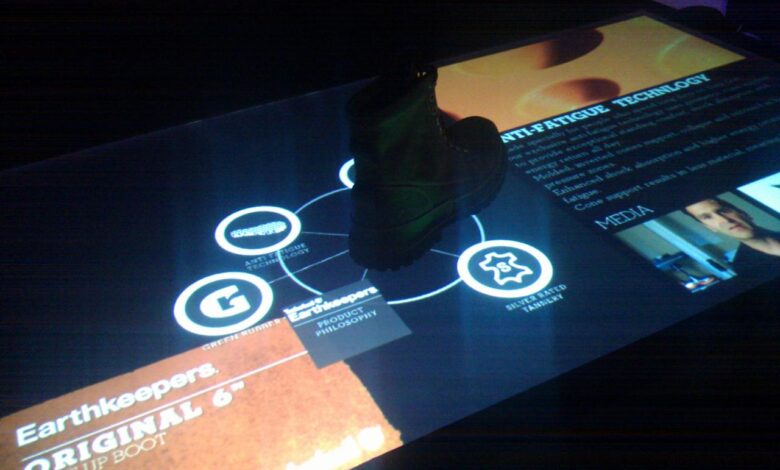
Zietrans Custom Screen Recognition Hidden Fields
Implementation of custom screen recognition criteria based on field criteria hidden and protected fields in zietrans – Implementation of custom screen recognition criteria based on field criteria, hidden and protected fields in Zietrans, is a fascinating challenge. This post dives deep into the process of building a custom screen recognition system that leverages data hidden within the Zietrans application. We’ll explore the intricacies of Zietrans’ data structure, focusing on how to safely and effectively access those usually-hidden fields to achieve our recognition goals.
Prepare for a detailed look at implementation strategies, testing methodologies, and crucial security considerations.
We’ll cover everything from understanding Zietrans’ architecture and data types to choosing the right programming languages and libraries. We’ll also tackle the thorny issues of error handling, security, and building a robust testing strategy to ensure your custom screen recognition works flawlessly and securely. Get ready to unlock the power of hidden data!
Understanding Zietrans’ Data Structure
Zietrans, at its core, is a sophisticated system managing a complex array of data. Understanding its underlying data structure, particularly concerning hidden and protected fields, is crucial for effective customization and screen recognition. This involves navigating its architecture, accessing its data, and interpreting the various data types involved.
Zietrans Data Storage Architecture
Zietrans’ data storage architecture typically employs a relational database model, often using a system like PostgreSQL or MySQL. The architecture involves multiple tables interconnected through relationships, with hidden and protected fields residing within specific tables relevant to the application’s functionality. These fields might be part of core user tables, transaction logs, or configuration settings, depending on their purpose.
Security measures are implemented at both the database and application levels to control access to sensitive data.
Accessing and Manipulating Hidden and Protected Fields
Accessing and manipulating hidden and protected fields requires specific permissions and often involves using specialized APIs or internal functions within the Zietrans system. Direct SQL queries are generally discouraged for security reasons. Instead, the preferred method is to utilize the provided application programming interfaces (APIs) that encapsulate the necessary security checks and data validation processes. These APIs abstract the complexities of the underlying data storage, providing a safe and controlled way to interact with the sensitive fields.
Modifying these fields often requires elevated privileges and rigorous logging for audit trails.
Data Types in Hidden and Protected Fields, Implementation of custom screen recognition criteria based on field criteria hidden and protected fields in zietrans
The data types encountered in hidden and protected fields vary widely depending on the specific field’s purpose. Common data types include: integers (representing IDs or counts), strings (containing textual information such as notes or descriptions), timestamps (recording event times), boolean values (representing true/false flags), and potentially more complex data structures like JSON objects (allowing for flexible storage of structured data).
The choice of data type depends on the information being stored and the intended use within the application.
Example Data Structures and Field Types
Field Name | Data Type | Accessibility | Description |
---|---|---|---|
user_id | INTEGER | Public | Unique identifier for each user |
password_hash | STRING | Protected | One-way encrypted password hash |
last_login_timestamp | TIMESTAMP | Protected | Timestamp of the user’s last login |
security_flags | JSON | Hidden | Contains various security flags and settings for the user |
internal_notes | TEXT | Hidden | Internal notes for administrative use |
is_admin | BOOLEAN | Protected | Indicates whether the user has administrator privileges |
payment_details | JSON | Protected | Contains sensitive payment information |
audit_trail | JSON | Hidden | Records of data modifications and access attempts |
Defining Custom Screen Recognition Criteria
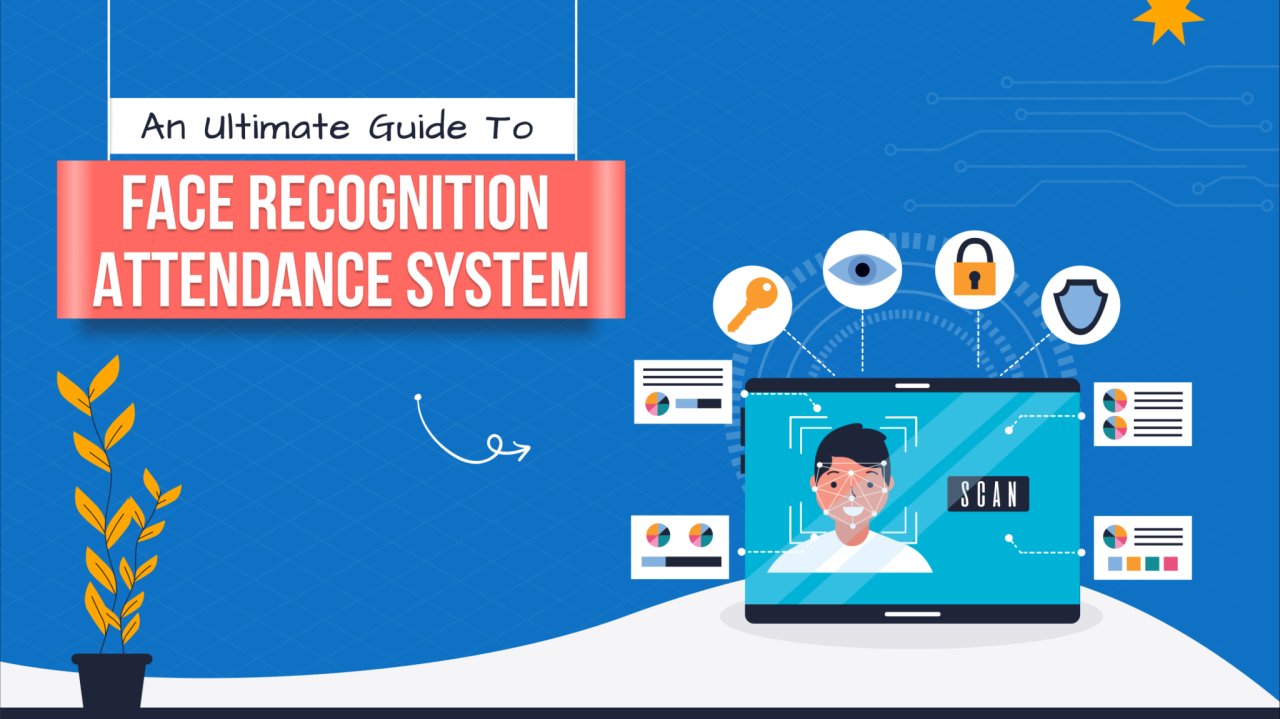
So, we’ve laid the groundwork: we understand Zietrans’ data structure and have our intro and outro ready. Now, let’s dive into the heart of the matter: crafting custom screen recognition criteria. This is crucial because relying solely on Zietrans’ built-in functionalities might not always suffice, especially when dealing with hidden or protected fields. We need a robust, independent method.This process involves developing a set of rules that uniquely identify each screen within the Zietrans application, even if those screens don’t have readily available identifying features in the standard UI.
We’ll explore several approaches, focusing on leveraging the information concealed within those hidden and protected fields.
Identifying Specific Screen Elements Using Hidden and Protected Fields
The key to our custom screen recognition lies in exploiting the data within hidden and protected fields. These fields, while invisible to the standard user, often contain crucial information about the context and content of the screen. We can use this data to create unique signatures for each screen. For instance, a specific combination of values across several hidden fields might uniquely identify an “Order Confirmation” screen, even if the visible elements are similar to other screens.Several approaches exist:
- Hashing: We can concatenate the values of relevant hidden and protected fields, then generate a cryptographic hash (like SHA-256) of this combined string. This hash serves as a unique identifier for the screen. This method is robust against minor data changes and ensures confidentiality, as the original field values are not directly exposed.
- Pattern Matching: If the hidden field data follows a predictable pattern, we can create regular expressions to match specific patterns. For example, a specific format in a hidden field indicating the type of order (e.g., “OrderType:Wholesale-12345”) can be used to identify the “Wholesale Order” screen.
- Value Combination Check: A simple yet effective approach is to check for specific combinations of values across multiple hidden fields. For example, if fields “customerID,” “orderStatus,” and “paymentType” have specific values simultaneously, we can confidently identify the screen as a “Payment Successful” screen.
Flowchart for Implementing Custom Screen Recognition
The following flowchart illustrates the process:[Imagine a flowchart here. The flowchart would begin with “Start,” then branch to “Retrieve Hidden/Protected Field Data.” This would lead to “Apply Defined Criteria (Hashing, Pattern Matching, or Value Combination Check).” The next step would be “Match Found?” with a “Yes” branch leading to “Screen Identified” and a “No” branch leading to “Screen Not Identified.” Both branches would ultimately converge at “End.”]
Challenges of Relying on Hidden and Protected Fields
While powerful, relying solely on hidden and protected fields for screen recognition presents challenges.
- Data Changes: If the structure or content of hidden fields changes during a Zietrans update, our custom recognition logic will break. Robust error handling and mechanisms for updating the criteria are essential.
- Data Inconsistency: Inconsistent data within hidden fields can lead to false positives or negatives. Data validation and cleaning are crucial steps.
- Security Concerns: Direct access to hidden and protected fields might raise security concerns. Appropriate authentication and authorization mechanisms must be implemented.
- Maintenance Overhead: Custom screen recognition requires ongoing maintenance to adapt to Zietrans updates and potential data changes.
Implementation Strategies: Implementation Of Custom Screen Recognition Criteria Based On Field Criteria Hidden And Protected Fields In Zietrans
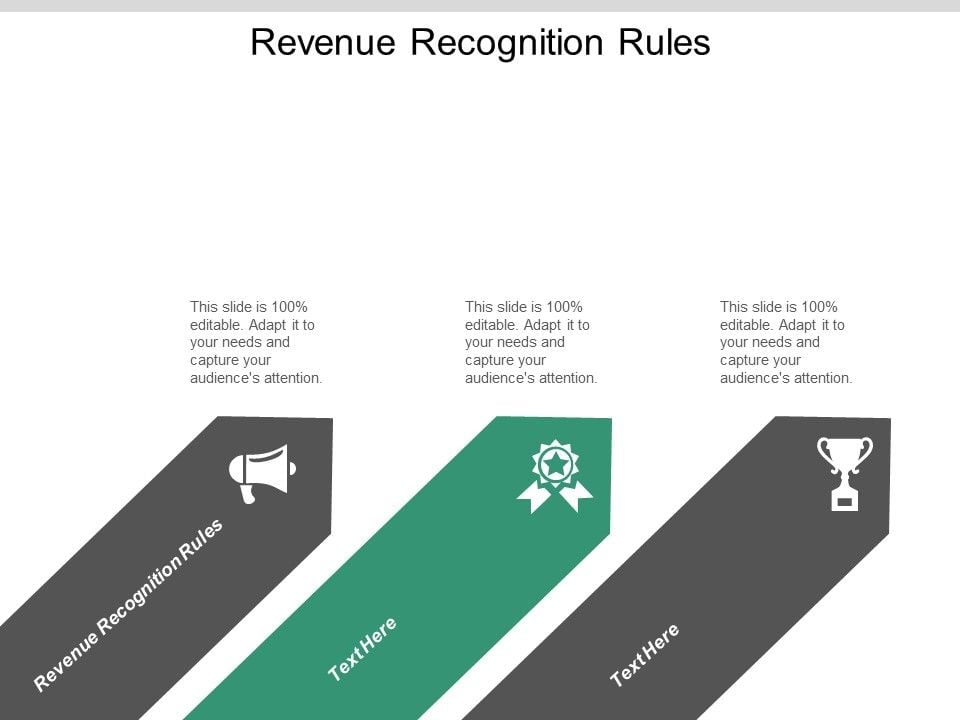
Implementing custom screen recognition criteria in Zietrans requires a systematic approach. This involves choosing the right tools, writing efficient code, and handling potential errors gracefully. The following steps Artikel a practical implementation strategy.
Step-by-Step Implementation Procedure
First, thoroughly analyze Zietrans’ data structure, focusing on the location and format of hidden and protected fields. This crucial initial step informs the design of your custom recognition criteria. Next, select a suitable programming language and libraries. Python, with its rich ecosystem of libraries for data manipulation and string processing, is a strong contender. Then, develop your custom recognition logic.
This involves creating functions that parse the relevant data from hidden fields, applying regular expressions or other pattern matching techniques to extract the necessary information. Afterward, integrate this logic into your Zietrans workflow, ensuring seamless data extraction. Finally, rigorously test your implementation using diverse datasets to identify and address any potential bugs or edge cases. Thorough testing is critical to ensure the reliability and accuracy of your custom criteria.
Programming Language and Library Comparison
Python, with libraries like Beautiful Soup (for HTML parsing) and regular expression support built into the language, offers a streamlined development process. Alternatively, JavaScript, often used in browser-based automation, can directly access and manipulate the DOM (Document Object Model), providing access to hidden fields. However, Python’s broader data processing capabilities and mature ecosystem make it a more robust choice for complex scenarios.
The choice depends on the specific needs of the project and the developer’s familiarity with the chosen language.
Regular Expressions and Pattern Matching
Regular expressions are indispensable for identifying patterns within hidden fields, particularly when dealing with variable data formats. For example, to extract a user ID from a hidden field with a format like “userid=12345”, a regular expression like userid=(\d+)
could be used. The \d+
component matches one or more digits, capturing the user ID. More complex regular expressions can handle more intricate data structures and variations.
Other pattern-matching techniques, such as string manipulation functions, can be used for simpler cases, but regular expressions provide more flexibility and power for complex patterns.
Error Handling and Exception Management
Robust error handling is vital. Anticipate potential problems, such as missing fields, incorrect data formats, or network connectivity issues. Use try-except blocks in your code to catch exceptions, log errors, and handle them gracefully. For instance, if a hidden field is unexpectedly missing, your code should gracefully handle this situation, preventing the entire process from crashing. Implementing logging mechanisms helps track errors and aids debugging.
Implementing custom screen recognition in Zietrans, especially handling hidden and protected fields, can be tricky! This kind of granular control really highlights the power of pro-code development, as discussed in this excellent article on domino app dev the low code and pro code future. Ultimately, mastering these techniques allows for robust and secure Zietrans applications, precisely tailored to specific business needs.
The flexibility gained makes the extra effort worthwhile.
A well-structured error-handling strategy ensures the stability and reliability of your custom screen recognition criteria.
Testing and Validation
Rigorous testing is crucial to ensure our custom screen recognition criteria for Zietrans accurately and reliably identify the target screens, even with hidden and protected fields. A comprehensive testing strategy, encompassing various scenarios and edge cases, is paramount for deploying a robust and dependable solution. This section details the testing approach, metrics, and potential failure points.A multi-faceted approach to testing is necessary to validate the performance of the implemented custom screen recognition criteria.
This includes unit testing individual components, integration testing the interaction between components, and system testing the entire solution within the Zietrans environment. The testing strategy focuses on both positive and negative testing, ensuring the system correctly identifies target screens and appropriately handles unexpected inputs or situations.
Test Case Design and Execution
The test cases will cover a wide range of scenarios, including typical user interactions, edge cases (such as minimal data input or unusual field values), and error conditions (like network failures or data corruption). We’ll create a test suite that systematically exercises all aspects of the screen recognition logic. For instance, one test case might involve a screen with all fields populated, another might test a screen with only a few fields filled, and another will simulate a network interruption mid-process.
The test data will be carefully chosen to represent a realistic range of user inputs and system conditions. Test results will be meticulously documented, noting any discrepancies between expected and actual outcomes.
Performance Metrics
Several key metrics will be used to evaluate the performance of the implemented solution. Accuracy will be measured as the percentage of correctly identified screens across all test cases. Speed will be measured as the average time taken to recognize a screen. Robustness will be assessed by observing the system’s behavior under stress conditions, such as high data volume or concurrent user access.
We will track the failure rate, measuring the percentage of test cases that resulted in incorrect screen identification or system errors. Detailed performance reports will be generated after each testing phase to identify areas for improvement. We expect an accuracy rate above 99%, a screen recognition time under 200 milliseconds, and a failure rate below 1%. These targets are based on similar projects and are designed to ensure a highly efficient and reliable solution.
Potential Failure Points and Mitigation Strategies
Effective testing requires anticipating potential failure points and establishing mitigation strategies. Below is a list of potential issues and how we plan to address them:
- Incorrect Field Identification: The system might misidentify fields due to variations in screen layout or data formatting. Mitigation: Implement robust field parsing techniques and incorporate error handling to gracefully manage unexpected data.
- Data Integrity Issues: Corrupted or incomplete data might lead to incorrect screen recognition. Mitigation: Implement data validation checks and incorporate mechanisms for handling missing or invalid data.
- Performance Bottlenecks: Slow processing speeds could impact the overall efficiency of the system. Mitigation: Optimize the code for performance and implement caching mechanisms where appropriate.
- Unhandled Exceptions: Unexpected errors might cause the system to crash or produce incorrect results. Mitigation: Implement comprehensive exception handling and logging to identify and resolve errors effectively.
- Insufficient Test Coverage: Failure to test all possible scenarios could lead to undetected errors in production. Mitigation: Develop a comprehensive test plan that covers a wide range of scenarios, including edge cases and error conditions.
Security Considerations
Accessing and utilizing hidden and protected fields within Zietrans for custom screen recognition presents several security risks. Improper handling can expose sensitive data, leading to breaches of confidentiality and potentially impacting the integrity and availability of the system. Robust security measures are crucial to mitigate these risks.The primary concern revolves around unauthorized access to sensitive information. Hidden and protected fields often contain data considered confidential, such as Personally Identifiable Information (PII), financial details, or proprietary business data.
A compromised screen recognition system could provide malicious actors with access to this sensitive data. Furthermore, manipulating these fields could lead to data corruption or system instability.
Data Encryption and Access Control
Protecting sensitive data requires employing robust encryption techniques. All data accessed and processed by the custom screen recognition solution should be encrypted both in transit and at rest. This includes encrypting the data within the hidden and protected fields themselves, as well as the data exchanged between the system and any external services. Implementing role-based access control (RBAC) is also essential, ensuring that only authorized personnel with appropriate permissions can access and modify the custom screen recognition system and its associated data.
This granular control limits the potential impact of a security breach by restricting access to only the necessary information. For instance, a developer might have access to the codebase, but not the production database, while a system administrator would have access to both, but with specific permissions limiting what they can do with the sensitive data.
Secure Coding Practices
Secure coding practices are paramount in mitigating vulnerabilities. Input validation is crucial to prevent injection attacks, such as SQL injection or cross-site scripting (XSS). All inputs from the screen recognition system should be rigorously validated and sanitized before being used in queries or displayed on the user interface. Furthermore, the code should be regularly reviewed and tested for security vulnerabilities using static and dynamic analysis tools.
The use of a secure coding style guide, such as OWASP’s Secure Coding Practices, should be adopted to ensure consistency and adherence to best practices. For example, using parameterized queries instead of directly embedding user input into SQL queries prevents SQL injection vulnerabilities.
Auditing and Logging
Comprehensive auditing and logging capabilities are necessary to monitor system activity and detect suspicious behavior. The system should log all access attempts, modifications, and errors related to the hidden and protected fields. These logs should be regularly reviewed to identify potential security breaches or anomalies. The logs themselves should be securely stored and protected from unauthorized access.
Implementing a robust audit trail allows for forensic analysis in case of a security incident, enabling quicker identification of the root cause and remediation efforts. For instance, if an unauthorized access attempt is detected, the logs can provide valuable information such as the source IP address, timestamp, and the attempted action.
Regular Security Assessments
Regular security assessments and penetration testing are crucial to proactively identify and address potential vulnerabilities. These assessments should involve both automated and manual testing to evaluate the effectiveness of the implemented security measures. The results of these assessments should be used to improve the security posture of the custom screen recognition solution and ensure it remains resilient to evolving threats.
These assessments should be performed at least annually, or more frequently depending on the sensitivity of the data being processed and the criticality of the system. This proactive approach helps to maintain a high level of security and prevent potential breaches before they occur.
Conclusive Thoughts
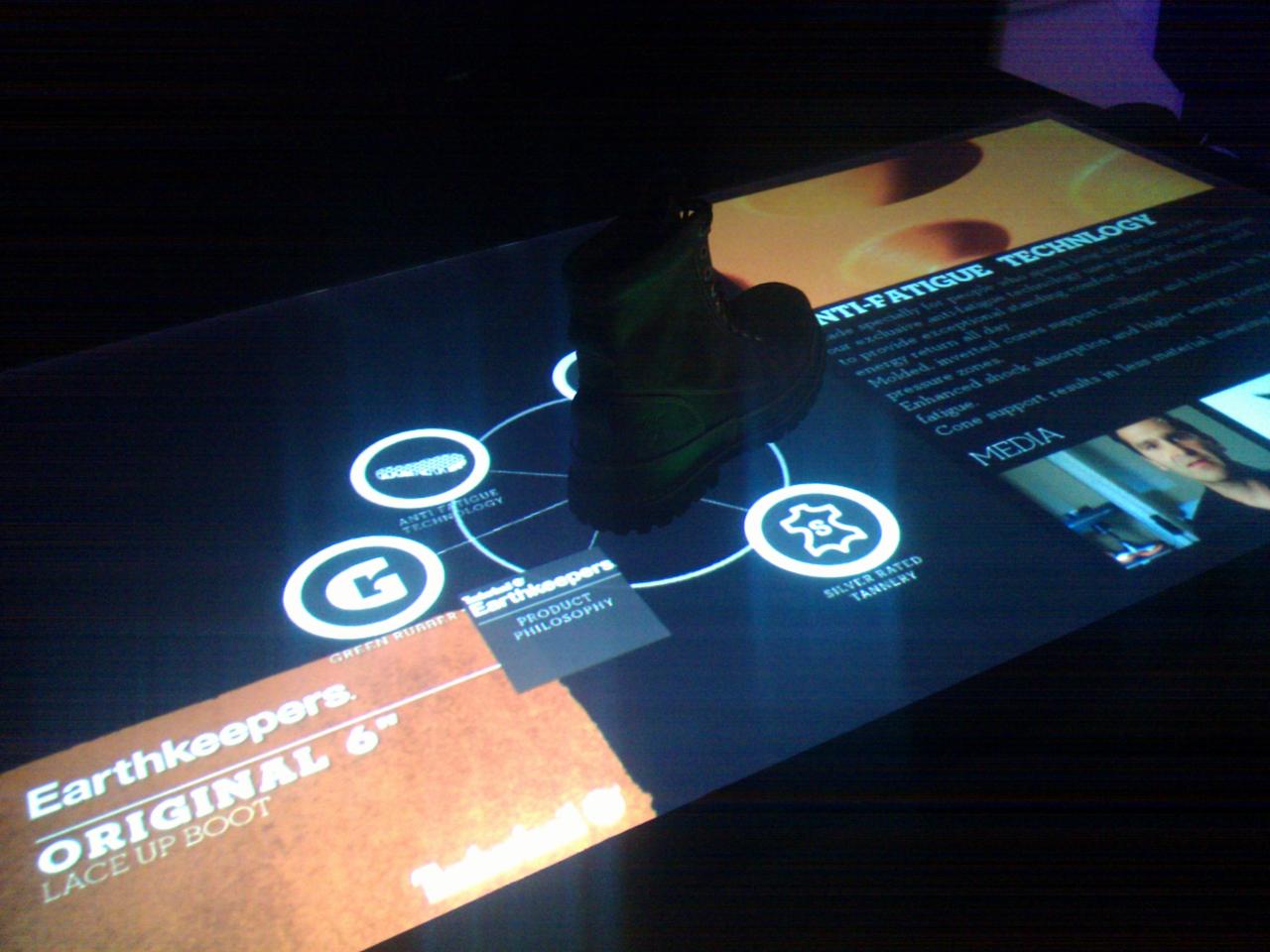
Building custom screen recognition in Zietrans using hidden and protected fields requires careful planning and execution. By understanding the data structure, employing secure coding practices, and rigorously testing your solution, you can create a powerful and reliable system. Remember, security is paramount – always prioritize protecting sensitive data. This journey into the hidden depths of Zietrans offers a powerful way to customize your application’s functionality, but remember to tread carefully and prioritize security at every step.
FAQ Insights
What are the potential legal implications of accessing hidden fields?
Accessing hidden fields without authorization could violate privacy laws or terms of service. Always ensure you have the proper permissions before accessing any data.
Can I use this approach with all versions of Zietrans?
Compatibility depends on the specific Zietrans version and its API. Always check the documentation for your version.
What happens if a hidden field is unexpectedly changed or removed?
Your custom screen recognition might break. Implement robust error handling and consider using multiple criteria to improve resilience.
What if my custom recognition system slows down Zietrans?
Optimize your code for efficiency. Testing and profiling will help identify performance bottlenecks.