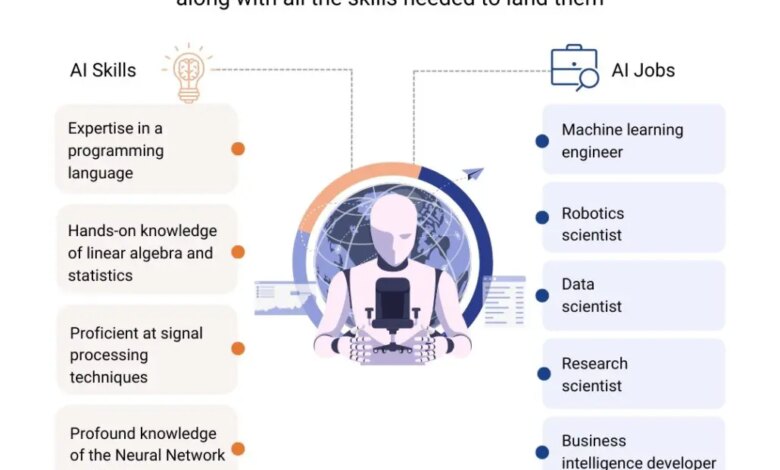
Essential Topics to Study for an AI Career
Essential topics to study for a career in artificial intelligence? Think of it like this: you’re building a rocket ship to the future. This isn’t just about coding; it’s about mastering the underlying mathematics that fuels AI’s power, understanding the intricate programming languages that bring algorithms to life, and navigating the ethical considerations that shape its development. We’re diving deep into the core subjects that will launch your AI career into orbit.
This post breaks down the key areas you need to conquer – from the fundamental math and programming skills to the complexities of machine learning, deep learning, NLP, computer vision, and the crucial ethical considerations. We’ll explore each area, providing practical examples and insights to help you chart your course towards a successful career in this exciting field. Get ready for liftoff!
Mathematics for AI
AI, at its core, is about building systems that can learn and make decisions. This learning and decision-making process relies heavily on mathematical foundations. Understanding these mathematical underpinnings is crucial for anyone serious about a career in AI, enabling you to not only use existing algorithms but also to develop new and innovative ones.
Linear Algebra
Linear algebra provides the framework for representing and manipulating data in high-dimensional spaces. This is fundamental because much of the data used in AI, such as images (represented as matrices of pixel values) and text (represented as vectors of word frequencies), is inherently high-dimensional. Key concepts include vectors, matrices, tensors, linear transformations, eigenvalues, and eigenvectors. These concepts are used extensively in machine learning algorithms like principal component analysis (PCA) for dimensionality reduction, and in deep learning architectures for processing data efficiently.
For example, a convolutional neural network (CNN) uses matrix multiplications extensively to process images, leveraging the power of linear algebra for feature extraction.
Calculus
Calculus, particularly differential and integral calculus, underpins many optimization algorithms crucial to AI. Machine learning models are trained by adjusting parameters to minimize a loss function, a process often achieved using gradient descent. Gradient descent relies on calculating the gradient of the loss function, which requires the application of partial derivatives (a concept from calculus). Moreover, understanding calculus is essential for comprehending backpropagation, the core algorithm for training deep neural networks.
In essence, calculus allows us to find the optimal settings for our models. For instance, in logistic regression, the model parameters are optimized by minimizing a cost function using gradient descent, a process that heavily relies on calculus.
Probability and Statistics
Probability and statistics are foundational to understanding uncertainty and making inferences from data. Many AI systems deal with noisy or incomplete data, and probability theory provides the tools to model and reason under uncertainty. Key concepts include probability distributions (e.g., Gaussian, Bernoulli), Bayes’ theorem, hypothesis testing, and statistical significance. These concepts are vital for tasks such as classification, regression, and anomaly detection.
For example, Naive Bayes classifiers use Bayes’ theorem to calculate the probability of a data point belonging to a particular class based on observed features. Similarly, statistical methods are essential for evaluating the performance of AI models and assessing their generalization ability. A/B testing, a common method for comparing different model versions, relies heavily on statistical significance testing.
Table Comparing Mathematical Importance Across AI Applications
Mathematical Area | Computer Vision | Natural Language Processing | Reinforcement Learning |
---|---|---|---|
Linear Algebra | High (image manipulation, feature extraction) | Medium (word embeddings, text representation) | Medium (state-action representation) |
Calculus | Medium (optimization of CNNs) | Medium (optimization of language models) | High (policy gradient methods) |
Probability & Statistics | Medium (object detection, image segmentation) | High (language modeling, sentiment analysis) | High (Markov Decision Processes, reward modeling) |
Programming for AI
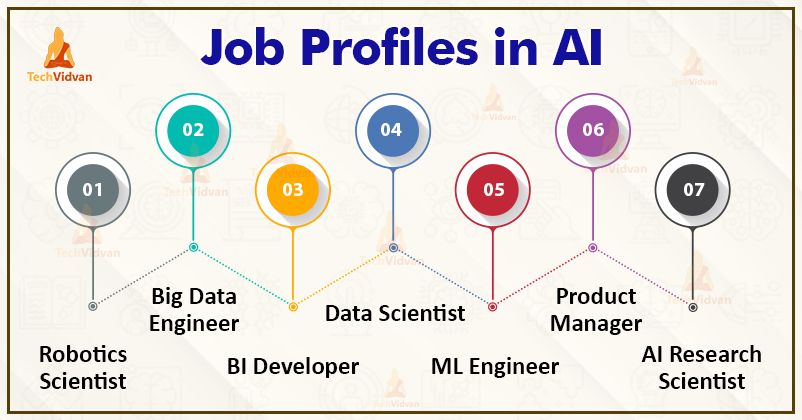
Building a career in artificial intelligence requires a strong foundation in programming. While several languages can be used, Python stands out as the dominant choice due to its extensive libraries specifically designed for AI and machine learning tasks. Understanding data structures and algorithms is crucial for writing efficient and scalable AI code. This section explores the essential programming languages, the importance of data structures and algorithms, and best practices for writing high-quality AI code.
Finally, a simple linear regression program will illustrate a core concept.
Essential Programming Languages for AI
Python’s popularity in the AI field stems from its readability, vast ecosystem of libraries, and active community support. Libraries like NumPy, Pandas, Scikit-learn, TensorFlow, and PyTorch provide pre-built functions and tools that significantly simplify the development process. Other languages like Java, C++, and R also have roles, particularly in specific applications or for performance-critical components, but Python remains the primary language for most AI projects.
The ease of prototyping and rapid development offered by Python makes it ideal for experimenting with different AI algorithms and approaches.
Data Structures and Algorithms in AI Programming
Efficient AI programming relies heavily on the appropriate selection and use of data structures and algorithms. Data structures like arrays, lists, dictionaries, and sets are fundamental for organizing and accessing data efficiently. Algorithms, such as search algorithms (e.g., breadth-first search, depth-first search), sorting algorithms (e.g., merge sort, quicksort), and graph algorithms (e.g., Dijkstra’s algorithm), are essential for processing large datasets and solving complex AI problems.
For example, a graph data structure is commonly used to represent relationships between data points in a network or social media analysis. Choosing the right algorithm can dramatically impact the performance of an AI system, especially when dealing with large datasets.
Best Practices for Writing Clean, Efficient, and Maintainable AI Code
Writing clean, efficient, and maintainable code is crucial for collaboration and long-term success in AI development. This involves adhering to coding style guidelines (like PEP 8 for Python), using meaningful variable names, adding comprehensive comments to explain complex logic, and breaking down code into modular functions. Version control systems (like Git) are essential for tracking changes and collaborating effectively.
Testing is also paramount; unit tests, integration tests, and other testing methodologies ensure the reliability and correctness of the code. Regular code reviews provide an additional layer of quality assurance and knowledge sharing among team members.
A Simple Linear Regression Program
This program demonstrates linear regression, a fundamental machine learning algorithm used to model the relationship between a dependent variable and one or more independent variables.“`pythonimport numpy as npimport matplotlib.pyplot as plt# Generate sample dataX = np.array([1, 2, 3, 4, 5])y = np.array([2, 3, 5, 4, 5])# Calculate the mean of X and yx_mean = np.mean(X)y_mean = np.mean(y)# Calculate the slope (m) and y-intercept (b)numerator = np.sum((X – x_mean)
(y – y_mean))
denominator = np.sum((X – x_mean)2)m = numerator / denominatorb = y_mean – m
x_mean
# Make predictionsy_predicted = m
X + b
# Plot the data and regression lineplt.scatter(X, y, color=’blue’, label=’Data Points’)plt.plot(X, y_predicted, color=’red’, label=’Regression Line’)plt.xlabel(‘X’)plt.ylabel(‘y’)plt.title(‘Linear Regression’)plt.legend()plt.show()# Print the equation of the lineprint(f”Equation of the line: y = m:.2fx + b:.2f”)“`This code first generates sample data, then calculates the slope and y-intercept of the best-fit line using the least squares method. Finally, it plots the data points and the regression line, providing a visual representation of the model.
The equation of the line is then printed, summarizing the relationship between X and y. This simple example showcases a core concept in AI programming and the power of Python’s libraries like NumPy and Matplotlib.
Machine Learning Fundamentals
Machine learning, a core component of AI, empowers computers to learn from data without explicit programming. This learning process allows systems to improve their performance on specific tasks over time, leading to increasingly accurate predictions and insightful analyses. Understanding the different types of machine learning is crucial for anyone aiming for a career in this field.
Supervised Learning
Supervised learning involves training a model on a labeled dataset, where each data point is paired with its corresponding output. The model learns to map inputs to outputs based on this labeled data, allowing it to predict the output for new, unseen inputs. This is akin to a teacher supervising a student’s learning process, providing feedback and correcting errors.
Common algorithms include linear regression, logistic regression, support vector machines (SVMs), and decision trees. For example, a supervised learning model could be trained on images of handwritten digits (inputs) and their corresponding numerical labels (outputs) to classify new handwritten digits.
Unsupervised Learning
In contrast to supervised learning, unsupervised learning deals with unlabeled data. The goal is to discover patterns, structures, or relationships within the data without any predefined output. This is like giving a child a box of toys and letting them explore and categorize them on their own. Common algorithms include clustering algorithms (k-means, hierarchical clustering), dimensionality reduction techniques (principal component analysis, t-SNE), and association rule mining (Apriori algorithm).
For example, customer segmentation based on purchasing behavior is a classic application of unsupervised learning. The algorithm identifies groups of customers with similar buying patterns without prior knowledge of customer segments.
Reinforcement Learning, Essential topics to study for a career in artificial intelligence
Reinforcement learning focuses on training an agent to interact with an environment and learn optimal actions to maximize a reward signal. The agent learns through trial and error, receiving rewards for desirable actions and penalties for undesirable ones. This learning paradigm is inspired by how animals learn through interactions with their environment. Examples include game playing (AlphaGo), robotics control, and resource management.
A self-driving car, for instance, uses reinforcement learning to learn optimal driving strategies by interacting with a simulated or real-world environment, receiving rewards for safe and efficient driving and penalties for accidents or traffic violations.
Comparison of Machine Learning Algorithms
Understanding the strengths and weaknesses of different algorithms is vital for selecting the most appropriate model for a given task.
Here’s a comparison of some common algorithms:
- Decision Trees:
- Strengths: Easy to understand and interpret, can handle both categorical and numerical data, requires little data preprocessing.
- Weaknesses: Prone to overfitting, can be unstable (small changes in data can lead to large changes in the tree).
- Support Vector Machines (SVMs):
- Strengths: Effective in high-dimensional spaces, relatively memory efficient.
- Weaknesses: Can be computationally expensive for large datasets, choice of kernel function is crucial.
- Neural Networks:
- Strengths: Can model complex non-linear relationships, highly accurate for many tasks, can handle large datasets.
- Weaknesses: Computationally expensive, requires large amounts of data, can be difficult to interpret (black box).
Deep Learning
Deep learning, a subfield of machine learning, utilizes artificial neural networks with multiple layers (hence “deep”) to extract higher-level features from raw input data. This allows for the modeling of complex patterns and relationships that shallower models struggle to capture, leading to significant advancements in fields like image recognition, natural language processing, and speech recognition. The power of deep learning lies in its ability to learn intricate representations automatically from data, reducing the need for extensive feature engineering.Deep learning models are characterized by their layered architecture, where each layer transforms the data received from the previous layer.
This hierarchical processing enables the network to learn increasingly abstract features. Different architectures are suited to different types of data and tasks.
Convolutional Neural Networks (CNNs)
CNNs are specifically designed for processing grid-like data, such as images and videos. Their architecture incorporates convolutional layers, which use filters to detect patterns in local regions of the input. These filters learn to identify features like edges, corners, and textures. Pooling layers then reduce the dimensionality of the feature maps, making the network more robust to variations in the input.
Fully connected layers at the end combine the extracted features to produce the final output, often a classification or regression result. For example, a CNN used for image classification might learn to identify edges in early layers, then shapes in intermediate layers, and finally objects in the later layers.
Recurrent Neural Networks (RNNs)
RNNs are designed for sequential data, such as text and time series. Unlike CNNs, RNNs have loops in their architecture, allowing them to maintain a hidden state that captures information from previous time steps. This allows them to model temporal dependencies in the data. A common type of RNN is the Long Short-Term Memory (LSTM) network, which is designed to address the vanishing gradient problem that can hinder the training of standard RNNs.
So you’re aiming for an AI career? That means mastering machine learning, deep learning, and data structures are key. But building AI applications often involves integrating with existing systems, which is where understanding development methodologies like those discussed in this insightful article on domino app dev the low code and pro code future becomes really helpful.
This knowledge helps bridge the gap between theoretical AI and practical implementation, making you a more well-rounded AI professional.
LSTMs are particularly effective in tasks requiring long-range dependencies, such as machine translation and speech recognition. For example, an LSTM might use information from earlier words in a sentence to accurately predict the next word.
Backpropagation
Backpropagation is the algorithm used to train deep learning models. It works by calculating the gradient of the loss function with respect to the model’s weights. The gradient indicates the direction of steepest descent in the loss landscape. By iteratively updating the weights in the direction opposite to the gradient, the model minimizes its loss and improves its performance.
The chain rule of calculus is crucial in backpropagation, allowing the gradient to be calculated efficiently through multiple layers of the network. This iterative process requires a significant amount of computational power, especially for large and deep networks.
Building and Training a Simple Deep Learning Model for Image Classification
Building a simple deep learning model for image classification involves several steps. First, a suitable dataset, like MNIST (handwritten digits), is chosen. The data is then preprocessed, which might involve resizing images and normalizing pixel values. A CNN architecture is defined, typically consisting of convolutional, pooling, and fully connected layers. A loss function, such as cross-entropy, and an optimizer, such as Adam, are selected.
The model is then trained using the backpropagation algorithm, iteratively updating its weights based on the calculated gradients. The training process is monitored using metrics like accuracy and loss, and the model’s performance is evaluated on a separate test set.
Hyperparameter Tuning
Hyperparameters are parameters that control the learning process, but are not learned during training. Examples include the learning rate, number of layers, and number of neurons per layer. Hyperparameter tuning is the process of finding the optimal values for these parameters to maximize model performance. Techniques such as grid search, random search, and Bayesian optimization can be used to explore the hyperparameter space efficiently.
For instance, experimenting with different learning rates can significantly impact the model’s convergence speed and final accuracy. A learning rate that is too high might lead to oscillations and prevent convergence, while a learning rate that is too low might result in slow training.
Natural Language Processing (NLP)
Natural Language Processing (NLP) bridges the gap between human language and computer understanding. It’s a fascinating field that allows machines to process, understand, and generate human language, opening up a world of possibilities in various applications. This involves tackling the complexities of syntax, semantics, and pragmatics to extract meaningful information from text and speech.NLP employs various techniques to analyze and manipulate text data.
These techniques are crucial for enabling computers to “understand” the nuances of human communication, going beyond simple matching to grasp context and meaning.
Fundamental NLP Concepts
Tokenization, stemming, and lemmatization are core processes in NLP that prepare text data for analysis. Tokenization is the process of breaking down text into individual words or units called tokens. For example, the sentence “The quick brown fox jumps over the lazy dog” would be tokenized into [“The”, “quick”, “brown”, “fox”, “jumps”, “over”, “the”, “lazy”, “dog”]. Stemming reduces words to their root form, often by removing suffixes.
For instance, “running,” “runs,” and “ran” would all be stemmed to “run.” Lemmatization, a more sophisticated approach, considers the context and reduces words to their dictionary form (lemma). Thus, “better” would become “good.” These processes help to reduce the dimensionality of the data and improve the accuracy of downstream NLP tasks.
NLP Tasks
NLP encompasses a wide range of tasks. Text classification categorizes text into predefined categories, such as spam detection (spam/not spam) or topic classification (sports, politics, etc.). Sentiment analysis determines the emotional tone of a text, identifying whether it expresses positive, negative, or neutral sentiment. This is vital for understanding customer feedback or social media trends. Machine translation automatically converts text from one language to another, breaking down language barriers and facilitating global communication.
These tasks often involve complex algorithms and models that leverage the power of machine learning and deep learning.
NLP Applications
NLP powers many applications we interact with daily. Chatbots use NLP to understand user queries and generate appropriate responses, providing automated customer service or assistance. Language assistants, such as Siri and Alexa, rely heavily on NLP to interpret voice commands and perform actions. Machine translation tools, like Google Translate, enable seamless communication across languages. In addition, NLP finds applications in areas such as medical diagnosis (analyzing patient records), legal research (summarizing legal documents), and literary analysis (identifying themes and stylistic patterns).
Building a Simple Sentiment Analysis Model
Let’s Artikel a simplified approach to building a sentiment analysis model.
- Data Collection and Preparation: Gather a dataset of text reviews with corresponding sentiment labels (positive, negative, neutral). Clean the data by removing irrelevant characters, handling missing values, and converting text to lowercase. A publicly available dataset like IMDB movie reviews would be suitable.
- Tokenization and Feature Extraction: Tokenize the text data. A simple approach would be to use bag-of-words, where each unique word becomes a feature. More advanced techniques like TF-IDF (Term Frequency-Inverse Document Frequency) can also be used to weigh words based on their importance.
- Model Selection and Training: Choose a machine learning model suitable for classification, such as Naive Bayes, Logistic Regression, or Support Vector Machines (SVM). Train the model on the prepared data, using the labeled sentiments as the target variable.
- Model Evaluation and Tuning: Evaluate the model’s performance using metrics like accuracy, precision, recall, and F1-score. Fine-tune the model by adjusting parameters or trying different algorithms to optimize its performance.
- Deployment and Prediction: Once satisfied with the model’s accuracy, deploy it to make predictions on new, unseen text data. This could involve integrating the model into a web application or other system.
This simplified guide provides a basic framework. Real-world sentiment analysis often involves more complex techniques, such as deep learning models (Recurrent Neural Networks or Transformers) and advanced feature engineering for higher accuracy.
Computer Vision
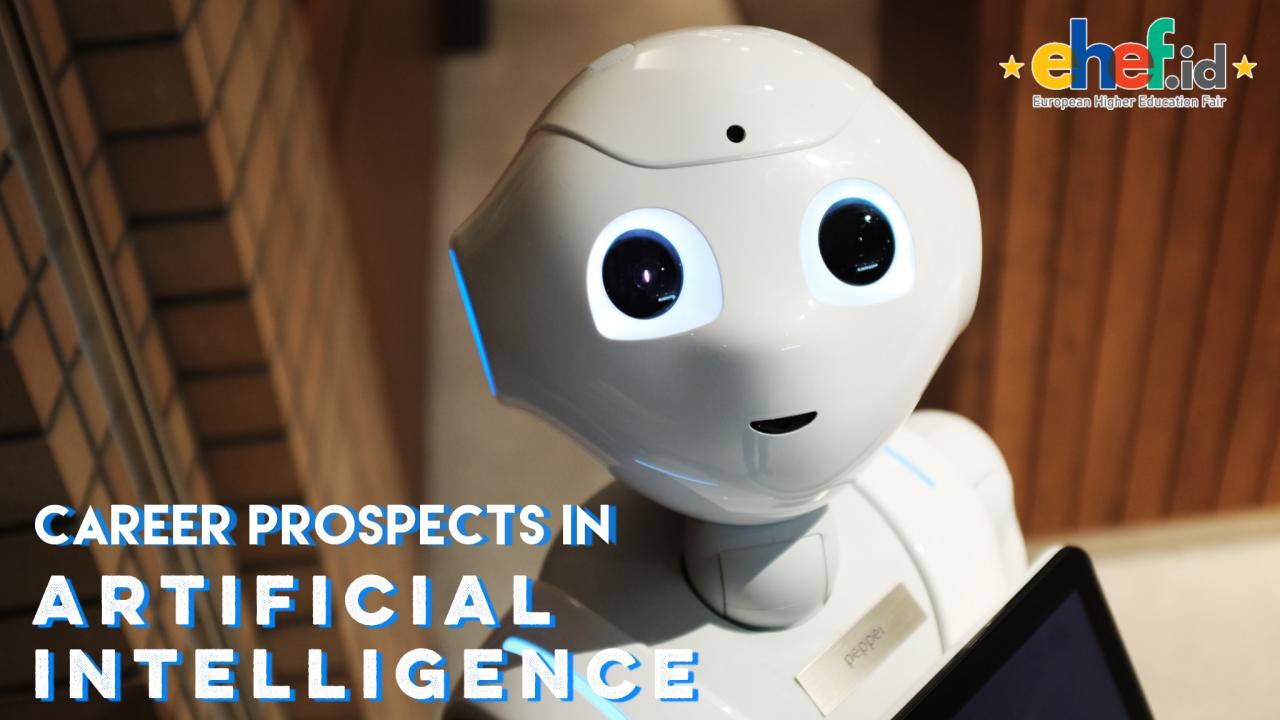
Computer vision, a field at the heart of AI, empowers computers to “see” and interpret images and videos in much the same way humans do. It bridges the gap between the digital world of pixels and the real-world understanding of objects, scenes, and events. This involves complex processes that mimic human visual perception, allowing machines to extract meaningful information from visual data.Image processing and computer vision are intertwined but distinct.
Image processing focuses on manipulating and enhancing images – think contrast adjustment, noise reduction, or sharpening. Computer vision goes further, using processed images to understand their content, identify objects, and even predict future events based on visual input. Feature extraction is key; this involves identifying specific characteristics of an image, like edges, corners, or textures, that can be used to distinguish objects or regions.
Object detection builds upon this by pinpointing the location and classification of objects within an image.
Core Concepts of Image Processing and Computer Vision
Image processing techniques form the foundation of computer vision systems. Basic operations include filtering (smoothing, sharpening), transformations (resizing, rotation), and color space conversions (RGB to HSV, for example). More advanced techniques involve techniques like edge detection (using algorithms like the Sobel operator to identify boundaries between objects), feature extraction (using techniques like SIFT or SURF to create robust representations of image features), and image segmentation (partitioning an image into meaningful regions).
These processes are crucial for preparing raw image data for higher-level computer vision tasks. Object detection, a crucial component, involves identifying and localizing objects within an image using algorithms like YOLO (You Only Look Once) or Faster R-CNN (Region-based Convolutional Neural Networks). These algorithms typically combine feature extraction with classification to pinpoint objects and label them.
Computer Vision Tasks
Computer vision tackles a diverse range of tasks. Image classification involves assigning a label (e.g., “cat,” “dog,” “car”) to an entire image. Object recognition extends this by identifying multiple objects within a single image and determining their locations. Image segmentation goes even further, dividing the image into multiple regions corresponding to different objects or areas of interest. Other tasks include pose estimation (determining the orientation of an object), image retrieval (finding similar images in a database), and video analysis (tracking objects over time).
Applications of Computer Vision
Computer vision is revolutionizing numerous industries. In autonomous driving, it’s essential for obstacle detection, lane keeping, and navigation. Cameras and sophisticated algorithms allow self-driving cars to perceive their surroundings and make real-time decisions. In medical imaging, computer vision aids in disease diagnosis, assisting radiologists in analyzing X-rays, CT scans, and MRIs to detect anomalies like tumors or fractures with higher accuracy and speed.
Other applications include facial recognition for security systems, robotic vision for industrial automation, and augmented reality applications that overlay digital information onto the real world.
Hypothetical Computer Vision System for Retail Inventory Management
Imagine a computer vision system designed for automated retail inventory management. This system would consist of several components: high-resolution cameras strategically placed throughout a store, a powerful processing unit capable of handling real-time image analysis, and a software system integrating the image analysis with the store’s inventory database. The system would capture images of shelves, using image processing techniques to enhance image quality and reduce noise.
Object detection algorithms would then identify and locate individual products on the shelves. By comparing the detected products with the inventory database, the system could automatically track stock levels, identifying low-stock items or discrepancies between physical inventory and recorded data. This system could significantly improve efficiency, reduce manual labor, and minimize stockouts. The system’s accuracy would be regularly evaluated and refined using machine learning techniques, improving its performance over time.
This system could potentially use deep learning models trained on a large dataset of product images to ensure high accuracy in object detection and classification.
Data Science and AI Ethics
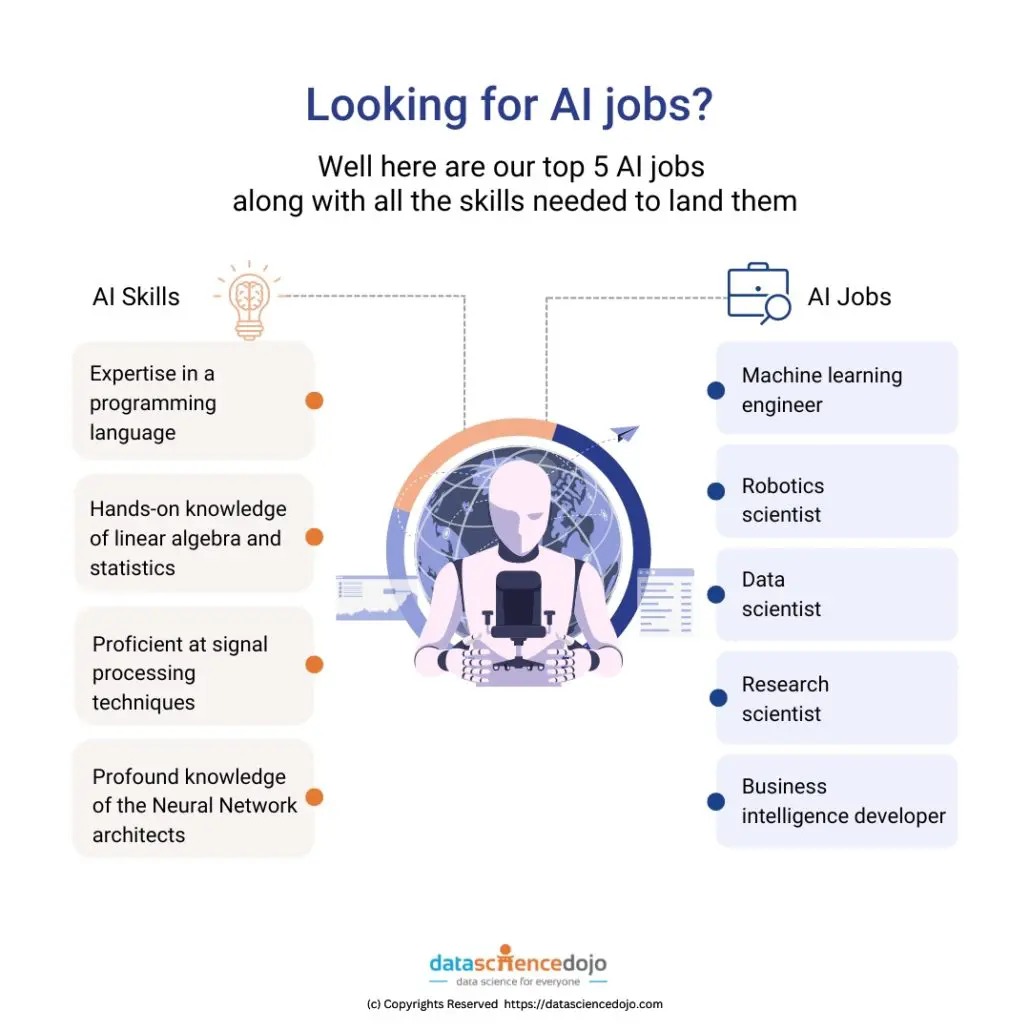
Building robust and reliable AI systems requires more than just sophisticated algorithms; it demands a deep understanding of data science principles and a strong ethical compass. Data forms the bedrock of AI, and its quality directly impacts the performance and trustworthiness of the resulting system. Ethical considerations, meanwhile, are paramount to ensuring AI benefits society as a whole and avoids perpetuating or exacerbating existing inequalities.Data preprocessing and feature engineering are critical steps in the AI development pipeline.
These techniques transform raw data into a format suitable for machine learning algorithms, significantly influencing the accuracy and efficiency of the model. Without proper data cleaning, transformation, and feature selection, even the most advanced algorithms can produce unreliable or biased results.
Data Preprocessing and Feature Engineering in AI
Data preprocessing involves cleaning, transforming, and preparing raw data for analysis. This includes handling missing values, dealing with outliers, and converting data into appropriate formats. Feature engineering, on the other hand, focuses on creating new features from existing ones to improve model performance. For example, extracting relevant information from text data (NLP) or creating composite features from numerical data can significantly enhance model accuracy.
Consider a dataset predicting house prices: raw data might include square footage and number of bedrooms. Feature engineering could create a new feature representing “price per square foot,” providing a more insightful predictor. Effective preprocessing and feature engineering significantly reduce noise, improve model interpretability, and enhance overall prediction accuracy.
Ethical Considerations in AI Development and Deployment
The development and deployment of AI systems raise several ethical concerns. Bias in data can lead to discriminatory outcomes. For example, a facial recognition system trained primarily on images of light-skinned individuals might perform poorly on individuals with darker skin tones. Fairness necessitates designing AI systems that treat all individuals equitably, regardless of their background or characteristics. Accountability is crucial to determine responsibility when AI systems make errors or cause harm.
Clear lines of responsibility must be established to address potential issues and ensure transparency. These considerations require careful attention throughout the AI lifecycle, from data collection to model deployment and ongoing monitoring.
Potential Risks and Challenges Associated with AI Systems
AI systems, while powerful, present several risks and challenges. Job displacement due to automation is a major concern, requiring proactive measures for retraining and workforce adaptation. The potential for misuse of AI in malicious activities, such as deepfakes or autonomous weapons, poses significant societal risks. Algorithmic bias can perpetuate and amplify existing societal inequalities, leading to unfair or discriminatory outcomes.
The lack of transparency in some AI systems (“black box” models) makes it difficult to understand their decision-making processes, hindering accountability and trust. Finally, the rapid pace of AI development necessitates robust regulatory frameworks to mitigate potential harms and ensure responsible innovation.
Strategies for Mitigating Bias and Ensuring Responsible AI Development
Mitigating bias requires careful attention to data collection, preprocessing, and model development. Using diverse and representative datasets is crucial. Techniques like data augmentation can help balance datasets and reduce bias. Algorithmic fairness metrics can be used to assess and improve model fairness. Explainable AI (XAI) techniques aim to increase transparency and interpretability, making it easier to identify and address biases.
Establishing ethical guidelines and regulations, fostering interdisciplinary collaboration between AI experts and ethicists, and promoting public education and engagement are all vital steps towards responsible AI development. Regular audits and monitoring of AI systems are also essential to detect and address potential biases and unintended consequences.
Last Point
So, there you have it – a roadmap to navigating the exciting world of artificial intelligence. Building a career in AI requires a multifaceted skillset, blending mathematical prowess, programming expertise, and a deep understanding of various AI subfields. Remember, continuous learning is key in this rapidly evolving field. Embrace the challenges, stay curious, and watch your AI journey unfold.
The future is intelligent, and you’re about to be a part of it.
Questions Often Asked: Essential Topics To Study For A Career In Artificial Intelligence
What’s the best programming language to learn for AI?
Python is the dominant language in AI due to its extensive libraries (like TensorFlow and PyTorch) and ease of use.
How much math do I
-really* need for AI?
A solid foundation in linear algebra, calculus, probability, and statistics is essential. The level of depth needed depends on your specific AI specialization.
Is a PhD necessary for a career in AI?
No, while a PhD can open doors to research roles, many successful AI careers are built with Master’s degrees or even strong undergraduate backgrounds coupled with practical experience.
What are some entry-level AI jobs?
Consider roles like Data Scientist, Machine Learning Engineer, AI/ML Intern, or even a Junior Software Engineer focusing on AI projects.