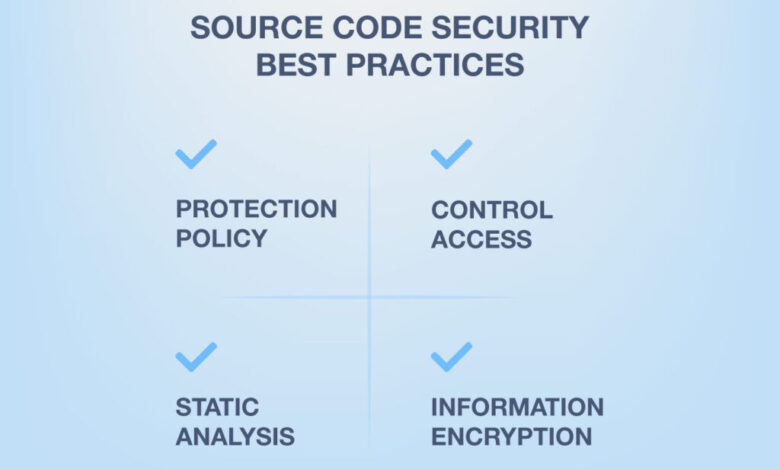
Enhancing Application Code Security Best Practices and Strategies
Enhancing application code security best practices and strategies is crucial in today’s digital landscape. From preventing common vulnerabilities like SQL injection and cross-site scripting to implementing robust authentication and authorization methods, securing your applications requires a multi-faceted approach. This involves not only writing secure code but also carefully managing dependencies, securing your infrastructure, and implementing a comprehensive security testing and vulnerability management program.
By integrating security throughout the entire software development lifecycle (SDL), you can significantly reduce your risk exposure and build more resilient applications.
This journey through application security will cover everything from secure coding principles and Software Composition Analysis (SCA) to securing your deployment environment and integrating security into your development process. We’ll explore practical techniques, tools, and strategies to help you build secure, reliable, and trustworthy applications. We’ll delve into real-world examples and best practices to make the concepts easier to understand and apply to your own projects.
Secure Coding Practices
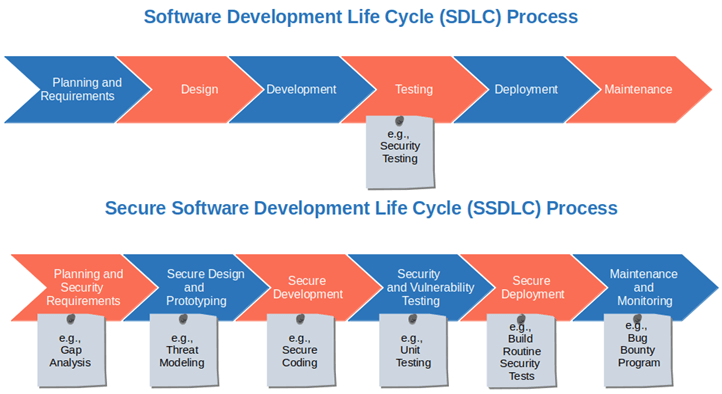
Writing secure code is paramount in today’s interconnected world. Neglecting security best practices can lead to devastating consequences, from data breaches and financial losses to reputational damage and legal repercussions. This section delves into common vulnerabilities, secure coding guidelines, authentication methods, and security testing methodologies to help developers build robust and resilient applications.
Common Coding Vulnerabilities
Several vulnerabilities frequently plague applications. Understanding these weaknesses is the first step towards mitigating them. Three prominent examples are SQL injection, cross-site scripting (XSS), and buffer overflows.
SQL Injection: This vulnerability allows attackers to inject malicious SQL code into an application’s database queries, potentially leading to data breaches, data manipulation, or even complete database takeover. Here’s an example in Python:
Vulnerable Python Code:
user_input = input("Enter username: ")query = "SELECT
FROM users WHERE username = '" + user_input + "'"
# ... execute query ...
An attacker could input ' OR '1'='1
, modifying the query to always return all users.
Vulnerable Java Code:
String username = request.getParameter("username");String query = "SELECT
FROM users WHERE username = '" + username + "'";
// ... execute query ...
Similar injection attacks are possible here. Proper parameterized queries prevent this.
Cross-Site Scripting (XSS): XSS attacks involve injecting malicious scripts into websites viewed by other users. These scripts can steal cookies, redirect users to phishing sites, or perform other malicious actions. Consider this Python example:
Vulnerable Python Code:
user_input = input("Enter your message: ")print("
" + user_input + "
")
If a user enters , the script will execute in the user’s browser.
Buffer Overflow: Buffer overflows occur when a program attempts to write data beyond the allocated buffer size. This can overwrite adjacent memory locations, leading to crashes, arbitrary code execution, or other security issues. While less common in managed languages like Java and Python, it can still happen in lower-level code or when interacting with C/C++ libraries.
Secure Coding Guidelines
Implementing robust input validation, output encoding, and error handling is crucial for building secure applications.
Input Validation: Always validate user inputs to ensure they conform to expected formats and lengths. Sanitize inputs to remove or escape potentially harmful characters. Never trust user-supplied data.
Output Encoding: Encode output data appropriately to prevent XSS attacks. HTML encoding should be used when displaying user-supplied data on a web page. Different encoding mechanisms exist for different contexts (e.g., URL encoding, JSON encoding).
Error Handling: Avoid revealing sensitive information in error messages. Generic error messages should be displayed to users, while detailed logs should be maintained for debugging purposes. Never display stack traces to end-users.
Secure Login Module Design
A secure login module incorporates all the above principles. It should use parameterized queries to prevent SQL injection, HTML-encode user inputs displayed on the page, and provide generic error messages in case of authentication failures. Strong password hashing (e.g., using bcrypt or Argon2) is also essential. The module should also implement rate limiting to prevent brute-force attacks.
Authentication and Authorization Methods
Various methods exist for authentication and authorization. Authentication verifies the user’s identity, while authorization determines what resources the user can access.
Common authentication methods include password-based authentication, multi-factor authentication (MFA), and OAuth 2.0. Authorization often uses role-based access control (RBAC) or attribute-based access control (ABAC).
Multi-Factor Authentication (MFA) Best Practices: MFA significantly enhances security by requiring users to provide multiple forms of authentication. Common factors include something you know (password), something you have (phone, security token), and something you are (biometrics). Implementing MFA involves integrating with MFA providers or building a custom solution, ensuring proper key management and secure storage of authentication factors.
Security Testing Methodologies
Different security testing methodologies offer unique advantages and disadvantages.
Type | Description | Advantages | Disadvantages |
---|---|---|---|
Static Analysis | Examines code without execution, identifying potential vulnerabilities through code review and automated tools. | Early detection of vulnerabilities, cost-effective for early-stage identification. | False positives, may miss runtime vulnerabilities. |
Dynamic Analysis | Tests running applications, identifying vulnerabilities during runtime. | Detects runtime vulnerabilities, more realistic testing environment. | More expensive and time-consuming than static analysis, may require specialized tools. |
Penetration Testing | Simulates real-world attacks to identify vulnerabilities in the application’s security posture. | Comprehensive security assessment, identifies exploitable vulnerabilities. | Expensive, requires skilled security professionals, can be disruptive. |
Software Composition Analysis (SCA)
Software Composition Analysis (SCA) is a critical security practice that’s become increasingly vital in today’s software development landscape. With the rise of open-source components and third-party libraries, understanding the security implications of the building blocks of our applications is paramount. Regularly scanning dependencies for vulnerabilities helps us identify and mitigate risks before they can be exploited, protecting our applications and our users.The Importance of Regularly Scanning Dependencies and the Impact of Outdated LibrariesRegularly scanning your project’s dependencies for known vulnerabilities is essential for maintaining a secure application.
Outdated libraries are a major source of security risks. These libraries may contain known vulnerabilities that have been patched in newer versions. Failing to update exposes your application to potential exploits, potentially leading to data breaches, denial-of-service attacks, or even complete system compromise. Imagine a scenario where a widely used logging library, vital to your application’s functionality, contains a vulnerability that allows attackers to inject malicious code.
Regular SCA scans would immediately flag this risk, allowing for prompt remediation. The consequences of ignoring this could be severe.
Common Open-Source Vulnerabilities and Their Potential Impact
Several common open-source vulnerabilities frequently impact application security. Examples include Cross-Site Scripting (XSS), SQL Injection, and insecure deserialization. XSS allows attackers to inject malicious scripts into web pages viewed by users, potentially stealing sensitive information like session cookies. SQL Injection allows attackers to manipulate database queries, potentially granting them unauthorized access to data. Insecure deserialization can allow attackers to execute arbitrary code by manipulating serialized data.
The impact of these vulnerabilities can range from minor inconveniences to major data breaches and significant financial losses.
SCA Tools
Several tools are available to perform SCA. These tools typically analyze your project’s dependency tree, comparing it against known vulnerability databases like the National Vulnerability Database (NVD). Examples of popular SCA tools include Snyk, Black Duck, and SonarQube. These tools offer various features, such as vulnerability detection, license compliance checks, and remediation guidance. The choice of tool depends on factors such as project size, complexity, and budget.
Managing Open-Source Components in the Software Development Lifecycle
A robust strategy for managing open-source components is crucial. This strategy should be integrated into the entire software development lifecycle (SDLC).
- Dependency Management: Use a consistent and version-controlled dependency management system (e.g., npm, Maven, Gradle) to track and manage all dependencies.
- Regular Vulnerability Scanning: Integrate SCA into your CI/CD pipeline to automatically scan for vulnerabilities during development and before deployment.
- Vulnerability Remediation: Establish a clear process for addressing identified vulnerabilities, prioritizing based on severity and risk.
- Policy Enforcement: Define and enforce policies regarding the use of open-source components, including allowed licenses and acceptable vulnerability thresholds.
- Security Training: Educate developers about secure coding practices and the importance of selecting secure open-source components.
Integrating SCA into a CI/CD Pipeline
Integrating SCA into your CI/CD pipeline automates the vulnerability scanning process, ensuring that security checks are performed consistently and efficiently.
- Choose an SCA Tool: Select an SCA tool that integrates with your CI/CD system (e.g., Jenkins, GitLab CI, Azure DevOps).
- Configure the Tool: Configure the selected tool to scan your project’s dependencies. This usually involves specifying the project’s source code location and the dependency management system.
- Integrate into CI/CD: Add a step to your CI/CD pipeline that executes the SCA tool. This step should run after the build process and before deployment.
- Configure Reporting: Configure the tool to generate reports that highlight identified vulnerabilities. These reports should be easily accessible to developers and security teams.
- Automated Remediation: Explore automated remediation options offered by some SCA tools to automatically update vulnerable dependencies when possible.
- Fail Build on Critical Vulnerabilities: Configure the CI/CD pipeline to fail the build if critical vulnerabilities are detected, preventing deployment of insecure applications.
Secure Deployment and Infrastructure
Securing your application’s deployment environment is as crucial as writing secure code. A robust infrastructure protects your application from external threats and ensures data integrity and availability. This section will explore best practices for securing cloud infrastructure, web servers, databases, application servers, and networks, culminating in a deployment security checklist.
Cloud Infrastructure Security Best Practices
Securing cloud infrastructure requires a multi-layered approach encompassing identity and access management (IAM), network security, data protection, and compliance. Different cloud providers offer unique security features, but common principles apply across AWS, Azure, and GCP.
- IAM: Implement the principle of least privilege, granting users only the necessary permissions. Regularly review and revoke unnecessary access. Utilize multi-factor authentication (MFA) for all accounts.
- Virtual Private Cloud (VPC): Isolate your resources within a VPC to control network access and prevent unauthorized communication. Use security groups and network access control lists (NACLs) to restrict inbound and outbound traffic.
- Data Encryption: Encrypt data at rest and in transit using industry-standard encryption algorithms. Leverage cloud provider-managed encryption services whenever possible.
- AWS Specifics: Utilize AWS Identity and Access Management (IAM), AWS Key Management Service (KMS), and AWS Shield for DDoS protection. Implement security best practices for services like S3, EC2, and RDS.
- Azure Specifics: Leverage Azure Active Directory (Azure AD), Azure Key Vault, and Azure Firewall. Implement security best practices for services like Azure Blob Storage, Azure Virtual Machines, and Azure SQL Database.
- GCP Specifics: Utilize Google Cloud Identity and Access Management (IAM), Cloud Key Management Service (KMS), and Cloud Armor for DDoS protection. Implement security best practices for services like Cloud Storage, Compute Engine, and Cloud SQL.
Secure Web Server Configuration (Apache)
Apache web servers require careful configuration to prevent vulnerabilities. Key considerations include disabling unnecessary modules, strengthening authentication mechanisms, and implementing robust logging.
- Disable unnecessary modules: Remove modules not required by your application to reduce the attack surface.
- Strong authentication: Use HTTPS with strong SSL/TLS certificates. Implement robust password policies and consider two-factor authentication.
- Regular updates: Keep Apache and all associated software updated with the latest security patches.
- Detailed logging: Configure detailed logging to track access attempts and potential security breaches.
- Restrict access: Use .htaccess files or other mechanisms to restrict access to sensitive directories and files.
Secure Database Configuration (MySQL)
MySQL database security centers around user access control, encryption, and regular updates.
- Strong passwords: Enforce strong password policies for all database users. Use a password manager to generate and store secure passwords.
- Least privilege: Grant users only the necessary privileges to perform their tasks.
- Regular backups: Implement regular database backups to protect against data loss and ransomware attacks.
- Encryption: Encrypt data at rest and in transit using strong encryption algorithms.
- Regular updates: Keep MySQL and all associated software updated with the latest security patches.
Secure Application Server Configuration (Tomcat)
Tomcat, like other application servers, requires careful configuration to prevent vulnerabilities.
- Restrict access: Configure Tomcat to listen only on the necessary network interfaces. Avoid exposing Tomcat directly to the public internet.
- Secure manager application: Protect the Tomcat Manager application with strong authentication and authorization mechanisms.
- Regular updates: Keep Tomcat and all associated software updated with the latest security patches.
- Disable unnecessary features: Disable unnecessary features and modules to reduce the attack surface.
- Use HTTPS: Configure Tomcat to use HTTPS with a strong SSL/TLS certificate.
Network Security and Firewalls
Firewalls act as the first line of defense, controlling network traffic and preventing unauthorized access. Different firewall types offer varying levels of protection.
- Packet filtering firewalls: Examine individual packets based on source and destination IP addresses, ports, and protocols.
- Stateful inspection firewalls: Track the state of network connections to filter traffic more effectively.
- Application-level firewalls: Inspect application-level traffic to identify and block malicious activity.
- Next-generation firewalls (NGFWs): Combine multiple firewall technologies with advanced features like intrusion prevention systems (IPS) and malware detection.
The choice of firewall depends on the application’s security requirements and the level of protection needed. NGFWs are generally recommended for higher security needs.
Deployment Security Checklist
This checklist summarizes key steps for securing a deployment environment.
So, you’re thinking about enhancing application code security best practices and strategies? It’s a crucial aspect of modern development, and the approach often depends on your development methodology. For instance, consider how these best practices apply to the evolving landscape of application development, as outlined in this insightful piece on domino app dev the low code and pro code future.
Ultimately, robust security needs to be built into every stage, regardless of your chosen development path.
- Operating System Hardening: Disable unnecessary services, regularly update the OS and applications, and implement strong password policies.
- Network Security: Configure firewalls to restrict access to only necessary ports and IP addresses. Implement intrusion detection/prevention systems.
- Access Control: Implement role-based access control (RBAC) to restrict access to sensitive resources. Use multi-factor authentication (MFA) wherever possible.
- Data Encryption: Encrypt data at rest and in transit using industry-standard encryption algorithms.
- Regular Security Audits: Conduct regular security audits and penetration testing to identify and address vulnerabilities.
- Vulnerability Management: Implement a vulnerability management program to track and address known vulnerabilities in your applications and infrastructure.
- Incident Response Plan: Develop and regularly test an incident response plan to handle security breaches effectively.
Security Testing and Vulnerability Management
Ensuring application security isn’t a one-time task; it’s an ongoing process requiring continuous monitoring and improvement. Security testing and vulnerability management are crucial components of this process, allowing us to proactively identify and mitigate risks before they can be exploited. This section delves into the practical aspects of implementing a robust security testing and vulnerability management program.
Penetration Testing Phases and Tools
Penetration testing, also known as ethical hacking, simulates real-world attacks to identify vulnerabilities in a system. A typical penetration test involves several distinct phases. These phases are iterative and may overlap depending on the specific test scope and methodology.
- Planning and Scoping: This initial phase defines the objectives, targets, and rules of engagement for the test. It includes identifying the systems and applications to be tested, defining the types of attacks allowed, and establishing communication channels with the client.
- Reconnaissance: The tester gathers information about the target system, including network topology, operating systems, and applications. This phase uses various tools like Nmap (for network scanning) and Shodan (for searching for exposed services).
- Vulnerability Analysis: Testers identify potential vulnerabilities using automated tools like Nessus and OpenVAS, as well as manual techniques. This stage focuses on identifying weaknesses in the system’s security posture.
- Exploitation: This phase involves attempting to exploit identified vulnerabilities to gain unauthorized access or control. The tester documents the success or failure of each exploit attempt.
- Post-Exploitation: If exploitation is successful, this phase focuses on determining the extent of the compromise. The tester may attempt to move laterally within the network to assess the impact of the breach.
- Reporting: The final phase involves documenting all findings, including identified vulnerabilities, exploitation techniques, and recommendations for remediation. A comprehensive report provides detailed information about the security posture of the system and actionable steps for improvement.
Vulnerability Management Program
A well-defined vulnerability management program is essential for maintaining a secure application environment. The program should encompass a systematic process for identifying, assessing, remediating, and reporting vulnerabilities.A flowchart representing this process could look like this:[Imagine a flowchart here. The flowchart would show a cyclical process starting with “Vulnerability Identification” (using tools like static/dynamic analysis, penetration testing, and vulnerability scanners).
This feeds into “Vulnerability Assessment” (prioritizing vulnerabilities based on severity and likelihood of exploitation, using risk matrices like CVSS). Next is “Remediation” (applying patches, code fixes, configuration changes). This leads to “Verification” (retesting to confirm the vulnerability is resolved). Finally, “Reporting” (generating reports for stakeholders, including management and developers) completes the cycle, feeding back into “Vulnerability Identification” for continuous monitoring.]
Comparison of Static and Dynamic Security Testing Tools, Enhancing application code security best practices and strategies
Static and dynamic analysis tools play crucial roles in identifying security vulnerabilities. Static analysis examines the source code without executing it, identifying potential flaws through pattern matching and code analysis. Dynamic analysis examines the application’s behavior during runtime, detecting vulnerabilities that may only appear during execution.
Feature | Static Analysis | Dynamic Analysis |
---|---|---|
Analysis Method | Analyzes source code without execution | Analyzes application behavior during runtime |
Vulnerability Detection | Identifies potential vulnerabilities based on code patterns | Identifies vulnerabilities based on application behavior |
Coverage | Can detect vulnerabilities that may not be apparent during runtime | May miss vulnerabilities that only appear under specific conditions |
Examples | FindBugs, SonarQube, Coverity | Burp Suite, OWASP ZAP, AppScan |
Code Review Process for Security Flaws
Code reviews are a crucial part of a secure development lifecycle. They involve systematically examining source code to identify security vulnerabilities and coding errors. A structured code review process should involve multiple reviewers, checklists, and a clear reporting mechanism.For example, a common issue found during code reviews is SQL injection. A vulnerable code snippet might look like this:
String query = "SELECT
FROM users WHERE username = '" + username + "'";
This code is vulnerable because it directly concatenates user input into the SQL query, allowing attackers to inject malicious SQL code. A secure version would use parameterized queries or prepared statements:
String query = "SELECT
FROM users WHERE username = ?";
PreparedStatement statement = connection.prepareStatement(query);statement.setString(1, username);
Another common issue is cross-site scripting (XSS). Failing to properly sanitize user input can lead to attackers injecting malicious JavaScript code into the application, potentially stealing user data or manipulating the user interface. A careful review of input validation and output encoding practices is essential to prevent such vulnerabilities.
Secure Development Lifecycle (SDL) Integration: Enhancing Application Code Security Best Practices And Strategies
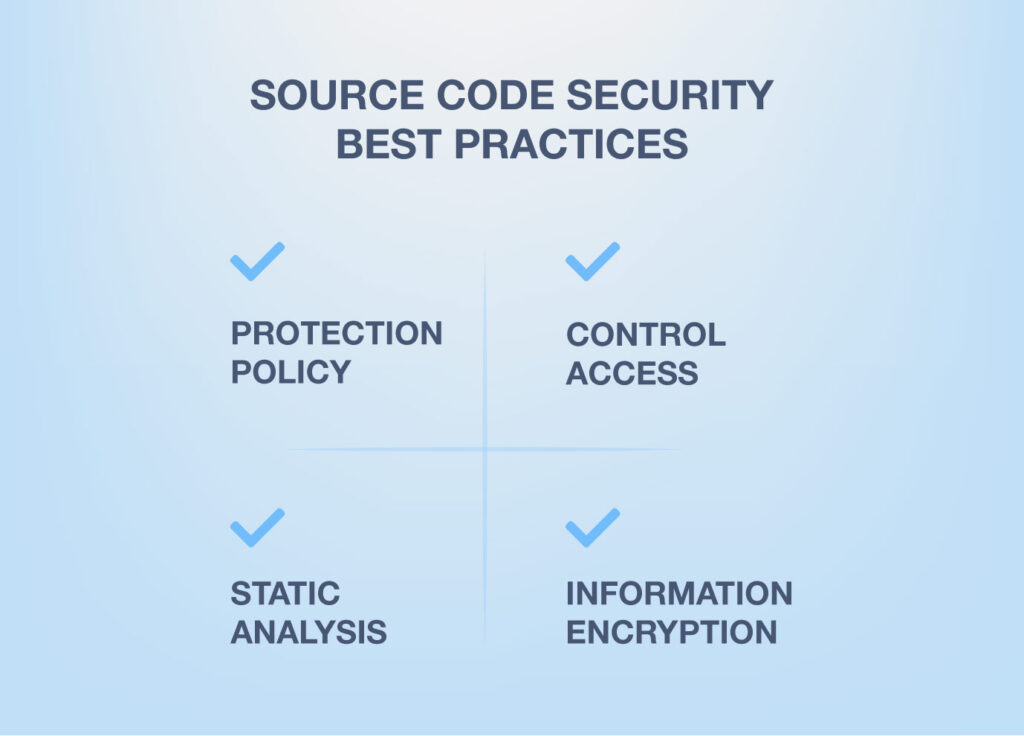
Integrating security practices throughout the entire software development lifecycle (SDL) is no longer a luxury; it’s a necessity. Building security in from the start, rather than trying to bolt it on later, significantly reduces vulnerabilities and ultimately lowers the cost of remediation. This shift-left approach to security emphasizes proactive measures, preventing issues before they become major problems.Shifting security left offers several key benefits.
Early detection of vulnerabilities is cheaper and easier to fix than addressing them in later stages. It also leads to more secure code, reduced development time (by avoiding costly rework), and improved developer productivity. By embedding security into every phase, we build a more robust and resilient system.
Incorporating Security Requirements into Design and Architecture Reviews
Security requirements should be considered alongside functional requirements from the very beginning of a project. This involves integrating security considerations into design and architecture reviews, using threat modeling techniques to identify potential vulnerabilities and mitigating them proactively. For example, during the design phase, a review might highlight the need for input validation to prevent injection attacks or the implementation of robust authentication mechanisms to protect sensitive data.
Secure design patterns, such as the use of layered security architectures, principle of least privilege, and defense in depth, should be applied consistently.
Secure Design Patterns
Several well-established secure design patterns can be incorporated to improve application security. The principle of least privilege dictates that users and processes should only have the minimum access rights necessary to perform their tasks. This limits the damage that can be caused by a security breach. Defense in depth involves implementing multiple layers of security controls, so that if one layer fails, others are in place to protect the system.
A layered security architecture might involve firewalls, intrusion detection systems, and access control lists working together.
Security Training and Awareness for Developers
Security training is not a one-time event; it’s an ongoing process. Regular training sessions, workshops, and awareness campaigns are essential to fostering a security-conscious culture. Training should cover topics such as secure coding practices, common vulnerabilities (OWASP Top 10), and the importance of secure development practices. Best practices include incorporating security training into onboarding processes, providing regular updates on emerging threats, and encouraging developers to report vulnerabilities proactively.
Creating a culture where security is everyone’s responsibility is crucial. This can be achieved through incentives, recognition programs, and open communication channels.
Security Activities Throughout the Software Development Lifecycle
The following table Artikels the security activities for each phase of a typical SDL. Note that these activities are iterative and often overlap.
Phase | Activity | Responsible Party | Deliverables |
---|---|---|---|
Requirements | Identify security requirements, threat modeling | Security Architect, Developers | Threat model, security requirements specification |
Design | Security design review, architecture review, secure design pattern selection | Security Architect, Developers, Architects | Secure design document, architecture diagrams |
Implementation | Secure coding practices, code reviews, static analysis | Developers, Security Engineers | Secure code, static analysis reports |
Testing | Security testing (penetration testing, vulnerability scanning), code review | Security Engineers, Testers | Security test reports, vulnerability remediation plan |
Deployment | Secure configuration management, infrastructure security hardening | DevOps Engineers, Security Engineers | Secure deployment plan, hardened infrastructure |
Maintenance | Vulnerability management, security patching, monitoring | Security Engineers, Operations Team | Vulnerability reports, security patches, monitoring dashboards |
Closing Summary
Ultimately, enhancing application code security isn’t a one-time fix, but an ongoing process requiring vigilance and a commitment to best practices. By embracing a proactive, holistic approach that incorporates secure coding, robust testing, and a well-defined vulnerability management program, you can significantly minimize vulnerabilities and protect your applications from evolving threats. Remember, a secure application is a resilient application, safeguarding both your data and your reputation.
Questions Often Asked
What are some common misconceptions about application security?
A common misconception is that security is an afterthought, added at the end of the development process. In reality, security should be integrated throughout the entire SDLC (Software Development Lifecycle). Another misconception is that only large companies need to worry about application security; small and medium-sized businesses are equally vulnerable.
How often should I perform security testing?
The frequency of security testing depends on several factors, including the criticality of your application, the frequency of code changes, and the threat landscape. However, regular testing – at least annually, and ideally more frequently for high-risk applications – is essential.
What’s the difference between static and dynamic application security testing (SAST and DAST)?
SAST analyzes code without executing it, identifying vulnerabilities in the source code itself. DAST analyzes a running application, identifying vulnerabilities in the application’s behavior and interactions.
How can I encourage a security-conscious culture within my development team?
Regular security training, integrating security into code reviews, and rewarding secure coding practices are all crucial steps in fostering a security-conscious culture. Open communication and a blame-free environment for reporting vulnerabilities are also essential.