing systems and making it useful. This involves seamlessly connecting your custom locations with mapping APIs and ensuring data consistency and reliability. We’ll explore strategies for handling updates and maintaining data integrity throughout the process.Integrating custom location data requires careful planning and execution.
Success hinges on choosing the right methods for data transfer and handling potential inconsistencies. Below, we’ll cover several key aspects of this integration process.
Integrating with Mapping APIs
Using established mapping APIs like Google Maps or Mapbox requires a structured approach to data integration. Typically, this involves transforming your custom location data into a format compatible with the API’s requirements. For instance, you’ll likely need latitude and longitude coordinates, which you’ll have already derived from your custom location definition process. Many APIs accept data in GeoJSON format, a widely used standard for representing geographic data.
If your data isn’t already in this format, you’ll need to convert it. Once converted, you can then use the API’s functionalities to display your custom locations on a map, add markers, calculate distances, and more. Remember to adhere to the API’s rate limits to avoid exceeding usage allowances.
Error Handling and Data Consistency
Inconsistent or erroneous custom location data can severely impact the accuracy and reliability of your applications. Implementing robust error handling is crucial. This might involve data validation checks before integrating the data into your system. For example, you might verify that latitude and longitude coordinates fall within reasonable geographical bounds. You should also implement mechanisms to detect and handle missing or invalid data points.
A well-designed system might log errors, flag problematic data, or even automatically correct minor inconsistencies using algorithms based on nearby known locations. The key is to build a system that’s resilient to imperfect data.
Updating Custom Location Data
Updating custom location data requires a persistent storage mechanism, such as a database (e.g., PostgreSQL, MySQL, MongoDB). The choice of database depends on your specific needs and the volume of data you expect to handle. The update process itself involves modifying existing records or adding new ones. For example, if a business moves to a new address, you would update its corresponding latitude and longitude coordinates in the database.
Efficient database design, including appropriate indexing, is critical for fast and reliable updates. Regular backups are also essential to protect against data loss.
Comparing Update Approaches
Several approaches exist for handling updates. A simple approach is to batch updates, collecting changes and applying them periodically. This minimizes the number of database interactions, improving efficiency. Alternatively, real-time updates can provide immediate feedback, crucial for applications requiring up-to-the-minute accuracy. Real-time updates usually involve technologies like message queues or websockets.
The optimal approach depends on the frequency of updates and the sensitivity of your application to delays. Consider a system where user-submitted location updates are queued and processed in batches for efficiency, while critical system locations are updated in real-time.
Utilizing Custom Locations for Application Logic
Custom location data, beyond simple latitude and longitude, opens up a world of possibilities for enhancing application functionality. By enriching location information with contextual details – like addresses, points of interest, or even user-defined zones – we can build far more sophisticated and engaging applications. This allows for a level of personalization and precision not achievable with generic location services alone.
The ability to define and utilize custom locations empowers developers to create applications that go beyond simple map displays. It allows for the implementation of location-based logic that directly influences user experience and application behavior, leading to more efficient and user-friendly systems.
Calculating Distances and Proximity
Determining distances and proximity between custom locations is a fundamental operation in many location-based applications. This calculation often goes beyond simple “as the crow flies” distances, needing to account for factors like road networks or terrain for more realistic results. For example, a delivery service might use custom location data to calculate the most efficient route, factoring in traffic conditions and delivery restrictions within specific zones.
This calculation usually involves using algorithms like the Haversine formula for great-circle distances or more advanced techniques incorporating road networks from mapping APIs. For instance, if we have two custom locations, A (latitude: 34.0522, longitude: -118.2437) and B (latitude: 37.7749, longitude: -122.4194), we can use the Haversine formula to calculate the great-circle distance between them. This calculation will provide a straight-line distance, ignoring the curvature of the Earth, but this is sufficient for many applications.
More complex scenarios might require integration with routing APIs for accurate road distances.
Searching and Filtering Data Based on Custom Location Criteria
Efficiently searching and filtering data based on custom location criteria is essential for creating responsive and user-friendly location-based services. Imagine a real estate application allowing users to search for properties within a specific radius of a custom-defined “downtown” area, rather than just a simple coordinate point. This requires the application to be able to query a database of properties, comparing their locations to the custom location data using spatial queries.
A common approach involves using spatial indexing techniques like R-trees or quadtrees to speed up searches. For example, the application might use a query like “SELECTFROM properties WHERE ST_DWithin(location, ST_GeomFromText(‘POINT(custom_longitude custom_latitude)’), radius)” assuming a PostGIS database. This query finds all properties within a specified radius of a custom location.
Utilizing Custom Locations for Location-Based Services
Custom location data significantly enhances the capabilities of location-based services (LBS). Consider a restaurant finder application that allows users to define their own “neighborhood” as a custom location. The application can then use this custom location to display only restaurants within that specific area, rather than relying on a pre-defined administrative boundary. Similarly, targeted advertising can be improved by using custom locations to define specific zones for campaign delivery.
For example, a local business might want to target ads only to users within a certain radius of their store, which is defined as a custom location. This approach allows for more precise and effective targeting compared to broader geographical targeting methods. Another example is a ride-sharing service using custom locations to define “hot zones” with dynamic pricing based on demand in those areas, which could be defined by the system based on real-time data or by user-defined zones.
Data Presentation and Visualization of Custom Locations
Effectively presenting custom location data is crucial for understanding spatial patterns and making informed decisions. This involves choosing appropriate visualization methods that clearly communicate the data’s meaning to the intended audience. The right visualization can transform raw coordinates and addresses into insightful geographical representations.
HTML Table Representation of Custom Location Data
A simple and effective way to initially present custom location data is through an HTML table. This allows for a structured and easily digestible view of individual locations. The table below demonstrates this, providing latitude, longitude, address, and a descriptive field for each location.
Latitude | Longitude | Address | Description |
---|---|---|---|
34.0522 | -118.2437 | 123 Main St, Los Angeles, CA 90001 | Headquarters Office |
40.7128 | -74.0060 | 456 Elm Ave, New York, NY 10001 | East Coast Branch |
37.7749 | -122.4194 | 789 Oak Rd, San Francisco, CA 94101 | West Coast Branch |
29.7604 | -95.3698 | 101 Pine Ln, Houston, TX 77001 | Southern Office |
Map Visualization of Custom Locations
Mapping custom location data provides a powerful visual representation of spatial distribution. Using a mapping library like Leaflet or Google Maps JavaScript API, we can plot each location as a marker on a map. Each marker can be customized with icons representing different categories or attributes. Clicking a marker could reveal a pop-up window displaying detailed information from the table, such as the address and description.
This interactive element enhances user engagement and data understanding. For example, a red marker could indicate a high-priority location, while a blue marker signifies a lower-priority location. The pop-up windows could even include images related to each location.
Visualizing Relationships Between Custom Locations, Using code base to set a custom location
To show relationships between multiple custom locations, we can employ various techniques. Lines connecting locations could illustrate routes, dependencies, or connections between different offices or facilities. For instance, lines could show delivery routes from a central warehouse to various retail stores. Alternatively, clustering markers can visually group nearby locations, highlighting areas of high concentration. This approach is useful for managing a large number of locations, preventing map clutter, and showing areas with high activity.
The clustering algorithm could use proximity to group points, and the cluster markers could show the number of points aggregated.
Setting a custom location within your application’s codebase can be surprisingly powerful, especially when dealing with user preferences or geographically-specific data. This level of control is crucial, and understanding how to do this effectively is a key skill, no matter whether you’re using low-code or pro-code approaches as discussed in this great article on domino app dev the low code and pro code future.
Ultimately, mastering code-based location setting lets you build truly customized and responsive Domino applications.
Effective Communication of Custom Location Data Through Visualizations
Effective communication relies on clarity and simplicity. Choosing the right map projection, ensuring clear labeling of locations and axes, and using a consistent color scheme are vital. Interactive elements, such as zoom functionality and tooltips, significantly improve user experience. Keeping the visual representation concise and avoiding unnecessary details is crucial for preventing confusion. Consider the audience’s technical expertise when designing visualizations.
For a non-technical audience, simpler representations might be more appropriate than complex charts or maps. A legend clearly explaining the visual elements is always beneficial.
Security and Privacy Considerations for Custom Location Data: Using Code Base To Set A Custom Location
Handling custom location data responsibly is paramount. The potential for misuse, whether intentional or accidental, necessitates a robust security and privacy strategy. This goes beyond simply storing the data; it encompasses every stage, from collection to disposal. Failing to address these concerns can lead to significant legal and reputational damage, not to mention the erosion of user trust.
Data Encryption and Access Control
Protecting custom location data requires employing strong encryption both in transit and at rest. This means using protocols like HTTPS for all data transfers and encrypting data stored in databases using algorithms like AES-256. Access control mechanisms, such as role-based access control (RBAC), should be implemented to restrict access to sensitive location data to only authorized personnel. Regular security audits and penetration testing should be conducted to identify and address vulnerabilities.
For example, a system might grant only engineers access to raw location data for debugging purposes, while marketing analysts would only see aggregated, anonymized statistics.
Privacy Concerns Associated with Location Data
The nature of location data inherently reveals personal information. Storing and using this data without proper safeguards can lead to several privacy violations. For instance, precise location data can expose a user’s home address, workplace, or frequented locations, potentially making them vulnerable to stalking, targeted advertising, or even physical harm. The aggregation of location data over time can also reveal sensitive patterns of behavior, such as religious affiliations or political leanings, based on visits to specific places.
Even seemingly innocuous data can be pieced together to create a comprehensive profile.
Anonymization and Aggregation Techniques
Anonymizing and aggregating location data are crucial for protecting user privacy. Anonymization techniques, such as differential privacy, add carefully calibrated noise to the data, making it difficult to identify individual users while preserving the overall statistical properties. Aggregation involves combining individual location data points into larger groups, reducing the granularity and making it harder to trace individual movements.
For example, instead of storing precise GPS coordinates, the system could record location data only at the level of city blocks or zip codes. Similarly, aggregating location data across a large user base can reveal trends and patterns without compromising individual privacy.
Obtaining User Consent for Location Data Collection
A transparent and comprehensive consent mechanism is essential. Users must be clearly informed about what location data is being collected, how it will be used, who will have access to it, and how long it will be stored. Consent should be explicit, meaning users actively agree to the collection and use of their location data, rather than passively accepting it through pre-selected options.
The consent process should be easy to understand and accessible, allowing users to easily withdraw their consent at any time. Providing clear examples of how the location data will be used, like enhancing service accuracy or enabling personalized features, helps users understand the value exchange. Regular review and updates to the consent process are also vital to maintain transparency and adapt to evolving privacy regulations.
Last Recap
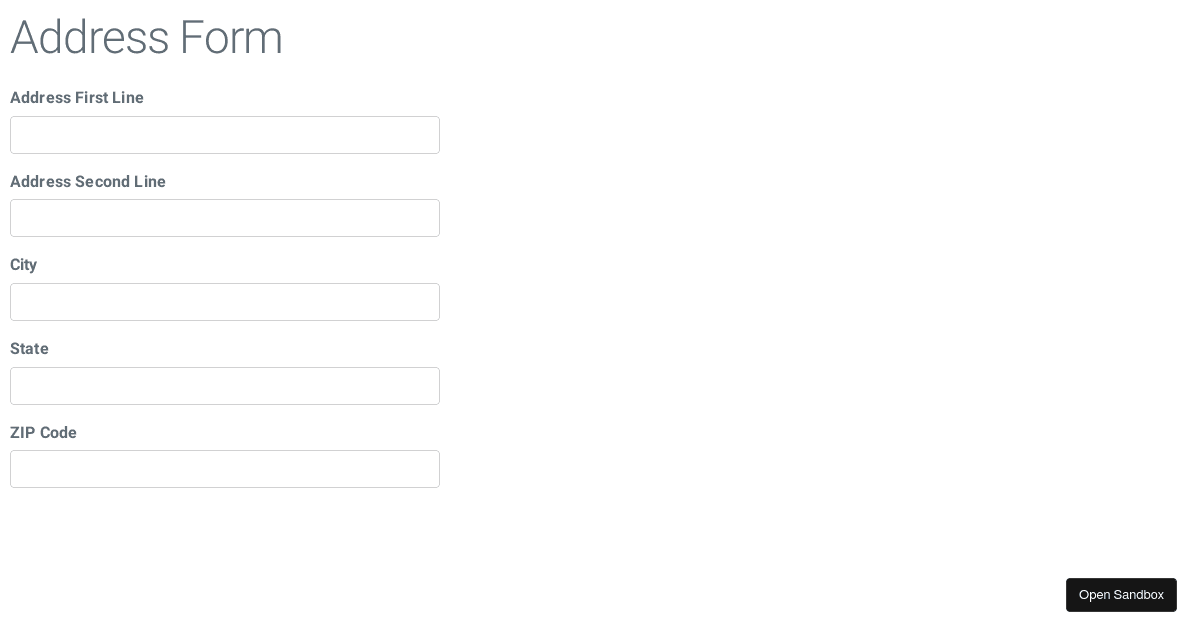
Mastering the art of setting custom locations within your codebase unlocks a powerful toolkit for creating truly location-aware applications. From efficiently storing and managing location data to creating stunning map visualizations and implementing robust security measures, we’ve covered the essential steps. Remember, understanding the nuances of data structures, API integrations, and user privacy is key to building successful and responsible location-based features.
So go forth and create location-centric applications that truly shine!
Common Queries
How do I handle address inconsistencies in my custom location data?
Address inconsistencies are common. Use a geocoding API (like Google Maps Geocoding API) to convert addresses into latitude/longitude coordinates. This provides a standardized representation, even if the original addresses were slightly different.
What are the privacy implications of storing user location data?
User location data is sensitive. Always obtain explicit consent before collecting and storing it. Anonymize or aggregate data whenever possible, and follow all relevant privacy regulations (like GDPR or CCPA).
How can I optimize database queries for location-based searches?
Use spatial indexing techniques (like R-trees or quadtrees) in your database to significantly speed up location-based searches. These indexes are specifically designed for efficient querying of geographical data.